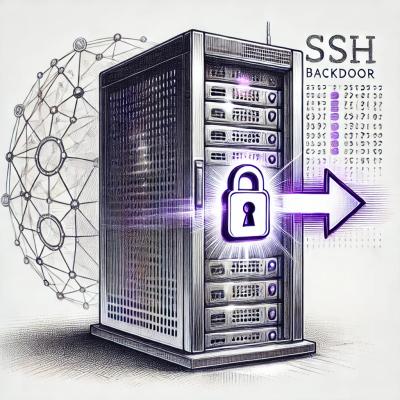
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
jsx-dom-runtime
Advanced tools
A tiny in 500 bytes library to JSX syntax templates for DOM.
npm i jsx-dom-runtime
# or
yarn add jsx-dom-runtime
Add preset to your .babelrc
file.
.babelrc
{
"presets": [
"jsx-dom-runtime/babel-preset"
]
}
// add to the end of the head
document.head.append(
<link rel="stylesheet" href="/style.css" />
);
// add to the end the the body
document.body.append(
<main class="box">
<h1 class="title">Hello World!</h1>
</main>
);
Support general JSX syntax.
Function components must start with a capital letter or they won’t work.
const App = ({ name }) => (
<div>Hello {name}</div>
);
document.body.append(<App name="Bob" />);
Use <>...</>
syntax, to group multiple elements together. Under the hood, it use DocumentFragment
interface.
document.body.append(
<>
<p>Hello</p>
<p>World</p>
</>
);
Adding a reference to a DOM Element
import { useRef } from 'jsx-dom-runtime';
const ref = useRef();
const addItem = () => {
// add to the start of the list
ref.current.prepend(<li>New Item</li>);
};
document.body.append(
<>
<button type="button" onclick={addItem}>
Add Item
</button>
<ul ref={ref} />
</>
);
const callbackRef = (node) => {
node.addEventListener('focusin', () => {
node.style.backgroundColor = 'pink';
});
node.addEventListener('focusout', () => {
node.style.backgroundColor = '';
});
};
document.body.append(
<input type="text" ref={callbackRef} />
);
Use the Text node in a DOM tree.
import { useText } from 'jsx-dom-runtime';
const [text, setText] = useText('The initial text');
document.body.append(
<>
<p>{text}</p>
<button type="button" onclick={() => {
setText('Clicked!');
}}>
Click me
</button>
</>
);
Get template from a string.
import { Template } from 'jsx-dom-runtime';
document.body.append(
<Template>
{`<svg width="24" height="24" aria-hidden="true">
<path d="M12 12V6h-1v6H5v1h6v6h1v-6h6v-1z"/>
</svg>`}
</Template>
);
Add custom attributes in JSX.Element
.
import { extensions } from 'jsx-dom-runtime';
extensions
.set('x-class', (node, value) => {
node.setAttribute('class', value.filter(Boolean).join(' '));
})
.set('x-dataset', (node, value) => {
for (let key in value) {
if (value[key] != null) {
node.dataset[key] = value[key];
}
}
})
.set('x-autofocus', (node, value) => {
setTimeout(() => node.focus(), value);
});
document.body.append(
<input
x-class={['one', 'two']}
x-dataset={{ testid: 'test', hook: 'text' }}
x-autofocus={1000}
/>
);
global.d.ts
declare global {
namespace JSX {
interface Attributes {
'x-class'?: string[];
'x-dataset'?: Record<string, string>;
'x-autofocus'?: number;
}
}
}
export {};
Result
<input class="one two" data-testid="test" data-hook="text">
Add support of the DOM Element object properties. By default supported property value
.
import { properties } from 'jsx-dom-runtime';
properties.add('textContent') // https://developer.mozilla.org/en-US/docs/Web/API/Node/textContent
.add('innerHTML') // https://developer.mozilla.org/en-US/docs/Web/API/Element/innerHTML
.add('volume') // https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/volume
.add('muted'); // https://developer.mozilla.org/en-US/docs/Web/API/HTMLMediaElement/muted
document.body.append(
<>
<span textContent="Hello, world!" />
<span innerHTML="<p>Hello, world!</p>" />
<audio
src="https://interactive-examples.mdn.mozilla.net/media/cc0-audio/t-rex-roar.mp3"
controls
volume={0.9}
muted
/>
</>
);
global.d.ts
declare global {
namespace JSX {
interface Attributes {
textContent?: string;
innerHTML?: string;
muted?: boolean;
volume?: number;
}
}
}
export {};
TypeScript is able used only for type checking. The library doesn't support compilation with TypeScript. Use @babel/preset-typescript
.babelrc
{
"presets": [
"@babel/typescript",
"jsx-dom-runtime/babel-preset"
]
}
To provide type checking add tsconfig.json
file to your project:
tsconfig.json
{
"compilerOptions": {
"jsx": "react-jsx",
"jsxImportSource": "jsx-dom-runtime",
"moduleResolution": "node",
"noEmit": true,
"lib": [
"DOM"
]
}
}
Example:
src/index.tsx
import type { FC } from 'jsx-dom-runtime';
interface Props {
text: string;
}
const App: FC<Props> = ({ text }) => (
<div class="card">
<div class="text">{text}</div>
</div>
);
document.body.append(<App text="Hello!" />);
FAQs
A tiny in 500 bytes library to JSX syntax templates for DOM. Support HTML, SVG and MathML tags
The npm package jsx-dom-runtime receives a total of 107 weekly downloads. As such, jsx-dom-runtime popularity was classified as not popular.
We found that jsx-dom-runtime demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.