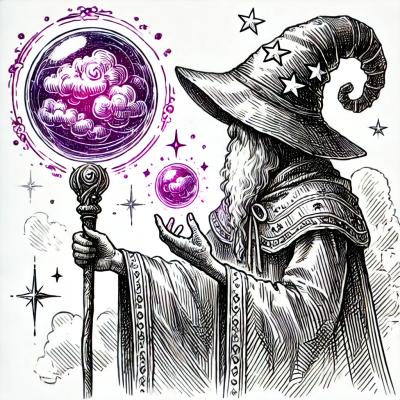
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Official API Wrapper for KSoft API, written in Node.js
installing...
npm install ksoft.js --save
ksoft.images.getRandomMeme();
ksoft.images.getRandomImage(tag);
ksoft.images.getTags();
ksoft.images.searchTags(tag);
ksoft.images.getImageFromId(snowflake);
ksoft.images.getRandomWikiHow(nsfw);
ksoft.images.getRandomCutePicture();
ksoft.images.getRandomNSFW();
ksoft.images.getRandomReddit(subReddit, removeNSFW, span);
ksoft.bans.add({
user: String,
mod: String,
user_name: String,
user_discriminator: String,
reason: String,
proof: String,
appeal_possible: Boolean,
});
ksoft.bans.getBanInfo(userID);
ksoft.bans.check(userID);
ksoft.bans.list(page, perPage);
ksoft.bans.getUpdate(epochDate);
ksoft.kumo.search(q, {
fast: Boolean,
more: Boolean,
mapZoom: Number,
includeMap: Boolean,
});
ksoft.kumo.getSimpleWeather(reportType, q, units, lang, icons);
ksoft.kumo.geoip(ip);
ksoft.kumo.convertCurrency(from, to, value);
ksoft.lyrics.search(q, {
textOnly: Boolean,
limit: Number,
});
ksoft.lyrics.getArtistById(id);
ksoft.lyrics.getAlbumById(id);
ksoft.lyrics.getTrackById(id);
This just requires adding a few things when we initiate ksoft.js
const Ksoft = require('ksoft.js');
const ksoft = new Ksoft('your ksoft token', {
useWebhooks: true,
port: 2000, // this is the port the http server is going to run on. This can be whatever port you want I am just using 2000 as an example
Authentication: 'your webhook authentication token',
});
ksoft.webhook.on('ready', info => {
console.log(info); // this will return the host your http server is running on. This is what you will give to ksoft to send the info to. { "host": "yourpublicip:theportyouspecified"}
});
that was just to get everything up and running now let's see how we can actually access that data. It's really simple :)
// this is an extension of the previous example. Everything works on events so you can simply do this
ksoft.webhook.on('ban', banData => {
console.log(banData); // there you simply get the banInfo sent from ksoft. All the event names are the same as on the ksoft documentation so if you want more info just go to https://docs.ksoft.si/api/webhooks
});
// the ksoft variable I am using is the initialized Ksoft class
const ban = new ksoft.CreateBan()
.setUserID('1234567892355822') // this is required
.setModID('44457845784574578')
.setUserName('blahblahblah')
.setUserDiscriminator('1234')
.setReason('testing123') // also required
.setProof('proof') // this is also required
.isAppealable(true);
ksoft.bans.add(ban).then(res => {
console.log(res); // { success: true}
});
Special thanks to sdf for helping me troubleshoot some stuff :)
FAQs
Official Node.js Wrapper for the KSoft.Si API.
The npm package ksoft.js receives a total of 21 weekly downloads. As such, ksoft.js popularity was classified as not popular.
We found that ksoft.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.