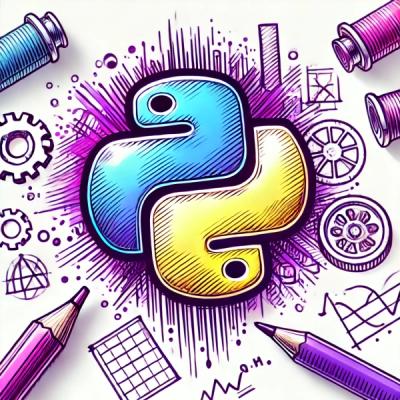
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
leaflet-editable
Advanced tools
# Leaflet.Editable
Make geometries editable in Leaflet.
This is not a plug and play UI, and will not. This is a minimal, lightweight, and fully extendable API to control editing of geometries. So you can easily build your own UI with your own needs and choices.
See the demo UI, an more examples below. This is also the drawing engine behind uMap.
Design keys:
Note: only geojson features are supported for now: Marker, Polyline, Polygon, and MultiPolygon/MultiPolylines (no Rectangle, Circle…)
## Install
You need Leaflet >= 0.7.3, and then include src/Leaflet.Editable.js
.
## Quick start
Allow Leaflet.Editable in the map options:
var map = L.map('map', {editable: true});
Then, to start editing an existing feature, call the enableEdit
method on it:
var polyline = L.polyline([[43.1, 1.2], [43.2, 1.3],[43.3, 1.2]]).addTo(map);
polyline.enableEdit();
If you want to draw a new line:
map.editTools.startPolyline(); // map.editTools has been created
// by passing editable: true option to the map
If you want to continue an existing line:
polyline.editor.continueForward();
// or
polyline.editor.continueBackward();
## Examples
## API
Leaflet.Editable is made to be fully extendable. You have three ways to customize the behaviour: using options, listening to events, or extending.
### L.Map
Leaflet.Editable add options and events to the L.Map
object.
#### Options
option name | default | usage |
---|---|---|
editable | false | Whether to create a L.Editable instance at map init or not. |
editOptions | {} | Options to pass to L.Editable when instanciating. |
event name | properties | usage |
---|---|---|
editable:created | layer | Fired when a new feature (Marker, Polyline…) has been created. |
editable:enable | layer | Fired when an existing feature is ready to be edited |
editable:disable | layer | Fired when an existing feature is not ready anymore to be edited |
editable:editing | layer | Fired as soon as any change is made to the feature geometry |
editable:drawing:start | layer | Fired when a feature is to be drawn |
editable:drawing:end | layer | Fired when a feature is not drawn anymore |
editable:drawing:cancel | layer | Fired when user cancel drawing while a feature is being drawn |
editable:drawing:commit | layer | Fired when user finish drawing a feature |
editable:drawing:click | layer | Fired when user click while drawing |
editable:vertex:ctrlclick | originalEvent, latlng, vertex, layer | Fired when a click having ctrlKey is issued on a vertex |
editable:vertex:shiftclick | originalEvent, latlng, vertex, layer | Fired when a click having shiftKey is issued on a vertex |
editable:vertex:altclick | originalEvent, latlng, vertex, layer | Fired when a click having altKey is issued on a vertex |
editable:vertex:contextmenu | originalEvent, latlng, vertex, layer | Fired when a contextmenu is issued on a vertex |
editable:vertex:deleted | originalEvent, latlng, vertex, layer | Fired after a vertex has been deleted by user |
editable:vertex:deleted | originalEvent, latlng, vertex, layer | Fired after a vertex has been deleted by user |
editable:vertex:drag | originalEvent, latlng, vertex, layer | Fired when a vertex is dragged by user |
editable:vertex:dragstart | originalEvent, latlng, vertex, layer | Fired before a vertex is dragged by user |
editable:vertex:dragend | originalEvent, latlng, vertex, layer | Fired after a vertex is dragged by user |
You will usually have only one instance of L.Editable, and generally the one
created automatically at map init: map.editTools
. It's the toolbox you will
use to create new features, and also the object you will configure with options.
Let's see them.
Note: you can pass them when creating a map using the editOptions
key.
option name | default | usage |
---|---|---|
polylineClass | L.Polyline | Class to be used when creating a new Polyline |
polygonClass | L.Polygon | Class to be used when creating a new Polygon |
markerClass | L.Marker | Class to be used when creating a new Marker |
drawingCSSClass | leaflet-editable-drawing | CSS class to be added to the map container while drawing |
editLayer | new L.LayerGroup() | Layer used to store edit tools (vertex, line guide…) |
featuresLayer | new L.LayerGroup() | Default layer used to store drawn features (marker, polyline…) |
vertexMarkerClass | L.Editable.VertexMarker | Class to be used as vertex, for path editing |
middleMarkerClass | L.Editable.MiddleMarker | Class to be used as middle vertex, pulled by the user to create a new point in the middle of a path |
polylineEditorClass | L.Editable.PolylineEditor | Class to be used as Polyline editor |
polygonEditorClass | L.Editable.PolygonEditor | Class to be used as Polygon editor |
markerEditorClass | L.Editable.MarkerEditor | Class to be used as Marker editor |
lineGuideOptions | {} | Options to be passed to the line guides |
skipMiddleMarkers | null | Set this to true if you don't want middle markers |
Those are the public methods. You will generally access them by the map.editTools
instance:
map.editTools.startPolyline();
method name | params | return | usage |
---|---|---|---|
startPolyline | latlng* | created L.Polyline instance | Start drawing a polyline. If latlng is given, a first point will be added. In any case, continuing on user click. |
startPolygon | latlng* | created L.Polygon instance | Start drawing a polygon. If latlng is given, a first point will be added. In any case, continuing on user click. |
startMarker | latlng* | created L.Marker instance | Start adding a marker. If latlng is given, the marker will be shown first at this point. In any case, it will follow the user mouse, and will have a final latlng on next click (or touch). |
stopDrawing | — | — | When you need to stop any ongoing drawing, without needing to know which editor is active. |
Same as L.Map.
The marker used to handle path vertex. You will usually interact with a VertexMarker
instance when listening for events like editable:vertex:ctrlclick
.
Those are its public methods.
method name | params | return | usage |
---|---|---|---|
delete | — | — | Delete a vertex and the related latlng. |
getIndex | — | int | Get the index of the current vertex among others of the same LatLngs group. |
getLastIndex | — | int | Get last vertex index of the LatLngs group of the current vertex. |
getPrevious | — | VertexMarker instance | Get the previous VertexMarker in the same LatLngs group. |
getNext | — | VertexMarker instance | Get the next VertexMarker in the same LatLngs group. |
When editing a feature (marker, polyline…), an editor is attached to it. This editor basically knows how to handle the edition.
It has some public methods:
method name | params | return | usage |
---|---|---|---|
enable | — | this | Set up the drawing tools for the feature to be editable. |
disable | — | this | Remove editing tools. |
Inherit from L.Editable.BaseEditor
.
Inherit from L.Editable.BaseEditor
.
Inherited by L.Editable.PolylineEditor
and L.Editable.PolygonEditor
.
Interesting new method:
method name | params | return | usage |
---|---|---|---|
reset | — | — | Rebuild edit elements (vertex, middlemarker, etc.) |
### L.Editable.PolylineEditor
Inherit from L.Editable.PathEditor
.
Useful specific methods:
method name | params | return | usage |
---|---|---|---|
continueForward | — | — | Set up drawing tools to continue the line forward |
continueBackward | — | — | Set up drawing tools to continue the line backward |
### L.Editable.PolygonEditor
Inherit from L.Editable.PathEditor
.
method name | params | return | usage |
---|---|---|---|
newHole | latlng* | — | Set up drawing tools for creating a new hole on the polygon. If the latlng param is given, a first point is created. |
EditableMixin
is included to L.Polyline
, L.Polygon
and L.Marker
. It
adds the following methods to them.
When editing is enabled, the editor is accessible on the instance with the
editor
property.
#### Methods
method name | params | return | usage |
---|---|---|---|
enableEdit | — | related editor instance | Enable editing, by creating an editor if not existing, and then calling enable on it |
disableEdit | — | — | Disable editing, also remove the editor property reference. |
toggleEdit | — | — | Enable or disable editing, according to current status. |
editEnabled | — | boolean | Return true if current instance has an editor attached, and this editor is enabled. |
Some events are also fired on the feature itself.
event name | properties | usage |
---|---|---|
editable:drawing:start | layer | Fired when a feature is to be drawn |
editable:drawing:end | layer | Fired when a feature is not drawn anymore |
editable:drawing:cancel | layer | Fired when user cancel drawing while a feature is being drawn |
editable:drawing:commit | layer | Fired when user finish drawing a feature |
editable:drawing:click | layer | Fired when user click while drawing |
editable:vertex:ctrlclick | originalEvent, latlng, vertex, layer | Fired when a click having ctrlKey is issued on a vertex |
editable:vertex:shiftclick | originalEvent, latlng, vertex, layer | Fired when a click having shiftKey is issued on a vertex |
editable:vertex:altclick | originalEvent, latlng, vertex, layer | Fired when a click having altKey is issued on a vertex |
editable:vertex:contextmenu | originalEvent, latlng, vertex, layer | Fired when a contextmenu is issued on a vertex |
editable:vertex:deleted | originalEvent, latlng, vertex, layer | Fired after a vertex has been deleted by user |
## Licence
Leaflet.Editable
is released under the WTFPL licence.
FAQs
Make geometries editable in Leaflet
The npm package leaflet-editable receives a total of 6,033 weekly downloads. As such, leaflet-editable popularity was classified as popular.
We found that leaflet-editable demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.