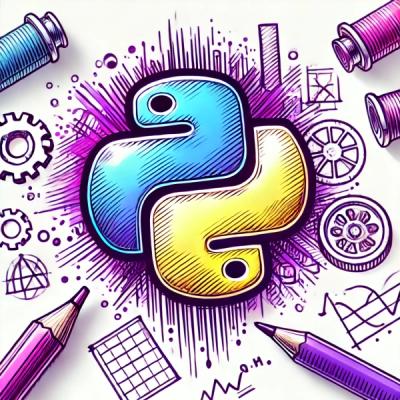
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
koreografeye
Advanced tools
A minimal orchestrator and policy executor component for the Mellon Researcher Pod project
This is a miniature implementation of an choreographer implementing the Orchestrator for a decentralized Web network specification using an N3 reaoner (such as EYE) as internal rule engine.
Koreografeye was created to facilitate running automated processes against Solid pods. Our main use case is monitoring the LDN Inbox of Solid Pods for new notifications and running scripts when new data arrives.
Koreografeye can be run on an input directory containing one or more RDF files (in Turtle or N3 format). For each of these files one or more N3 rule scripts can be executed. The results of this execution will be put in an output directory. If the N3 output contains Policy fragments, they can be executed using a policy executor.
Koreografeye is mainly targeted for processing Activity Streams (AS2) notifications. For each of these notifications N3 rules express one or more Policies what execution steps should be executed when receiving a AS2 notification of a particular shape. The orchestrator component reads all incoming AS2 notifications and executes the EYE reasoner (or EYE-JS, Roxi,... via configuration) on each of them and writes the results to an output directory. Policies are implemented as JavaScript plugins and can be execute by a policy executor.
A Policy is an RDF snippet that explains what should happen when running N3 rules against an AS2 input resource is successfull. Each policy has a name (identifier) and zero or more instances of an fno:Execution, which defines what JavaScript implementation should be executed. Optionally zero or more execution parameters can be provided for each execution.
The example below is an example of a Policy (prefix declarations omitted):
ex:MyDemoPolicy pol:policy [
a fno:Execution ;
fno:executes ex:helloWorld ;
ex:text "Hello, world!" ;
] .
This policy requests the execution of a ex:helloWorld
implementation with one parameter ex:text
with value "Hello, world!"
.
The ex:MyDemoPolicy
can be the result of an N3 rule (prefix declarations omitted):
{
?id a as:Announce.
}
=>
{
ex:MyDemoPolicy pol:policy [
a fno:Execution ;
fno:executes ex:helloWorld ;
ex:text "Hello, world!" ;
] .
}.
The N3 above states: "If we find an AS2 notification of type as:Announce
, then request executing the ex:MyDemoPolicy
.
npm install koreografeye -g
The experimental blogic , policy and solid examples require the Prolog version of EYE installed. We refer to the install instructions of this project for more information.
in
directoryrules
directorynpx orch --info --keep rules/* --in in --out out
to run the rules on alle in notification in the in
directory
--info
option to show informational messages--keep
option if you don't want to automatic clean the in
after processing notificationsout
directorynpm run orch
npx pol --info --keep --in out
to run the policy executor with all the processed notifications from the out
directory
--keep
option if you don't want to automatic clean the out
after processing notificationsnpm run pol
npm run orch:blogic
: this will execute the rules/blogic/00_demo.n3 rule which is a direct translation of rules/00_demo.n3 in the RDF Surfaces languagenpm run orch:policy
: this will read rules/blogic/00_policy.n3 (written in a small DSL language) and compile these rules into RDF Surfaces using a compiler available in rules/blogic/policydata/solid.jsonld
to the in
directorynpm run orch:solid
: this will check if a new resource actually exists on a backend server and send a Ntfy notifications (when running npm pol
)Small javascript example to execute Koreografeye using demo.ttl
and 00_demo.n3
.
const { EyeJsReasoner } = require('./dist/orchestrator/reasoner/EyeJsReasoner')
const { executePolicies } = require('./dist/policy/Executor');
const { parseAsN3Store, readText, storeAddPredicate, makeComponentsManager } = require('./dist/util');
const store = await parseAsN3Store('./data/demo.ttl'); // input graph
const rules = [readText('./rules/00_demo.n3')]; // array of n3 rules serialized as string
// add main subject and origin for the reasoner
const mainSubject = 'urn:uuid:42D2F3DC-0770-4F47-BF37-4F01E0382E32';
storeAddPredicate(store, 'https://www.example.org/ns/policy#mainSubject', mainSubject);
storeAddPredicate(store, 'https://www.example.org/ns/policy#origin', './data/demo.ttl');
// execute reasoning (orchestration)
const reasoner = new EyeJsReasoner([ "--quiet" , "--nope" , "--pass"])
const reasoningResult = await reasoner.reason(store, rules);
const manager = await makeComponentsManager('./config.jsonld','.');
// execute policies
await executePolicies(manager, reasoningResult);
Note: for this code to run, the project has to be compiled first (npm run build
).
Run the orchstrator with one or more N3 rule files on an input directory of notifications.
bin/orch [options] rule [rule ...]
Options:
Run a policy executor on one of the output files of the orchestrator
bin/pol [options] file
Options:
A Koreografeye implements the side-effects for the policy executor. These plugins handle sending
emails, updating Solid Pod, sending notifications, start engines and fire rockets. The plugins
require an entry in the config.jsonld
Components.js configuration file and a JavaScript/Typescript
implementation.
Each Koreografeye plugin is a javascript class that extends PolicyPlugin
and should implement
a policyTarget
function with the following signature:
export async function policyTarget(mainStore: N3.Store, policyStore: N3.Store , policy: IPolicyType) : Promise<boolean>;
where:
N3.Store
containing the parsed input file (without the policies)N3.Store
containing the current active parsed policyIPolicyType
structure containg all parameters for the requested policy:
N3.NamedNode | N3.BlankNode
: the policy node ()string
: path to the input filestring
: identifier of the policy instancestring
: identifier of the policy targetstring
: the activity identifier of the notificationstring
: path to the original notification input fileany { [key: string] : RDF.Term | undefined }
: key/value pairs of all arguments that were provided for the policyAn example IPolicyType
contents (in JSON):
After running rules/00_demo.n3 on data/demo.ttl
{
"node": {
"termType": "NamedNode",
"value": "http://example.org/MyDemoPolicy"
},
"path": "out/demo.ttl",
"policy": "bc_0_b1_b0_bn_1",
"target": "http://example.org/demoPlugin",
"args": {
"http://www.w3.org/1999/02/22-rdf-syntax-ns#type": {
"termType": "NamedNode",
"value": "https://w3id.org/function/ontology#Execution"
},
"https://w3id.org/function/ontology#executes": {
"termType": "NamedNode",
"value": "http://example.org/demoPlugin"
},
"http://example.org/param1": {
"termType": "Literal",
"value": "my@myself.and.i",
"language": "",
"datatype": {
"termType": "NamedNode",
"value": "http://www.w3.org/2001/XMLSchema#string"
}
},
"http://example.org/param2": {
"termType": "Literal",
"value": "you@yourself.edu",
"language": "",
"datatype": {
"termType": "NamedNode",
"value": "http://www.w3.org/2001/XMLSchema#string"
}
},
"http://example.org/body": {
"termType": "NamedNode",
"value": "urn:uuid:42D2F3DC-0770-4F47-BF37-4F01E0382E32"
}
},
"mainSubject": "urn:uuid:42D2F3DC-0770-4F47-BF37-4F01E0382E32",
"origin": "file:///var/folders/g8/czx2gjfs3_bbvvk1hjlcsj9m0000gn/T/in/demo.ttl"
}
correct
ex:MyDemoPolicy pol:policy [
a fno:Execution ;
fno:executes ex:demoPlugin ;
ex:param1 "my@myself.and.i" ;
ex:param2 "you@yourself.edu" ;
] .
incorrect
[ pol:policy [
a fno:Execution ;
fno:executes ex:demoPlugin ;
ex:param1 "my@myself.and.i" ;
ex:param2 "you@yourself.edu" ;
ex:subject "A new resource was created!" ;
ex:body ?s
] ] .
FAQs
A Solid choreography / orchestrator agent with a reasoning component
The npm package koreografeye receives a total of 23 weekly downloads. As such, koreografeye popularity was classified as not popular.
We found that koreografeye demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.