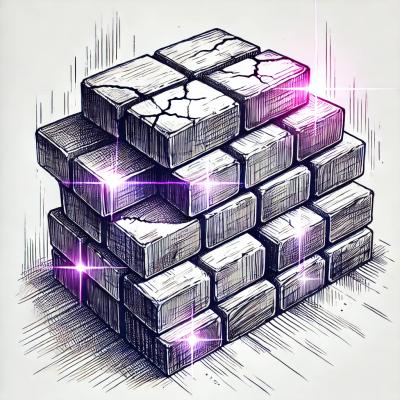
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Query the Live for Speed API in your projects.
Note: This package does not yet support authorization flows with PKCE and is therefore only suitable for secure applications at this point in time. Do not use this module in insecure or single page applications (SPAs).
Add this module to your project with npm install lfs-api
or yarn add lfs-api
Before you can make requests, you need to register an application with the LFS API. Next, choose the most appropriate LFS API OAuth2 flow for your project of those supported.
Your application can securely store a secret. The source code is hidden from the public.
Coming soon!
Your application cannot securely store a secret because all source code is public domain.
You can use your client_id
and client_secret
to make LFS API calls in your app:
// Import the LFSAPI module
import LFSAPI from "lfs-api";
// Keep your client ID and secret out of version control!
import { CLIENT_ID, CLIENT_SECRET } from "./secrets";
// Create an api class instance
const api = new LFSAPI(CLIENT_ID, CLIENT_SECRET);
// Make queries
// 1) With request API methods (recommended)
const modList = await api.getVehicleMods();
// 2) Based on endpoint url
const myMod = await api.makeRequest("vehiclemod/39CEEB");
This flow allows you to authenticate a user with their LFS account.
client_id
, scope
, redirect_uri
and an optional CSRF token (state
). The redirect_uri
is set in your lfs.net API settings (can be localhost for development and testing).redirect_uri
.Here's a short example:
// Import the LFSAPI module
import LFSAPI from "lfs-api";
// Optional: Choose a suitable library for your CSRF token E.g. uuid
import { v4 as uuidv4 } from "uuid";
// Keep your client ID and secret out of version control!
import { CLIENT_ID, CLIENT_SECRET } from "./secrets";
// Create an api class instance with your client ID, secret and redirect uri
const api = new LFSAPI(
CLIENT_ID,
CLIENT_SECRET,
"http://localhost:3100/api/v1/lfs"
);
// Generate an auth URL for users to authenticate with lfs.net
// Include scopes and optional CSRF token arguments
const csrf = uuidv4();
const { authURL } = api.generateAuthFlowURL("openid email profile", csrf);
// Or let lfs-api generate a CSRF Token for you:
const { authURL, csrfToken } = api.generateAuthFlowURL("openid email profile");
// Once the user visited your auth url...
// ...has accepted scopes at lfs.net...
// ...has been returned to your redirect uri...
// ...you will need to generate a set of tokens.
// Use the authorization code from the response URL to generate tokens
const authFlowTokens = await api.getAuthFlowTokens(req.query.code);
// This example uses ExpressJS to access the query ^^^^^^^^^^^^^^
// You can then store these tokens in a database and set cookies.
// This is left as an exercise for the reader
// You can now access the API by sending your token along with each request
const user = await api.getUserInfo(authFlowTokens.access_token);
const mod = await api.getVehicleMod("39CEEB", authFlowTokens.access_token);
// The LFSAPI.getAuthFlowTokens() method also receives a refresh token and an expiry in seconds.
// The latter is useful for checking ahead of time whether an access token has expired.
// You can use the refresh token to get a new set of tokens:
const newTokens = await api.refreshAccessToken(authFlowTokens.refresh_token);
// You would normally retrieve this refresh token from your database rather than using it directly.
This package does not yet support authorization flow with PKCE.
LFSAPI.constructor(client_id, client_secret, [redirect_url])
Create an LFS API class instance.
Parameter | Type | Description |
---|---|---|
client_id | string | Your application's client ID |
client_secret | string | Your application's client secret |
redirect_uri | string | Your application's redirect URI (Use only with Auth Flow) |
// Import the LFSAPI module
import LFSAPI from "lfs-api";
// Keep your client ID and secret out of version control!
import { CLIENT_ID, CLIENT_SECRET } from "./secrets";
// Create an api class instance for Client Credentials flow
const api = new LFSAPI(CLIENT_ID, CLIENT_SECRET);
// Create an api class instance for Authorization flow
const authApi = new LFSAPI(
CLIENT_ID,
CLIENT_SECRET,
"https://localhost:3100/api/v1/lfs"
);
async
LFSAPI.makeRequest(endpoint, [token])
Make an LFS API request based on the full API endpoint string. It is recommended to use one of the API request methods listed below.
Parameter | Type | Description |
---|---|---|
endpoint | string | An LFS API endpoint |
token | string | Access token (Use only with Auth Flow) |
API response as JSON object (see below for examples)
LFSAPI.generateAuthFlowURL(scope, [state])
Generate a URL for authorization flow to direct users to lfs.net for authentication. You can include a CSRF token and the scopes below.
Parameter | Type | Description |
---|---|---|
scope | string | A space delimited string of scopes (see table below) |
state | string | A CSRF Token (Optional). If not specified, one will be generated for you |
Scope | Type | Description |
---|---|---|
openid | Required | Gives just the user ID |
profile | Optional | Adds the username and last profile update date |
email | Optional | Adds the email address and whether it's verified |
// Choose a suitable library for your CSRF token E.g. uuid
import { v4 as uuidv4 } from "uuid";
// Generate an auth URL for users to authenticate with lfs.net
// Include scopes and optional CSRF token arguments
const csrf = uuidv4();
const { authURL } = api.generateAuthFlowURL("openid email profile", csrf);
// Or let lfs-api generate a CSRF Token for you:
const { authURL, csrfToken } = api.generateAuthFlowURL("openid email profile");
{
authURL: "https://id.lfs.net/oauth2/authorize?response_type=code&client_id=YOUR_CLIENT_ID&redirect_uri=http%3A%2F%2Flocalhost%3A3100%2Fapi%2Fv1%2Flfs&scope=openid+profile&state=11bf5b37-e0b8-42e0-8dcf-dc8c4aefc000",
csrfToken: "11bf5b37-e0b8-42e0-8dcf-dc8c4aefc000"
}
LFSAPI.getAuthFlowTokens(code)
Use authorization flow code returned from lfs.net during authentication in exchange for access and refresh tokens. Use only with auth flow.
Parameter | Type | Description |
---|---|---|
code | string | The code query string parameter added by lfs.net when redirecting back to your site |
LFSAPI.refreshAccessToken(refresh_token)
Returns a new access and refresh token. You should use the expires_in
property from LFSAPI.getAccessTokens()
to check whether an access token has expired before calling this function as this invalidates the previous access token and destroys a user session.
Parameter | Type | Description |
---|---|---|
refresh_token | string | A refresh_token previously received from LFSAPI.getAccessTokens() to refresh a user session. |
When using authorization flows, all API methods should be passed an access token as the last argument.
This module currently offers the following LFS API methods:
async
LFSAPI.getHosts([token])
List all hosts that you are an admin of.
async
LFSAPI.getHost(id, [token])
Get specific host you are an admin of by ID: id
string - Host ID
static
hostStatusesstatic
hostLocationsLFSAPI.lookupHostStatus(status)
Lookup the status
property index fetched from the hosts API. A list of host statuses is available via the LFSAPI.hostStatuses
static property.
LFSAPI.lookupHostLocation(location)
Lookup the location
property index fetched from the hosts API. A list of host locations is available via the LFSAPI.hostLocations
static property.
async
LFSAPI.getVehicleMods([token])
List all vehicle mods
async
LFSAPI.getVehicleMod(id, [token])
Get specific vehicle mod by ID: id
string - Vehicle mod ID
static
vehicleClassTypesstatic
vehicleICELayoutTypesstatic
vehicleDriveTypesstatic
vehicleShiftTypesLFSAPI.lookupVehicleClassType(id)
Lookup the class
property index fetched from the vehicle API. A list of vehicle class types is available via the LFSAPI.vehicleClassTypes
static property.
LFSAPI.lookupVehicleICELayoutType(id)
Lookup the iceLayout
property index fetched from the vehicle API. A list of ICE layout types is available via the LFSAPI.vehicleClassTypes
static property.
LFSAPI.lookupVehicleDriveType(id)
Lookup the drive
property index fetched from the vehicle API. A list of drive types is available via the LFSAPI.vehicleDriveTypes
static property.
LFSAPI.lookupVehicleShiftType(id)
Lookup the shiftType
property index fetched from the vehicle API. A list of shift types is available via the LFSAPI.vehicleShiftTypes
static property.
Use helper methods to present vehicle properties.
// Request a vehicle mod details
const { data: FZ5V8SafetyCar } = await api.getVehicleMod("39CEEB");
// Destructure some properties
const { class: vehicleClass } = FZ5V8SafetyCar;
const { iceLayout, drive, shiftType } = FZ5V8SafetyCar.vehicle;
// Use helper methods...
const result = {
class: api.lookupVehicleClassType(vehicleClass),
iceLayout: api.lookupVehicleICELayoutType(iceLayout),
drive: api.lookupVehicleDriveType(drive),
shiftType: api.lookupVehicleShiftType(shiftType),
};
{
"class": "Touring car",
"iceLayout": "V",
"drive": "Rear wheel drive",
"shiftType": "H-pattern gearbox"
}
async
LFSAPI.getUserInfo(token)
AUTHORIZATION FLOW ONLY - Required scopes: openid
Get information about the user you are authenticating
LFSAPI.setVerbose(verbose)
Toggle debug logging
verbose
boolean - Verbose debug messages
FAQs
Query the Live for Speed OAuth2 API in your Web projects
The npm package lfs-api receives a total of 0 weekly downloads. As such, lfs-api popularity was classified as not popular.
We found that lfs-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.