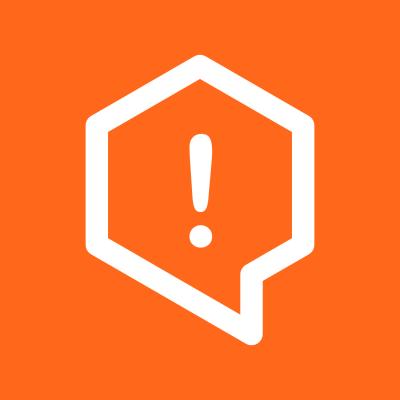
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
lazy-init-value
Advanced tools
A library for lazy initialization of values, supporting both synchronous and asynchronous scenarios.
A library for lazy initialization of values, supporting both synchronous and asynchronous scenarios.
npm install lazy-init-value
import { LazyInitValue } from 'lazy-init-value';
const value = new LazyInitValue(() => {
console.log('Initializing...');
return 42;
});
console.log(value.value); // Logs: "Initializing..." then "42"
console.log(value.value); // "42" (doesn't reinitialize)
import { LazyAsyncInitValue } from 'lazy-init-value';
const asyncValue = new LazyAsyncInitValue(async () => {
console.log('Initializing...');
await new Promise(resolve => setTimeout(resolve, 1000));
return 'Async Result';
});
console.log(await asyncValue.value()); // Logs: "Initializing..." then "Async Result"
console.log(await asyncValue.value()); // "Async Result" (doesn't reinitialize)
You can freeze a value by calling freeze()
method, then it cannot be reset(...)
.
When a value is set to be autoFreeze
, it will be frozen after initialization.
There is a static autoFreeze
property defaulting to true
in LazyInitValue
,
LazyAsyncInitValue
and LazyInitValueBase
to control whether the following values will be frozen
automatically after initialized. These three are binded to the same value.
And you can also control autoFreeze
for each instance when creating it:
import { LazyInitValue } from 'lazy-init-value';
const value1 = new LazyInitValue(() => 42, true); // will be auto-frozen, default
const value2 = new LazyInitValue(() => 42, false); // will not be frozen, can `reset(...)` later
value2.reset(() => 43);
console.log(value2.value); // 43
value2.freeze();
try {
value2.reset(() => 44);
} catch (e) {
console.log(e); // Cannot reset value after `freeze()`
}
console.log(LazyInitValue.autoFreeze); // true, default
const value3 = new LazyInitValue(() => 42); // will be auto-frozen
LazyInitValue.autoFreeze = false;
const value4 = new LazyInitValue(() => 42); // will not be frozen
import { LazyAsyncInitValue } from 'lazy-init-value';
console.log(LazyAsyncInitValue.autoFreeze); // false, same as `LazyInitValueBase.autoFreeze`
LazyInitValue
and LazyAsyncInitValue
inited: boolean
- Check if the value has been initializedfreeze()
- Then it cannot be reset
LazyInitValue<T>(initFn: () => T, autoFreeze?: boolean)
value: T
- Get the lazily initialized valuereset(initFn: () => T)
- Reset the initialization functionLazyAsyncInitValue<T>(initFn: () => Promise<T>, autoFreeze?: boolean)
value(): Promise<T> | T
- Get the value or a promise resolves to the lazily initialized valuevalue_sync: T | undefined
- Get the initialized value or trigger initializationiniting: boolean
- Check if async initialization is in progressreset(initFn: () => Promise<T>)
- Reset the initialization functionSo here is a tip when using LazyAsyncInitValue
:
await obj.value()
to get the value when you are not sure whether the value is initialized.obj.value_sync
to get the value when you ensure the value is already initialized. It's faster.This project is licensed under the MIT License.
FAQs
Lazy initialization of values, supporting both synchronous and asynchronous scenarios
The npm package lazy-init-value receives a total of 3 weekly downloads. As such, lazy-init-value popularity was classified as not popular.
We found that lazy-init-value demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.