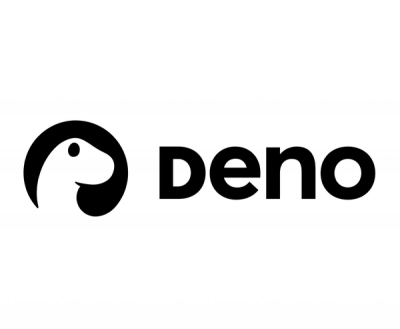
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
keep-or-skip
Advanced tools
This wrapper allows you to choose one or more middlewares to execute based on a certain condition. The aforementioned condition could be, for instance, a specific request value.
You can choose one of the following methods:
In your package.json add the following item to get the latest available version:
"keep-or-skip": "*"
or, if you need a specific version, just add an item like the following, specifying the version number:
"keep-or-skip": "1.0.0"
then launch this command:
npm install
Just launch this command:
npm install keep-or-skip --save
keepOrSkip(middlewares, predicate[, debug])
middlewares
<Function> | <Function[]> A middleware or an array of middlewares to handle dynamically.predicate
<Function> A function that returns a boolean value, by which, one or more middlewares will be executed or skipped. This function takes as input two optional parameters, the request
and the response
objects. If the predicate doesn't return a boolean value, the middleware/middlewares contained in the middlewares
parameter will be skipped.debug
<Boolean> An optional boolean parameter to enable a warning log which notifies that the predicate
parameter doesn't return a boolean value. Default: false.If the parameters' type does not match with those required, an error will be thrown. In pariticular, the error will be an instance of KeepOrSkipError.
Consider the following example(all the middlewares defined have demonstration purposes only):
const express = require('express')
const keepOrSkip = require('keep-or-skip')
function m1(req, res, next) {
if (!req.middlewares) {
req.middlewares = []
}
req.middlewares.push('executed m1')
next()
}
function m2(req, res, next) {
if (!req.middlewares) {
req.middlewares = []
}
req.middlewares.push('executed m2')
next()
}
function respond(req, res, next) {
res.status(200).json({
middlewares: req.middlewares
})
}
function setRandomValue(req, res, next) {
var max = 5
var min = -5
req.random = Math.random() * (max - min) + min
next()
}
app.get('/random',
setRandomValue,
keepOrSkip([m2, m1, m2], (req, res) => {
if (req.random < 0) {
// Execute [m2, m1, m2]
return true
}
// Skip [m2, m1, m2]
return false
}),
keepOrSkip([m1, m2], (req, res) => {
if (req.random >= 0) {
// Execute [m1, m2]
return true
}
// Skip [m1, m2]
return false
}),
respond
)
app.listen(3000)
http://localhost:3000/random
will produce the following result if the random
value is lower than 0:
{
"middlewares": [
"executed m2",
"executed m1",
"executed m2"
]
}
otherwise it will produce:
{
"middlewares": [
"executed m1",
"executed m2"
]
}
In the case the predicate
parameter doesn't return a boolean value, the
middleware/middlewares contained in the middlewares
parameter will be skipped.
In this situation, it's possible to log a warning by using the debug mode.
It's possible to activate the debug globally, directly on the imported module:
const keepOrSkip = require('keep-or-skip')
keepOrSkip.debug(true)
the above code will set the debug mode to true every time keepOrSkip
will be
used, unless otherwise specified at the time of use as shown in the following
example:
const keepOrSkip = require('keep-or-skip')
// Set debug mode globally.
keepOrSkip.debug(true)
/**
* Specifying the debug parameter at the time of use will overwrite the global debug variable.
*/
keepOrSkip(myMiddlewares, myPredicate, false) // Debug OFF.
keepOrSkip(myMiddlewares, myPredicate) // Debug ON because of the global debug variable.
1.0.0 / 2018-03-31
keep-or-skip
- dynamically execute or skip express middlewares.FAQs
Dynamically execute or skip express middlewares
The npm package keep-or-skip receives a total of 80 weekly downloads. As such, keep-or-skip popularity was classified as not popular.
We found that keep-or-skip demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.