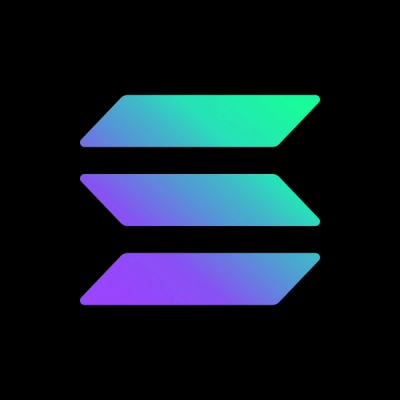
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
jsonVerse is a lightweight JSON-based database package for Node.js. It provides a simple interface to store, retrieve, and manage data using JSON files.
jsonVerse is a lightweight JSON-based database package for Node.js. It provides a simple interface to store, retrieve, and manage data using JSON files.
The jsonVerse package is a powerful utility designed to simplify the management of JSON data files within a designated folder. It offers methods for adding, editing, deleting, and retrieving data from JSON files. This wiki provides detailed examples and usage scenarios to help you effectively implement the jsonVerse package in your projects.
To begin using the jsonVerse package, you'll need to install it via npm. Open your terminal and run the following command:
npm install jsonverse
To get started, import the required modules, set up an Express router, and initialize the jsonVerse instance:
const express = require("express");
const app = express();
const jsonverse = require("jsonverse");
// Initialize the JSONDatabase instance
const db = new jsonverse({
dataFolderPath: "./MyData", // data directory
logFolderPath: "./MyLogs", // logs directory
activateLogs: true, // to enable the logs set this value to true
});
You can display all the data from your website using the following code:
app.get("/", async (req, res) => {
try {
const allData = await db.allData();
// ... (rendering logic)
} catch (err) {
// ... (error handling)
}
});
you can display data from a specific data file by file name
app.get("/", async (req, res) => {
try {
db.readData(dataName) // dataName: the name of the json file like test.json the data name will be "test"
.then((result) => {
res.send(result); // result is the content of the json file
})
.catch((err) => {
console.log(err);
});
} catch (err) {
// ... (error handling)
}
});
To add data, use the following code:
app.post("/add", async (req, res) => {
try {
const { dataName, name, social, rank, competition, date, edu, password } =
req.body;
const newData = {
social,
name,
rank,
competition,
date,
edu,
password: db.encrypt(password, "Your-Secret-Key"),
};
await db.saveData(dataName, newData);
// ... (redirect or response)
} catch (err) {
// ... (error handling)
}
});
Retrieve data by its ID with this code:
app.get("/:id", async (req, res) => {
const id = req.params.id;
try {
const result = await db.findById(id);
if (result) {
// ... (rendering logic)
// remember if the retrieved data contained encrypted data to use
// db.decrypt(encryptedData, secretKey)
} else {
// ... (not found logic)
}
} catch (err) {
// ... (error handling)
}
});
Delete data by its ID using this code:
app.delete("/:id", async (req, res) => {
const id = req.params.id;
try {
await db.delById(id);
// ... (response or redirect)
} catch (err) {
// ... (error handling)
}
});
Edit existing data using this code:
app.post("/edit/:id", async (req, res) => {
const id = req.params.id;
const { name, social, rank, competition, date, edu } = req.body;
try {
const updatedData = {
social,
name,
rank,
competition,
date,
edu,
};
await db.editById(id, updatedData);
// ... (response or redirect)
} catch (err) {
// ... (error handling)
}
});
you can encrypt/decrypt the data that you are saving by this commend
db.encrypt(data, secretKey);
db.decrypt(encryptedData, secretKey);
backup the data files you want by the dataName in Backup folder in Data Folder (the package create it automatically)
db.backupCreate(dataName); // dataName: the name of the data file you want to backup
Restore the backup data files you want by the dataName in Backup ./Data/Backup
./Data is the path you add to the data folder
db.backupRestore(dataName, backupFileName); // the dataName is the data you want to restore to it & the backupFileName is the backup file name you got after backing up
Delete the backup data json files you want by the dataName in Backup folder in Data Folder (the package create it automatically)
const retentionDays = 5; // write here with days the time you want to delete the backups since then like i want to delete the backup the had been saved the last 5 days
db.backupDelete(dataName, retentionDays);
you can import data from json files from outside the project files
db.importData(dataName, (format = "json"), filePath);
// dataName: the data file you want to import to if exist if doesn't write the new data name instead
// format: to set the format to json you don't need to write it it's json by default
// filePath: where is the file you want to import
you can Export data from json files
db.exportData(dataName, (format = "json"));
// dataName: the data file you want to Export
// format: to set the format of the new exported file like json and csv
The jsonVerse package simplifies the management of JSON data files within a specified folder. With the provided examples and usage instructions, you'll be able to efficiently integrate the jsonVerse package into your projects to streamline data operations.
FAQs
jsonVerse is a lightweight JSON-based database package for Node.js. It provides a simple interface to store, retrieve, and manage data using JSON files.
The npm package jsonverse receives a total of 1 weekly downloads. As such, jsonverse popularity was classified as not popular.
We found that jsonverse demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.