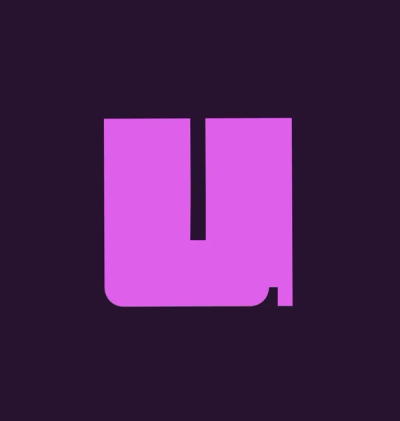
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
The `koa-mount` package is a middleware for the Koa framework that allows you to mount other Koa applications or middleware at specific paths. This is useful for structuring your application into smaller, more manageable pieces or for integrating third-party applications.
Mounting a Koa application at a specific path
This feature allows you to mount a sub-application at a specific path. In this example, requests to `/sub` will be handled by `subApp`.
const Koa = require('koa');
const mount = require('koa-mount');
const app = new Koa();
const subApp = new Koa();
subApp.use(async (ctx) => {
ctx.body = 'Hello from subApp!';
});
app.use(mount('/sub', subApp));
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
Mounting middleware at a specific path
This feature allows you to mount specific middleware at a given path. In this example, requests to `/middleware` will be handled by the `middleware` function.
const Koa = require('koa');
const mount = require('koa-mount');
const app = new Koa();
const middleware = async (ctx, next) => {
ctx.body = 'Hello from middleware!';
await next();
};
app.use(mount('/middleware', middleware));
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
The `koa-router` package provides a powerful routing solution for Koa applications. It allows you to define routes and their handlers, including support for nested routes and middleware. Unlike `koa-mount`, which is focused on mounting entire applications or middleware at specific paths, `koa-router` is more focused on defining and handling individual routes.
While not a Koa-specific package, `express` is a popular web framework for Node.js that includes built-in support for routing and middleware. It offers similar functionality to `koa-mount` in terms of mounting sub-applications and middleware, but it is designed for use with the Express framework rather than Koa.
Mount other Koa applications as middleware. The path
passed to mount()
is stripped
from the URL temporarily until the stack unwinds. This is useful for creating entire
apps or middleware that will function correctly regardless of which path segment(s)
they should operate on.
var mount = require('./');
var koa = require('koa');
// hello
var a = koa();
a.use(function(next){
return function *(){
yield next;
this.body = 'Hello';
}
});
// world
var b = koa();
b.use(function(next){
return function *(){
yield next;
this.body = 'World';
}
});
// app
var app = koa();
app.use(mount('/hello', a));
app.use(mount('/world', b));
app.listen(3000);
console.log('listening on port 3000');
Try the following requests:
$ GET /
Not Found
$ GET /hello
Hello
$ GET /world
World
$ npm install koa-mount
MIT
FAQs
Mounting middleware for koa
The npm package koa-mount receives a total of 65,052 weekly downloads. As such, koa-mount popularity was classified as popular.
We found that koa-mount demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.