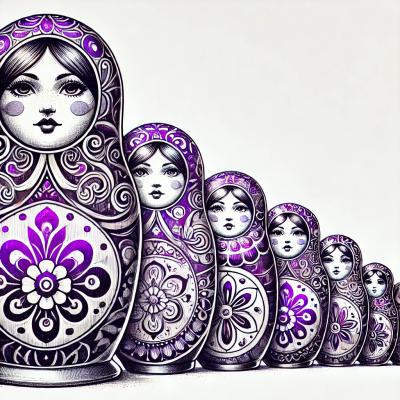
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
libnpmaccess
Advanced tools
The libnpmaccess package is a Node.js library that provides programmatic access to the npm access control features. It allows you to manage package access permissions, such as granting or revoking access to npm packages for different users or teams.
Grant Access
This feature allows you to grant access to a specific npm package for a user or team. In the code sample, 'read-write' access is granted to 'my-package' for the user 'username'.
const access = require('libnpmaccess');
async function grantAccess() {
await access.grant('read-write', 'my-package', 'user:username');
console.log('Access granted');
}
grantAccess();
Revoke Access
This feature allows you to revoke access to a specific npm package for a user or team. In the code sample, access to 'my-package' is revoked for the user 'username'.
const access = require('libnpmaccess');
async function revokeAccess() {
await access.revoke('my-package', 'user:username');
console.log('Access revoked');
}
revokeAccess();
List Access
This feature allows you to list the access permissions for a specific npm package. In the code sample, the access permissions for 'my-package' are listed.
const access = require('libnpmaccess');
async function listAccess() {
const accessList = await access.lsPackages('my-package');
console.log(accessList);
}
listAccess();
The npm package itself provides CLI commands for managing access control, such as `npm access grant` and `npm access revoke`. While libnpmaccess offers programmatic access, the npm package is more suited for command-line operations.
The np package is a streamlined tool for publishing npm packages. It includes some access control features but is primarily focused on simplifying the publishing process. It does not offer the same level of granularity in access control as libnpmaccess.
The npm-cli-login package is used for programmatically logging into npm. While it does not manage access control, it is often used in conjunction with other tools that do. It complements libnpmaccess by handling authentication.
libnpmaccess
is a Node.js
library that provides programmatic access to the guts of the npm CLI's npm access
command and its various subcommands. This includes managing account 2FA,
listing packages and permissions, looking at package collaborators, and defining
package permissions for users, orgs, and teams.
const access = require('libnpmaccess')
// List all packages @zkat has access to on the npm registry.
console.log(Object.keys(await access.lsPackages('zkat')))
$ npm install libnpmaccess
opts
for libnpmaccess
commandslibnpmaccess
uses npm-registry-fetch
.
All options are passed through directly to that library, so please refer to its
own opts
documentation
for options that can be passed in.
A couple of options of note for those in a hurry:
opts.token
- can be passed in and will be used as the authentication token for the registry. For other ways to pass in auth details, see the n-r-f docs.opts.otp
- certain operations will require an OTP token to be passed in. If a libnpmaccess
command fails with err.code === EOTP
, please retry the request with {otp: <2fa token>}
> access.public(spec, [opts]) -> Promise<Boolean>
spec
must be an npm-package-arg
-compatible
registry spec.
Makes package described by spec
public.
await access.public('@foo/bar', {token: 'myregistrytoken'})
// `@foo/bar` is now public
> access.restricted(spec, [opts]) -> Promise<Boolean>
spec
must be an npm-package-arg
-compatible
registry spec.
Makes package described by spec
private/restricted.
await access.restricted('@foo/bar', {token: 'myregistrytoken'})
// `@foo/bar` is now private
> access.grant(spec, team, permissions, [opts]) -> Promise<Boolean>
spec
must be an npm-package-arg
-compatible
registry spec. team
must be a fully-qualified team name, in the scope:team
format, with or without the @
prefix, and the team must be a valid team within
that scope. permissions
must be one of 'read-only'
or 'read-write'
.
Grants read-only
or read-write
permissions for a certain package to a team.
await access.grant('@foo/bar', '@foo:myteam', 'read-write', {
token: 'myregistrytoken'
})
// `@foo/bar` is now read/write enabled for the @foo:myteam team.
> access.revoke(spec, team, [opts]) -> Promise<Boolean>
spec
must be an npm-package-arg
-compatible
registry spec. team
must be a fully-qualified team name, in the scope:team
format, with or without the @
prefix, and the team must be a valid team within
that scope. permissions
must be one of 'read-only'
or 'read-write'
.
Removes access to a package from a certain team.
await access.revoke('@foo/bar', '@foo:myteam', {
token: 'myregistrytoken'
})
// @foo:myteam can no longer access `@foo/bar`
> access.tfaRequired(spec, [opts]) -> Promise<Boolean>
spec
must be an npm-package-arg
-compatible
registry spec.
Makes it so publishing or managing a package requires using 2FA tokens to complete operations.
await access.tfaRequires('lodash', {token: 'myregistrytoken'})
// Publishing or changing dist-tags on `lodash` now require OTP to be enabled.
> access.tfaNotRequired(spec, [opts]) -> Promise<Boolean>
spec
must be an npm-package-arg
-compatible
registry spec.
Disabled the package-level 2FA requirement for spec
. Note that you will need
to pass in an otp
token in opts
in order to complete this operation.
await access.tfaNotRequired('lodash', {otp: '123654', token: 'myregistrytoken'})
// Publishing or editing dist-tags on `lodash` no longer requires OTP to be
// enabled.
> access.lsPackages(entity, [opts]) -> Promise<Object | null>
entity
must be either a valid org or user name, or a fully-qualified team name
in the scope:team
format, with or without the @
prefix.
Lists out packages a user, org, or team has access to, with corresponding permissions. Packages that the access token does not have access to won't be listed.
In order to disambiguate between users and orgs, two requests may end up being made when listing orgs or users.
For a streamed version of these results, see
access.lsPackages.stream()
.
await access.lsPackages('zkat', {
token: 'myregistrytoken'
})
// Lists all packages `@zkat` has access to on the registry, and the
// corresponding permissions.
> access.lsPackages.stream(scope, [team], [opts]) -> Stream
entity
must be either a valid org or user name, or a fully-qualified team name
in the scope:team
format, with or without the @
prefix.
Streams out packages a user, org, or team has access to, with corresponding
permissions, with each stream entry being formatted like [packageName, permissions]
. Packages that the access token does not have access to won't be
listed.
In order to disambiguate between users and orgs, two requests may end up being made when listing orgs or users.
The returned stream is a valid asyncIterator
.
for await (let [pkg, perm] of access.lsPackages.stream('zkat')) {
console.log('zkat has', perm, 'access to', pkg)
}
// zkat has read-write access to eggplant
// zkat has read-only access to @npmcorp/secret
> access.lsCollaborators(spec, [user], [opts]) -> Promise<Object | null>
spec
must be an npm-package-arg
-compatible
registry spec. user
must be a valid user name, with or without the @
prefix.
Lists out access privileges for a certain package. Will only show permissions
for packages to which you have at least read access. If user
is passed in, the
list is filtered only to teams that user happens to belong to.
For a streamed version of these results, see access.lsCollaborators.stream()
.
await access.lsCollaborators('@npm/foo', 'zkat', {
token: 'myregistrytoken'
})
// Lists all teams with access to @npm/foo that @zkat belongs to.
> access.lsCollaborators.stream(spec, [user], [opts]) -> Stream
spec
must be an npm-package-arg
-compatible
registry spec. user
must be a valid user name, with or without the @
prefix.
Stream out access privileges for a certain package, with each entry in [user, permissions]
format. Will only show permissions for packages to which you have
at least read access. If user
is passed in, the list is filtered only to teams
that user happens to belong to.
The returned stream is a valid asyncIterator
.
for await (let [usr, perm] of access.lsCollaborators.stream('npm')) {
console.log(usr, 'has', perm, 'access to npm')
}
// zkat has read-write access to npm
// iarna has read-write access to npm
FAQs
programmatic library for `npm access` commands
The npm package libnpmaccess receives a total of 219,257 weekly downloads. As such, libnpmaccess popularity was classified as popular.
We found that libnpmaccess demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.