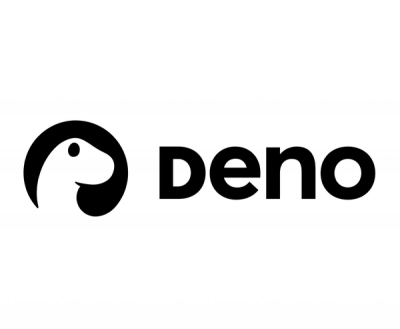
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
jss-preset-default
Advanced tools
The jss-preset-default package provides a set of default plugins for JSS (JavaScript Style Sheets). It includes a collection of plugins that help with various aspects of styling in JavaScript, such as vendor prefixing, nested selectors, and more.
Vendor Prefixing
Automatically adds vendor prefixes to CSS properties to ensure compatibility across different browsers.
const jss = create(preset());
const styles = {
button: {
display: 'flex',
justifyContent: 'center'
}
};
const { classes } = jss.createStyleSheet(styles).attach();
Nested Selectors
Allows the use of nested selectors within your styles, similar to how you would use them in Sass or Less.
const jss = create(preset());
const styles = {
container: {
'&:hover': {
backgroundColor: 'blue'
}
}
};
const { classes } = jss.createStyleSheet(styles).attach();
Global Styles
Enables the definition of global styles that apply to the entire document.
const jss = create(preset());
const styles = {
'@global': {
body: {
margin: 0,
padding: 0
}
}
};
const { classes } = jss.createStyleSheet(styles).attach();
styled-components is a library for React and React Native that allows you to use component-level styles in your application. It uses tagged template literals to style your components. Unlike jss-preset-default, styled-components is more tightly integrated with React and offers a more component-centric approach to styling.
Emotion is a performant and flexible CSS-in-JS library. It allows you to style applications quickly with string or object styles. Emotion offers a similar set of features to jss-preset-default but is known for its performance and flexibility, making it a popular choice for large-scale applications.
Aphrodite is a framework-agnostic CSS-in-JS library that focuses on performance and ease of use. It provides features like media queries and pseudo-selectors. Compared to jss-preset-default, Aphrodite is simpler and more lightweight, making it suitable for smaller projects or those that do not require extensive styling capabilities.
Preset allows to setup JSS quickly with default settings and a number of plugins so that you don't need to learn how to setup plugins.
preset()
Preset exports a default function which accepts options. Options is a map of plugin name in camel case and plugin options as value.
It returns a JSS options object, which can be passed to JSS constructor or the setup
.
preset({somePlugin: options})
import jss from 'jss'
import preset from 'jss-preset-default'
jss.setup(preset())
import {create} from 'jss'
import preset from 'jss-preset-default'
const jss = create(preset())
File a bug against cssinjs/jss prefixed with [jss-preset-default].
MIT
FAQs
Default preset for JSS with selected plugins.
The npm package jss-preset-default receives a total of 258,346 weekly downloads. As such, jss-preset-default popularity was classified as popular.
We found that jss-preset-default demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.