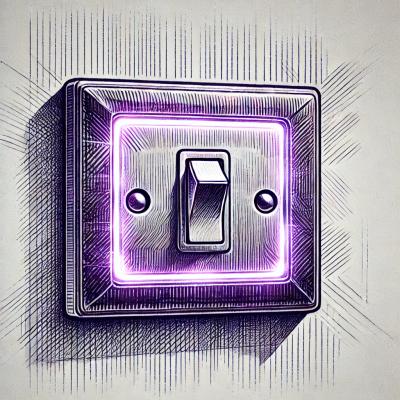
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
[](https://github.com/komarovalexander/ka-table/blob/master/LICENSE) [](https://
The customizable, extendable, lightweight (~50kb for js or ts scripts) and free React Table Component
Can easily be included in react projects, never mind it is ts or js
Command Column - Functional columns which are not bound to data and used to add custom command to table
Custom Cell - Best way to customise look of every column in table
Custom Editor - Table supports user created editors
Custom Header Cell - Customisation of header cell
Editing - Editing out of the box
Events - Most events are trackable
Filter Extended - Easy filtered by extended filters
Filter Row - Built-in filter row
Filter Row - Custom Editor - Customise filter cell every way you want
Grouping - Group data for most convenient work with it
25000 Rows - Virtualisation are supported
10000 Grouped Rows - Virtualisation work well with grouping
Search - Search by the whole Table is easy
Selection - Select and process specific rows
npm
npm install ka-table
yarn
yarn add ka-table
import React, { useState } from 'react';
import { ITableOption, Table } from 'ka-table';
import { DataType, SortDirection, SortingMode } from 'ka-table/enums';
import { OptionChangeFunc } from 'ka-table/types';
const dataArray: any[] = [
{ id: 1, name: 'Mike Wazowski', score: 80, passed: true },
{ id: 2, name: 'Billi Bob', score: 55, passed: false },
{ id: 3, name: 'Tom Williams', score: 45, passed: false },
{ id: 4, name: 'Kurt Cobain', score: 75, passed: true },
{ id: 5, name: 'Marshall Bruce', score: 77, passed: true },
{ id: 6, name: 'Sunny Fox', score: 33, passed: false },
];
const tableOption: ITableOption = {
columns: [
{
dataType: DataType.String,
key: 'name',
sortDirection: SortDirection.Descend,
style: { width: '33%' },
title: 'Name',
},
{ key: 'score', title: 'Score', style: { width: '10%' }, dataType: DataType.Number },
{ key: 'passed', title: 'Passed', dataType: DataType.Boolean },
],
rowKeyField: 'id',
sortingMode: SortingMode.Single,
};
const SortingDemo: React.FC = () => {
const [option, changeOptions] = useState(tableOption);
const onOptionChange: OptionChangeFunc = (value) => {
changeOptions({...option, ...value });
};
return (
<Table
{...option}
data={dataArray}
onOptionChange={onOptionChange}
/>
);
};
export default SortingDemo;
Properties
Name | Type | Description |
---|---|---|
columns | Column[] | Columns in table and their look and behaviour |
data | any[] | The data which is shown in Table's rows |
editableCells | Cell[] | This property contains the array of cells which are being edited |
editingMode | EditingMode | Sets the table's editing mode |
filterRow | FilterCondition[] | Sets filters for columns |
groups | Group[] | Group's in the table |
groupsExpanded | any[][] | Contains groups which are expanded in the grid |
rowKeyField | string | Property of data's item which is used to identitify row |
search | string | Specifies the text which should be found in the data |
selectedRows | any[] | Specifies the array of rows keys which are should be marked as selected |
sortingMode | SortingMode | Sorting mode |
virtualScrolling | VirtualScrolling | Virtual scrolling options - set it as empty object {} to enable virtual scrolling and auto calculate its parameters |
Describes column of table its look and behaviour Properties
Name | Type | Description |
---|---|---|
cell | CellFunc | Returns a custom cell if it is not in editable mode |
dataType | DataType | Specifies the type of column |
editor | EditorFunc | Returns an editor if cell is in editable mode |
filterCell | EditorFunc | Returns an editor for filter row cell |
field | string | Specifies the property of data's object which value will be used in column, if null value from key option will be used |
format | FormatFunc | Returns formated cell string |
headCell | HeaderCellFunc | Returns a custom header cell |
isEditable | boolean | Specifies can column be editable or not |
key | string | Mandatory field, specifies unique key for the column |
search | SearchFunc | Overrides the default search method for the cell. Executes if Table.search option is set |
sortDirection | SortDirection | Sets the direction of sorting for the column |
style | React.CSSProperties | Sets the style options of the elements |
title | string | Specifies the text of the header |
validation | ValidationFunc | Returns the validation error string or does not return anything in case of passed validation |
Describes the position of a cell in the table
Properties
Name | Type | Description |
---|---|---|
field | string | The field of |
specific column | ||
rowKeyValue | any | Data's key value of ะตัั specific row |
Properties
Name | Type | Description |
---|---|---|
field | string | The filtered column's field |
operator | string | Operator which will be applied for filtering |
value | any | Filtered value |
Properties
Name | Type | Description |
---|---|---|
field | string | The grouped column's field |
Properties
Name | Type | Description |
---|---|---|
scrollPosition | number | Current scroll top position |
itemHeight | ((data: any) => number) | number | Returns height of specific row |
tbodyHeight | number | tbody height |
Property | String value |
---|---|
Boolean | 'boolean' |
Date | 'date' |
Number | 'number' |
Object | 'object' |
String | 'string' |
Property | String value | Description |
---|---|---|
None | 'none' | Editing is disabled |
Cell | 'cell' | Data is edited by cell to cell, click by cell activates editing |
Property | String value |
---|---|
Ascend | 'ascend' |
Descend | 'descend' |
Property | String value |
---|---|
None | 'none' |
Single | 'single' |
(props: ICellContentProps
) => any;
Function which obtains ICellContentProps
as parameter and returns React component which should be shown instead of cell content.
(props: ICellEditorProps
) => any;
Function which obtains ICellEditorProps
as parameter and returns React component which should be shown instead of default editor.
(value: any) => any;
Function which obtains value as parameter and returns formated value which will be shown in cell.
(searchText?: string, rowData?: any, column?: Column) => boolean;
Function which obtains searchText?: string, rowData?: any, column?: Column - as parameters and returns boolean value which is true if cell's value is matched with searched value and false otherwise.
(value: any, rowData: any) => string | void;
Function which obtains value of specific cell and row - as parameters and returns validation error string or does not return anything in case of passed validation.
Properties
Name | Type | Description |
---|---|---|
column | Column | settings of the column in which editor is shown |
rowData | any | data of the row in which editor is shown |
close | () => void | call this method to close editor |
onValueChange | (newValue: any) => void | call this method to change value of the row: onValueChange({ ...rowData, ...{ [field]: value } }) |
Properties
Name | Type | Description |
---|---|---|
column | Column | settings of the column in which editor is shown |
openEditor | () => void | call this method to open editor of the cell |
rowData | any | data of the row in which editor is shown |
FAQs
The customizable, extendable, lightweight, and fully free React Table Component
The npm package ka-table receives a total of 5,733 weekly downloads. As such, ka-table popularity was classified as popular.
We found that ka-table demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.