leaflet-tile-loading-progress-control
Advanced tools
Comparing version 0.0.3 to 1.0.0
{ | ||
"name": "leaflet-tile-loading-progress-control", | ||
"version": "0.0.3", | ||
"version": "1.0.0", | ||
"homepage": "https://github.com/jbccollins/leaflet-tile-loading-progress-control", | ||
@@ -6,0 +6,0 @@ "authors": [ |
@@ -0,124 +1,132 @@ | ||
/* @preserve | ||
* Leaflet Control TileLoadingProgress 1.0.0 | ||
* https://github.com/jbccollins/leaflet-tile-loading-progress-control | ||
* | ||
* Copyright (c) 2018 James Collins | ||
* All rights reserved. | ||
*/ | ||
this.L = this.L || {}; | ||
this.L.Control = this.L.Control || {}; | ||
this.L.Control.TileLoadingProgress = (function (L) { | ||
'use strict'; | ||
'use strict'; | ||
L = L && L.hasOwnProperty('default') ? L['default'] : L; | ||
L = L && L.hasOwnProperty('default') ? L['default'] : L; | ||
var Control = { | ||
class: L.Control.extend({ | ||
options: { | ||
leafletElt: null, | ||
position: 'topright' | ||
}, | ||
initialize: function (options) { | ||
// constructor | ||
this.handleLoadingStatusUpdate = this.handleLoadingStatusUpdate.bind(this); | ||
this.handleTileLoadStart = this.handleTileLoadStart.bind(this); | ||
this.handleTileUnload = this.handleTileUnload.bind(this); | ||
this.handleTileLoad = this.handleTileLoad.bind(this); | ||
this.handleLayerLoading = this.handleLayerLoading.bind(this); | ||
this.handleTileError = this.handleTileError.bind(this); | ||
this.handleLayerLoad = this.handleLayerLoad.bind(this); | ||
this.getTileStatusCounts = this.getTileStatusCounts.bind(this); | ||
L.Util.setOptions(this, options); | ||
}, | ||
onAdd: function (map) { | ||
var container = L.DomUtil.create('div', 'leaflet-control-progress-bar'); | ||
var loadingContainer = L.DomUtil.create('div', 'loading-container'); | ||
var loadingBackground = L.DomUtil.create('div', 'loading-background'); | ||
var loadingForeground = L.DomUtil.create('div', 'loading-foreground'); | ||
var loadingText = L.DomUtil.create('div', 'loading-text'); | ||
loadingContainer.appendChild(loadingBackground); | ||
loadingContainer.appendChild(loadingForeground); | ||
container.appendChild(loadingContainer); | ||
container.appendChild(loadingText); | ||
for (var key in this.options.leafletElt._layers) { | ||
var layer = this.options.leafletElt._layers[key]; | ||
layer.on('tileloadstart', this.handleTileLoadStart); | ||
layer.on('tileunload', this.handleTileUnload); | ||
layer.on('tileload', this.handleTileLoad); | ||
layer.on('loading', this.handleLayerLoading); | ||
layer.on('tileerror', this.handleTileError); | ||
layer.on('load', this.handleLayerLoad); | ||
} | ||
this.loadingForegroundElt = loadingForeground; | ||
this.container = container; | ||
this.loadingText = loadingText; | ||
return container; | ||
}, | ||
onRemove: function (map) { | ||
// when removed | ||
}, | ||
handleLoadingStatusUpdate: function () { | ||
var status = null; | ||
var status = { | ||
var Control = { | ||
class: L.Control.extend({ | ||
options: { | ||
leafletElt: null, | ||
position: 'topright' | ||
}, | ||
initialize: function (options) { | ||
// constructor | ||
this.handleLoadingStatusUpdate = this.handleLoadingStatusUpdate.bind(this); | ||
this.handleTileLoadStart = this.handleTileLoadStart.bind(this); | ||
this.handleTileUnload = this.handleTileUnload.bind(this); | ||
this.handleTileLoad = this.handleTileLoad.bind(this); | ||
this.handleLayerLoading = this.handleLayerLoading.bind(this); | ||
this.handleTileError = this.handleTileError.bind(this); | ||
this.handleLayerLoad = this.handleLayerLoad.bind(this); | ||
this.getTileStatusCounts = this.getTileStatusCounts.bind(this); | ||
L.Util.setOptions(this, options); | ||
}, | ||
onAdd: function (map) { | ||
var container = L.DomUtil.create('div', 'leaflet-control-progress-bar'); | ||
var loadingContainer = L.DomUtil.create('div', 'loading-container'); | ||
var loadingBackground = L.DomUtil.create('div', 'loading-background'); | ||
var loadingForeground = L.DomUtil.create('div', 'loading-foreground'); | ||
var loadingText = L.DomUtil.create('div', 'loading-text'); | ||
loadingContainer.appendChild(loadingBackground); | ||
loadingContainer.appendChild(loadingForeground); | ||
container.appendChild(loadingContainer); | ||
container.appendChild(loadingText); | ||
for (var key in this.options.leafletElt._layers) { | ||
var layer = this.options.leafletElt._layers[key]; | ||
layer.on('tileloadstart', this.handleTileLoadStart); | ||
layer.on('tileunload', this.handleTileUnload); | ||
layer.on('tileload', this.handleTileLoad); | ||
layer.on('loading', this.handleLayerLoading); | ||
layer.on('tileerror', this.handleTileError); | ||
layer.on('load', this.handleLayerLoad); | ||
} | ||
this.loadingForegroundElt = loadingForeground; | ||
this.container = container; | ||
this.loadingText = loadingText; | ||
return container; | ||
}, | ||
onRemove: function (map) { | ||
// when removed | ||
}, | ||
handleLoadingStatusUpdate: function () { | ||
var status = null; | ||
var status = { | ||
loading: 0, | ||
loaded: 0 | ||
}; | ||
for (var key in this.options.leafletElt._layers) { | ||
var layer = this.options.leafletElt._layers[key]; | ||
var layerStatus = this.getTileStatusCounts(layer); | ||
status.loading += layerStatus.loading; | ||
status.loaded += layerStatus.loaded; | ||
} | ||
var numTiles = status.loading + status.loaded; | ||
var w = numTiles ? status.loaded / numTiles : 0; | ||
var percent = w * 100; | ||
this.loadingForegroundElt.style.width = percent + "%"; | ||
if (w === 1) { | ||
this.container.style.opacity = 0; | ||
} else { | ||
this.container.style.opacity = 1; | ||
} | ||
this.loadingText.innerHTML = "Loading Tiles (" + Math.floor(percent) + "%)"; | ||
}, | ||
handleLayerLoading: function (e) { | ||
this.handleLoadingStatusUpdate(); | ||
}, | ||
handleTileLoadStart: function (e) { | ||
this.handleLoadingStatusUpdate(); | ||
}, | ||
handleTileLoad: function (e) { | ||
this.handleLoadingStatusUpdate(); | ||
}, | ||
handleTileError: function (e) { | ||
this.handleLoadingStatusUpdate(); | ||
}, | ||
handleTileUnload: function (e) { | ||
this.handleLoadingStatusUpdate(); | ||
}, | ||
handleLayerLoad: function (e) { | ||
//console.log('loaded'); | ||
}, | ||
getTileStatusCounts: function (l) { | ||
var status = { | ||
loaded: 0, | ||
loading: 0, | ||
loaded: 0 | ||
}; | ||
for (var key in this.options.leafletElt._layers) { | ||
var layer = this.options.leafletElt._layers[key]; | ||
var layerStatus = this.getTileStatusCounts(layer); | ||
status.loading += layerStatus.loading; | ||
status.loaded += layerStatus.loaded; | ||
}; | ||
for (var key in l._tiles) { | ||
if (l._tiles[key].loaded) { | ||
status.loaded++; | ||
} else { | ||
status.loading++; | ||
} | ||
} | ||
return status; | ||
} | ||
var numTiles = status.loading + status.loaded; | ||
var w = numTiles ? status.loaded / numTiles : 0; | ||
var percent = w * 100; | ||
this.loadingForegroundElt.style.width = percent + "%"; | ||
if (w === 1) { | ||
this.container.style.opacity = 0; | ||
} else { | ||
this.container.style.opacity = 1; | ||
} | ||
this.loadingText.innerHTML = `Loading Tiles (${Math.floor(percent)}%)`; | ||
}, | ||
handleLayerLoading: function (e) { | ||
this.handleLoadingStatusUpdate(); | ||
}, | ||
handleTileLoadStart: function (e) { | ||
this.handleLoadingStatusUpdate(); | ||
}, | ||
handleTileLoad: function (e) { | ||
this.handleLoadingStatusUpdate(); | ||
}, | ||
handleTileError: function (e) { | ||
this.handleLoadingStatusUpdate(); | ||
}, | ||
handleTileUnload: function (e) { | ||
this.handleLoadingStatusUpdate(); | ||
}, | ||
handleLayerLoad: function (e) { | ||
//console.log('loaded'); | ||
}, | ||
getTileStatusCounts: function (l) { | ||
var status = { | ||
loaded: 0, | ||
loading: 0, | ||
}; | ||
for (var key in l._tiles) { | ||
if (l._tiles[key].loaded) { | ||
status.loaded++; | ||
} else { | ||
status.loading++; | ||
} | ||
} | ||
return status; | ||
}), | ||
factory: function(options) { | ||
return new L.Control.TileLoadingProgress(options); | ||
} | ||
}), | ||
factory: function(options) { | ||
return new L.Control.TileLoadingProgress(options); | ||
} | ||
}; | ||
}; | ||
var TileLoadingProgress = L.Util.extend(Control.class, {}); | ||
var TileLoadingProgress = L.Util.extend(Control.class, {}); | ||
L.Util.extend(L.Control, { | ||
TileLoadingProgress: TileLoadingProgress, | ||
tileLoadingProgress: Control.factory | ||
}); | ||
L.Util.extend(L.Control, { | ||
TileLoadingProgress: TileLoadingProgress, | ||
tileLoadingProgress: Control.factory | ||
}); | ||
return TileLoadingProgress; | ||
return TileLoadingProgress; | ||
}(L)); | ||
//# sourceMappingURL=Control.TileLoadingProgress.js.map |
{ | ||
"name": "leaflet-tile-loading-progress-control", | ||
"version": "0.0.3", | ||
"version": "1.0.0", | ||
"description": "A leaflet control that indicates tile loading progress for a group of tile layers", | ||
@@ -9,3 +9,3 @@ "main": "dist/Control.TileLoadingProgress.js", | ||
"build": "npm run build:js && npm run build:css", | ||
"build:js": "rollup --output.format=iife --name=L.Control.TileLoadingProgress --globals=leaflet:L src/index.js --output.file=dist/Control.TileLoadingProgress.js --sourcemap", | ||
"build:js": "rollup -c", | ||
"build:css": "cpr Control.TileLoadingProgress.css dist/Control.TileLoadingProgress.css --overwrite", | ||
@@ -17,3 +17,4 @@ "test": "npm run lint", | ||
"publish": "sh ./scripts/publish.sh", | ||
"postpublish": "sh ./scripts/postpublish.sh" | ||
"postpublish": "sh ./scripts/postpublish.sh", | ||
"dev": "rollup -c -w" | ||
}, | ||
@@ -33,4 +34,3 @@ "repository": { | ||
}, | ||
"dependencies": {}, | ||
"devDependencies": { | ||
"dependencies": { | ||
"cpr": "^3.0.1", | ||
@@ -40,4 +40,4 @@ "eslint": "^4.15.0", | ||
"prettier": "^1.9.2", | ||
"rollup": "^0.53.2" | ||
"rollup": "^0.64.1" | ||
} | ||
} |
# leaflet-tile-loading-progress-control | ||
A leaflet control that indicates tile loading progress for a group of tile layers. | ||
A leaflet control that indicates tile loading progress for a group of tile layers. This can be useful when you have a lot of tile layers or tiles that frequently refresh. | ||
My particualar use case was for a looping precipitation radar that was built using ten tile layers that each needed to be updated every five minutes. | ||
# Usage | ||
```javascript | ||
import 'leaflet-tile-loading-progress-control'; | ||
import 'leaflet-tile-loading-progress-control/dist/Control.TileLoadingProgress.css'; | ||
const tileLayer1 = L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png') | ||
const tileLayer2 = L.tileLayer('http://tile.stamen.com/toner/{z}/{x}/{y}.png'); | ||
const tileLayers = L.layerGroup([tileLayer1, tileLayer2]); | ||
const tileLoadingProgress = new L.Control.TileLoadingProgress({ | ||
leafletElt: tileLayers, | ||
position: 'bottomleft' | ||
}); | ||
tileLoadingProgress.addTo(map); | ||
``` | ||
## Options | ||
| Option | Type | Description | | ||
| --------------- | ---------------- | ----------------- | ----------- | | ||
| `leafletElt` | Leaflet LayerGroup | Group of tile layers tracked by the loading progress control. | | ||
# Example GIF | ||
from https://us-weather-monitor.herokuapp.com/ | ||
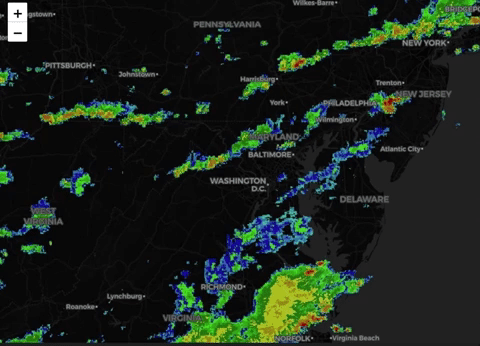 |
@@ -68,3 +68,3 @@ import L from 'leaflet'; | ||
} | ||
this.loadingText.innerHTML = `Loading Tiles (${Math.floor(percent)}%)` | ||
this.loadingText.innerHTML = "Loading Tiles (" + Math.floor(percent) + "%)"; | ||
}, | ||
@@ -71,0 +71,0 @@ handleLayerLoading: function (e) { |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
117035
0
16
346
1
32
5
+ Addedcpr@^3.0.1
+ Addedeslint@^4.15.0
+ Addedprettier@^1.9.2
+ Addedrollup@^0.64.1
+ Added@types/estree@0.0.39(transitive)
+ Added@types/node@22.9.3(transitive)
+ Addedacorn@3.3.05.7.4(transitive)
+ Addedacorn-jsx@3.0.1(transitive)
+ Addedajv@5.5.2(transitive)
+ Addedajv-keywords@2.1.1(transitive)
+ Addedansi-escapes@3.2.0(transitive)
+ Addedansi-regex@2.1.13.0.1(transitive)
+ Addedansi-styles@2.2.13.2.1(transitive)
+ Addedargparse@1.0.10(transitive)
+ Addedbabel-code-frame@6.26.0(transitive)
+ Addedbalanced-match@1.0.2(transitive)
+ Addedbrace-expansion@1.1.11(transitive)
+ Addedbuffer-from@1.1.2(transitive)
+ Addedcaller-path@0.1.0(transitive)
+ Addedcallsites@0.2.0(transitive)
+ Addedchalk@1.1.32.4.2(transitive)
+ Addedchardet@0.4.2(transitive)
+ Addedcircular-json@0.3.3(transitive)
+ Addedcli-cursor@2.1.0(transitive)
+ Addedcli-width@2.2.1(transitive)
+ Addedco@4.6.0(transitive)
+ Addedcolor-convert@1.9.3(transitive)
+ Addedcolor-name@1.1.3(transitive)
+ Addedconcat-map@0.0.1(transitive)
+ Addedconcat-stream@1.6.2(transitive)
+ Addedcore-util-is@1.0.3(transitive)
+ Addedcpr@3.0.1(transitive)
+ Addedcross-spawn@5.1.0(transitive)
+ Addeddebug@3.2.7(transitive)
+ Addeddeep-is@0.1.4(transitive)
+ Addeddoctrine@2.1.0(transitive)
+ Addedescape-string-regexp@1.0.5(transitive)
+ Addedeslint@4.19.1(transitive)
+ Addedeslint-plugin-prettier@2.7.0(transitive)
+ Addedeslint-scope@3.7.3(transitive)
+ Addedeslint-visitor-keys@1.3.0(transitive)
+ Addedespree@3.5.4(transitive)
+ Addedesprima@4.0.1(transitive)
+ Addedesquery@1.6.0(transitive)
+ Addedesrecurse@4.3.0(transitive)
+ Addedestraverse@4.3.05.3.0(transitive)
+ Addedesutils@2.0.3(transitive)
+ Addedexternal-editor@2.2.0(transitive)
+ Addedfast-deep-equal@1.1.0(transitive)
+ Addedfast-diff@1.3.0(transitive)
+ Addedfast-json-stable-stringify@2.1.0(transitive)
+ Addedfast-levenshtein@2.0.6(transitive)
+ Addedfigures@2.0.0(transitive)
+ Addedfile-entry-cache@2.0.0(transitive)
+ Addedflat-cache@1.3.4(transitive)
+ Addedfs.realpath@1.0.0(transitive)
+ Addedfunctional-red-black-tree@1.0.1(transitive)
+ Addedglob@7.2.3(transitive)
+ Addedglobals@11.12.0(transitive)
+ Addedgraceful-fs@4.2.11(transitive)
+ Addedhas-ansi@2.0.0(transitive)
+ Addedhas-flag@3.0.0(transitive)
+ Addediconv-lite@0.4.24(transitive)
+ Addedignore@3.3.10(transitive)
+ Addedimurmurhash@0.1.4(transitive)
+ Addedinflight@1.0.6(transitive)
+ Addedinherits@2.0.4(transitive)
+ Addedinquirer@3.3.0(transitive)
+ Addedis-fullwidth-code-point@2.0.0(transitive)
+ Addedis-resolvable@1.1.0(transitive)
+ Addedisarray@1.0.0(transitive)
+ Addedisexe@2.0.0(transitive)
+ Addedjest-docblock@21.2.0(transitive)
+ Addedjs-tokens@3.0.2(transitive)
+ Addedjs-yaml@3.14.1(transitive)
+ Addedjson-schema-traverse@0.3.1(transitive)
+ Addedjson-stable-stringify-without-jsonify@1.0.1(transitive)
+ Addedlevn@0.3.0(transitive)
+ Addedlodash@4.17.21(transitive)
+ Addedlru-cache@4.1.5(transitive)
+ Addedmimic-fn@1.2.0(transitive)
+ Addedminimatch@3.1.2(transitive)
+ Addedminimist@1.2.8(transitive)
+ Addedmkdirp@0.5.6(transitive)
+ Addedms@2.1.3(transitive)
+ Addedmute-stream@0.0.7(transitive)
+ Addednatural-compare@1.4.0(transitive)
+ Addedobject-assign@4.1.1(transitive)
+ Addedonce@1.4.0(transitive)
+ Addedonetime@2.0.1(transitive)
+ Addedoptionator@0.8.3(transitive)
+ Addedos-tmpdir@1.0.2(transitive)
+ Addedpath-is-absolute@1.0.1(transitive)
+ Addedpath-is-inside@1.0.2(transitive)
+ Addedpluralize@7.0.0(transitive)
+ Addedprelude-ls@1.1.2(transitive)
+ Addedprettier@1.19.1(transitive)
+ Addedprocess-nextick-args@2.0.1(transitive)
+ Addedprogress@2.0.3(transitive)
+ Addedpseudomap@1.0.2(transitive)
+ Addedreadable-stream@2.3.8(transitive)
+ Addedregexpp@1.1.0(transitive)
+ Addedrequire-uncached@1.0.3(transitive)
+ Addedresolve-from@1.0.1(transitive)
+ Addedrestore-cursor@2.0.0(transitive)
+ Addedrimraf@2.6.32.7.1(transitive)
+ Addedrollup@0.64.1(transitive)
+ Addedrun-async@2.4.1(transitive)
+ Addedrx-lite@4.0.8(transitive)
+ Addedrx-lite-aggregates@4.0.8(transitive)
+ Addedsafe-buffer@5.1.2(transitive)
+ Addedsafer-buffer@2.1.2(transitive)
+ Addedsemver@5.7.2(transitive)
+ Addedshebang-command@1.2.0(transitive)
+ Addedshebang-regex@1.0.0(transitive)
+ Addedsignal-exit@3.0.7(transitive)
+ Addedslice-ansi@1.0.0(transitive)
+ Addedsprintf-js@1.0.3(transitive)
+ Addedstring-width@2.1.1(transitive)
+ Addedstring_decoder@1.1.1(transitive)
+ Addedstrip-ansi@3.0.14.0.0(transitive)
+ Addedstrip-json-comments@2.0.1(transitive)
+ Addedsupports-color@2.0.05.5.0(transitive)
+ Addedtable@4.0.2(transitive)
+ Addedtext-table@0.2.0(transitive)
+ Addedthrough@2.3.8(transitive)
+ Addedtmp@0.0.33(transitive)
+ Addedtype-check@0.3.2(transitive)
+ Addedtypedarray@0.0.6(transitive)
+ Addedundici-types@6.19.8(transitive)
+ Addedutil-deprecate@1.0.2(transitive)
+ Addedwhich@1.3.1(transitive)
+ Addedword-wrap@1.2.5(transitive)
+ Addedwrappy@1.0.2(transitive)
+ Addedwrite@0.2.1(transitive)
+ Addedyallist@2.1.2(transitive)