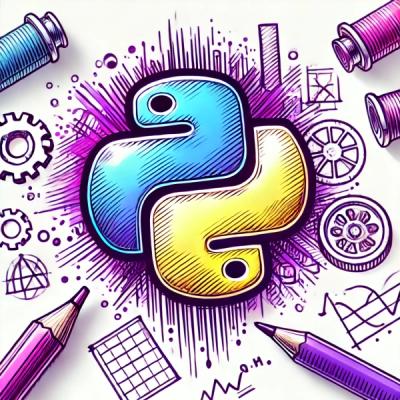
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
kapix-graphql-prisma-client
Advanced tools
This is a client-side library made for interacting with a Graphql/Prisma API backend.
This is primarily a TypeScript library.
npm install kapix-graphql-prisma-client
This library exposes TypeScript classes to build queries and mutation based on an entitiesModels
object you have to describe with a defineService()
function.
This library is best used inside a downloaded Kapix Project where everything surrounding this will generated for you.
The following code describes a simple 2 tables schema where a User has a Post List.
import type { NestedCreateInput, NestedListCreateInput, NestedUpdateInput, NestedListUpdateInput, WithOptional } from 'kapix-graphql-prisma-client-type'
import { GraphqlRequestQueryMode, defineModel } from 'kapix-graphql-prisma-client'
import { graphqlRequest } from '~/graphql'
export const entitiesModels = defineModel({
name: 'YouCanPutANameHere',
requestHandler: graphqlRequest
}, (defineEntityModel, defineEntityName) => ({
user: defineEntityModel(defineEntityName<Kapix.Entity.IUser, IUser, IUserWhereUniqueInput, IUserCreateInput, IUserUpdateInput>('user'), {
fields: {
id: {
mock: `uuid`,
type: `string`,
required: true,
isPk: true
},
posts: {
isRelation: true,
isList: true,
mode: `lazy`,
entityName: `post`,
targetProperty: `user`
}
},
endpoints: {
user: GraphqlRequestQueryMode.Get,
users: GraphqlRequestQueryMode.List,
count: GraphqlRequestQueryMode.Count,
createOneUser: GraphqlRequestQueryMode.CreateOne,
updateOneUser: GraphqlRequestQueryMode.UpdateOne,
deleteOneUser: GraphqlRequestQueryMode.DeleteOne,
createManyUser: GraphqlRequestQueryMode.CreateMany,
updateManyUser: GraphqlRequestQueryMode.UpdateMany,
deleteManyUser: GraphqlRequestQueryMode.DeleteMany
}
}),
post: defineEntityModel(defineEntityName<Kapix.Entity.IPost, IPost, IPostWhereUniqueInput, IPostCreateInput, IPostUpdateInput>('post'), {
fields: {
id: {
mock: `bigNumber`,
type: `number`,
required: true,
isPk: true
},
title: {
mock: `userName`,
type: `string`
},
user: {
isRelation: true,
mode: `lazy`,
targetProperty: `posts`,
fks: [`userId`],
required: true
},
userId: {
fetchByDefault: false,
isFk: true,
type: `string`,
targetProperty: `id`
}
},
endpoints: {
post: GraphqlRequestQueryMode.Get,
posts: GraphqlRequestQueryMode.List,
count: GraphqlRequestQueryMode.Count,
createOnePost: GraphqlRequestQueryMode.CreateOne,
updateOnePost: GraphqlRequestQueryMode.UpdateOne,
deleteOnePost: GraphqlRequestQueryMode.DeleteOne,
createManyPost: GraphqlRequestQueryMode.CreateMany,
updateManyPost: GraphqlRequestQueryMode.UpdateMany,
deleteManyPost: GraphqlRequestQueryMode.DeleteMany
}
})
}))
export interface IUser extends Kapix.Entity.IUser {}
export interface IUserWhereUniqueInput {
id?: string
}
export interface IUserCreateInput extends WithOptional<Omit<IUser, 'posts'>, 'id' > { posts?: NestedListCreateInput<Omit<IPostCreateInput, 'user' | 'userId'>, IPostWhereUniqueInput> }
export interface IUserUpdateInput extends Partial<Omit<IUser, 'posts'>> { posts?: NestedListUpdateInput<Omit<IPostUpdateInput, 'user' | 'userId'>, IPostCreateInput, IPostWhereUniqueInput> }
export interface IPost extends Kapix.Entity.IPost {}
export interface IPostWhereUniqueInput { id?: number }
export interface IPostCreateInput extends WithOptional<Omit<IPost, 'user'>, 'title' | 'id' | 'userId'> { user: NestedCreateInput<Omit<IUserCreateInput, 'posts'>, IUserWhereUniqueInput> }
export interface IPostUpdateInput extends Partial<Omit<IPost, 'user'>> { user?: NestedUpdateInput<Omit<IUserUpdateInput, 'posts'>, IUserCreateInput, IUserWhereUniqueInput> }
A simple query to get a post:
const fetchPost = await entitiesModels.Post.queries.post({
where: {
id: postId
},
select: {
...entitiesModels.post.defaultSelect
}
})
This will return the Post object you wanted, just as if you were writing:
`
query {
post(
where: {
id: '${postId}'
}
) {
id
title
}
}
`
But now you have typescript to guide you.
You can build a create input:
const postCreateInput = entitiesModels.post.factory.buildCreateInput({
title: 'firstPost'
})
And then use it for your mutation:
const createdPost = await entitiesModels.post.mutations.createOnePost({
data: postCreateInput,
select: {
...entitiesModels.post.defaultSelect,
userId: true,
user: true
}
})
This is the equivalent of:
`
mutation {
createOnePost(
data: {
title: '${title}'
}
) {
id
title
userId
user {
id
}
}
}
`
And the same for every endpoint and entity you have described in your entitiesModel
.
There is also a built-in data mocking service with a pseudo local storage, that you can use to emulate a real server.
To activate the mocking service, simply put mockData: true
in the defineService()
function args.
There are multiple other options you can give to customize the mocking, that you can pass like so:
mockData: true,
config: {
mock: {
maxItems
percentageOfNullWhenFieldNotRequired
randNumberOfItemsOptions
values
}
}
An example that will create 10 Post objects that will exist in the pseudo local storage:
const rideOffers = await entitiesModels.post.factory.mockItems({
id: true,
title: true,
user: {
id: true
}
}, {
min: 10,
max: 10
})
You can perform every action you want on these objects, as if it were a real server, so you can query and mutate on these created objects.
Copyright (c) 2020-2022 Stephane LASOUR
FAQs
Unknown package
The npm package kapix-graphql-prisma-client receives a total of 39 weekly downloads. As such, kapix-graphql-prisma-client popularity was classified as not popular.
We found that kapix-graphql-prisma-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.