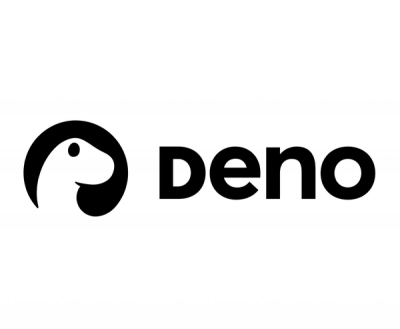
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
koa-core-server
Advanced tools
The koa server wrapper. That's all what you need to start build koa based server. ---
const Server = require('koa-core-server'); // Path to koa-core in node_modules
const path = require('path');
const routePath = path.join(__dirname, 'routers'); // dir where you routers live
const controllersPath = path.join(__dirname, 'controllers'); // dir where you controllers live
const dataAccessPath = path.join(__dirname, 'dataAccess'); // dir where you data access live
const server = new Server({
routePath,
controllersPath,
dataAccessPath,
clustering: {bool}, // by default is false, run you app with workers
loadersStatus: {bool}, // by default false, show loader statuses
});
server.use((ctx, next) => { // that how you can connect you middleware
console.log('hello from middleware');
next();
});
server.start();
const Router = require('koa-router');
module.exports = class HelloRouter extends Router {
constructor() {
super();
this.name = 'Hello router'; // optional if not define will be used file name"
this.rootPath = null; // optional by default will be using first part of the file name `hello.router -> /hello`
this.helloController = this.injector.get('helloCustomName'); // get controller from injector controller name defining in controller class via
// `this.name` or if not define use controller file name with postfix in camel case notation `user.controller -> userController`
}
load() {
this.get('/', this.helloController.getHello.bind(this.helloController)); // define you routers here
this.get('/by', this.helloController.sayBy.bind(this.helloController)); // define you routers here
return this; // return this is required in `this` lives you routers
}
};
module.exports = class HelloController {
constructor() {
this.name = 'helloCustomName'; // controller name will be set in controller injector and you can find controller by this name in injector or use
// controller file name with postfix in camel case notation `user.controller -> userController`
this.helloDataAccess = this.injector.get('helloDataAccess'); // get you data access for this controller by name defining in data access class `this.name` or use file name in camel case notation with postfix `hello.dataAccess -> helloDataAccess`
}
async getHello(ctx, next) { // controller methods uses in routers should be defined like plain koa function with async and ctx and next like parameters
ctx.response.body = await this.helloDataAccess.getHello();
}
async sayBy(ctx, next) { // controller methods uses in routers should be defined like plain koa function with async and ctx and next like parameters
ctx.response.body = await this.helloDataAccess.getGoodBy();
}
}
module.exports = class HelloDataAccess {
getHello() { // this methods will be using in controller
return Promise.resolve('hello');
}
getGoodBy() { // this methods will be using in controller
return Promise.resolve('good by');
}
}
FAQs
The koa server wrapper. That's all what you need to start build koa based server. ---
We found that koa-core-server demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.