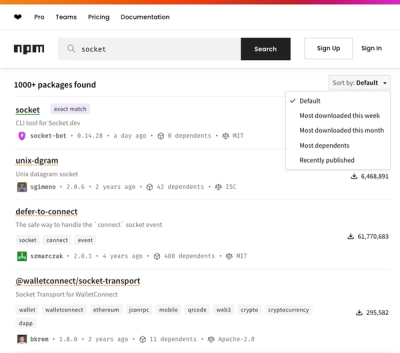
Security News
npm Updates Search Experience with New Objective Sorting Options
npm has a revamped search experience with new, more transparent sorting options—Relevance, Downloads, Dependents, and Publish Date.
lessthan3 development server
TODO: lessthan3 production server
TODO: lessthan3 package manager (lpm)
assists with create a package server
assists with creating new packages
assists with package submission to hosted environment
assists with package deployment to personal lt3 package server
app.coffee
class exports.App extends lt3.App
load: (next) ->
next()
template: ->
div class: 'pages'
package.cson
{ author: 'Bryant Williams' changelog: '0.1.1': 'test app' contact: 'bryant@lessthan3.com' description: 'My First App' id: 'bryant-cool-app' pages: index: title: 'string' monkey: kind: 'string' name: 'string' description: 'string' image: 'string' name: 'My First App' type: 'app' version: '0.1.1' }
style.styl
.exports .some-div padding 36px 50px
pages/monkey.coffee
class exports.Page extends lt3.Page
events: 'click .title': 'onTitleClick'
onTitleClick: (e) -> el = $(e.currentTarget) console.log el.text()
delegateEvents: -> super() $(window).bind 'resize', @onWindowResize
undelegateEvents: -> super() $(window).unbind 'resize', @onWindowResize
load: (next) -> @$el.html 'loading...' $.ajax { url: 'http://domain.com/api/get/ice-cream' success: (data) => @_.extra = data next() }
render: -> super() @$el.find('content').css { color: '#000' }
template: -> h2 class: 'title', -> @title
div class: 'image', ->
img src: @image
div class: 'content', ->
@content
{ author: 'Your Name' category: 'footer' changelog: 'major.minor.patch': ‘initial commit' contact: ‘me@domain.com' description: 'description of this package' id: 'namespace-name' name: 'readable name’ pages: type1: {DATA_MODEL_SCHEMA} type2: {DATA_MODEL_SCHEMA} settings: {DATA_MODEL_SCHEMA} tags: [ ‘tag1’ ‘tag2’ ] type: 'app' version: 'major.minor.patch' }
Full Verbose Schema Example settings: str: {type: ‘string’} str_enum: {type: ‘string’, enum: [‘foo’, ‘bar’]} str_long: {type: ‘string’, editor: ‘textarea’} bool: {type: ‘boolean’} int: {type: ‘integer’} arr: { type: ‘array’ legend: ‘foo’ items: foo: {type: ‘string’} bar: {type: ‘string’} } obj: { type: ‘object’ properties: foo: {type: ‘string’} bar: {type: ‘string’} }
3 Rules to simplify your syntax
Let’s now look at the above example in our simplified syntax settings: str: ‘string’ str_enum: {type: ‘string’, enum: [‘foo’, ‘bar’]} str_long: {type: ‘string’, editor: ‘textarea’} bool: ‘boolean’ int: ‘integer’ arr: [ foo: {type: ‘string’, legend: true} bar: ‘string’ ] obj: { foo: {type: ‘string’} bar: {type: ‘string’} }
express = require 'express' lessthan3 = require '../../lib/server' # require 'lessthan3' pkg = require './package'
app = express() app.use express.logger() app.use express.bodyParser() app.use express.methodOverride() app.use express.cookieParser() app.use lessthan3 { pkg_dir: "#{__dirname}/pkg" } app.use app.router app.use express.errorHandler {dumpExceptions: true, showStack: true}
app.listen pkg.config.port console.log "listening: #{pkg.config.port}"
http://localhost:3001/pkg/bryant-cool-app/0.1.1/package.json
http://localhost:3001/pkg/bryant-cool-app/0.1.1/main.js
http://localhost:3001/pkg/bryant-cool-app/0.1.1/style.css
http://localhost:3001/pkg/bryant-cool-app/0.1.1/public/test.txt
http://localhost:3001/pkg/bryant-cool-app/0.1.1/api/foo
module.exports = foo: -> # this will cache /pkg/bryant-cool-app/0.1.1/api/foo @cache {age: '10 minutes'}, (next) => next 'bar'
hello: ->
@res.send 'world'
ping: ->
# this will cache /pkg/bryant-cool-app/0.1.1/api/ping?hello=world
@cache {age: '10 minutes', qs: true}, (next) =>
next 'ack'
{ cache: (options, next) -> # options.age can be '10 minutes' or 600 # options.qs can be true|false to include the query params in the cache key # passing data to "next" will cache and return the data req: req res: res }
FAQs
LessThan3 Developer Tools
The npm package lessthan3 receives a total of 14 weekly downloads. As such, lessthan3 popularity was classified as not popular.
We found that lessthan3 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm has a revamped search experience with new, more transparent sorting options—Relevance, Downloads, Dependents, and Publish Date.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.