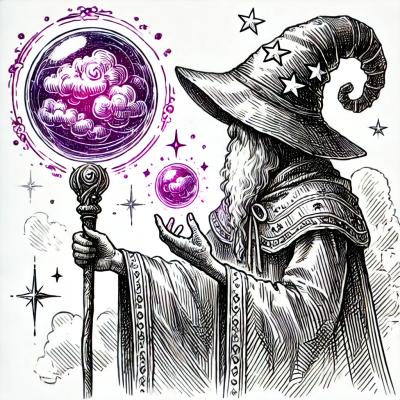
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
jsonschema
Advanced tools
The jsonschema npm package is a JSON Schema validator that can validate JavaScript objects using JSON Schemas. It is commonly used to ensure that data conforms to a predefined schema, and it can be used to validate data at runtime, as part of a testing suite, or during development to ensure data integrity.
Validation
This feature allows you to validate a JavaScript object against a JSON Schema. The code sample creates a new Validator instance, defines a schema with required properties, and validates a sample data object against this schema. It outputs an empty array if the data is valid or a list of errors if it is invalid.
{"const Validator = require('jsonschema').Validator; const v = new Validator(); const schema = { 'type': 'object', 'properties': { 'name': { 'type': 'string' }, 'age': { 'type': 'integer', 'minimum': 0 } }, 'required': ['name', 'age'] }; const sampleData = { 'name': 'John Doe', 'age': 25 }; const validation = v.validate(sampleData, schema); console.log(validation.errors); // [] if valid, or list of errors if invalid."}
Custom Formats
This feature allows you to define custom formats for validation. The code sample shows how to add a custom format to the Validator instance and then use it in a schema to validate data.
{"const Validator = require('jsonschema').Validator; const v = new Validator(); v.customFormats.myFormat = function(input) { return input === 'specialValue'; }; const schema = { 'type': 'string', 'format': 'myFormat' }; const sampleData = 'specialValue'; const validation = v.validate(sampleData, schema); console.log(validation.valid); // true if valid, or false if invalid."}
Asynchronous Validation
This feature allows for asynchronous validation, which can be useful when dealing with complex validations that may require I/O operations. The code sample demonstrates how to perform validation asynchronously using a callback function.
{"const Validator = require('jsonschema').Validator; const v = new Validator(); const schema = { 'type': 'object', 'properties': { 'name': { 'type': 'string' }, 'age': { 'type': 'integer', 'minimum': 0 } }, 'required': ['name', 'age'] }; const sampleData = { 'name': 'John Doe', 'age': 25 }; v.validate(sampleData, schema, function(errors, valid) { console.log(valid); // true if valid, or false if invalid });"}
Ajv is another popular JSON Schema validator that is known for its performance and being compliant with the JSON Schema specification. It supports all JSON Schema versions and includes features like compiling schemas for faster validation, adding custom keywords, and more. Compared to jsonschema, Ajv may offer better performance and more features.
Tiny Validator (tv4) is a lightweight JSON Schema validator. It supports draft v4 of the JSON Schema specification and is designed to be simple and easy to use. While it may not have as many features as jsonschema, it is a good choice for projects that require a minimalistic approach.
Joi is a powerful schema description language and data validator for JavaScript. Unlike jsonschema, which uses JSON Schema format, Joi allows you to create validation schemas using a fluent API. It is often used for validating data in REST APIs and can be more intuitive to use for those who prefer a code-based schema definition over JSON.
Simple and fast JSON schema validator.
var v = new Validator();
var instance = 4;
var schema = {"type": "number"};
console.log(v.validate(instance, schema));
Any non ticked off definition types are ignored.
Value | JSON Schema Draft | jsonschema | Comments |
---|---|---|---|
type | ✔ | ✔ | |
properties | ✔ | ✔ | |
patternProperties | ✔ | ✔ | |
additionalProperties | ✔ | ✔ | |
items | ✔ | ✔ | |
additionalItems | ✔ | ✔ | |
required | ✔ | ✔ | |
dependencies | ✔ | ✔ | |
minimum | ✔ | ✔ | |
maximum | ✔ | ✔ | |
exclusiveMinimum | ✔ | ✔ | |
exclusiveMaximum | ✔ | ✔ | |
minItems | ✔ | ✔ | |
maxItems | ✔ | ✔ | |
uniqueItems | ✔ | ✔ | |
pattern | ✔ | ✔ | |
minLength | ✔ | ✔ | |
maxLength | ✔ | ✔ | |
enum | ✔ | ✔ | |
default | ✔ | ✔ | informational only |
title | ✔ | ✔ | informational only |
description | ✔ | ✔ | informational only |
format | ✔ | ✔ | |
divisibleBy | ✔ | ✔ | |
disallow | ✔ | ✔ | |
extends | ✔ | ✔ | |
id | ✔ | ✔ | informational only |
$ref | ✔ | ✔ | |
$schema | ✔ | ignored |
Value | JSON Schema Draft | jsonschema | Comments |
---|---|---|---|
string | ✔ | ✔ | |
number | ✔ | ✔ | |
integer | ✔ | ✔ | |
boolean | ✔ | ✔ | |
object | ✔ | ✔ | |
array | ✔ | ✔ | |
null | ✔ | ✔ | |
date | ✔ | ||
any | ✔ | ✔ | |
Union Types | ✔ | ✔ |
Value | JSON Schema Draft | jsonschema | Comments |
---|---|---|---|
date-time | ✔ | ✔ | |
date | ✔ | ✔ | |
time | ✔ | ✔ | |
utc-millisec | ✔ | ✔ | Any number (integer or float) is allowed |
regex | ✔ | ✔ | We test for valid regular expression |
color | ✔ | ✔ | |
style | ✔ | ✔ | |
phone | ✔ | ✔ | Should follow http://en.wikipedia.org/wiki/E.123 standard. |
uri | ✔ | ✔ | |
email | ✔ | ✔ | |
ip-address | ✔ | ✔ | |
ipv6 | ✔ | ✔ | |
host-name | ✔ | ✔ | |
alpha | ✔ | ||
alphanumeric | ✔ |
Uses [https://github.com/Julian/JSON-Schema-Test-Suite](JSON Schema Test Suite) as well as our own. You'll need to update and init the git submodules:
git submodule update --init
npm test
jsonschema is licensed under MIT license.
Copyright (C) 2012 Tom de Grunt <tom@degrunt.nl>
Permission is hereby granted, free of charge, to any person obtaining a copy of
this software and associated documentation files (the "Software"), to deal in
the Software without restriction, including without limitation the rights to
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
of the Software, and to permit persons to whom the Software is furnished to do
so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
FAQs
A fast and easy to use JSON Schema validator
The npm package jsonschema receives a total of 2,643,718 weekly downloads. As such, jsonschema popularity was classified as popular.
We found that jsonschema demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.