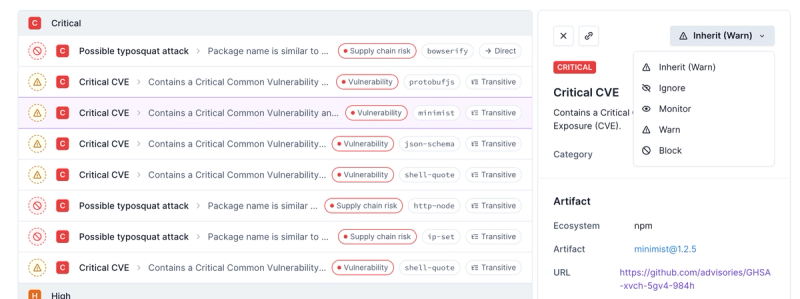
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
libsodium-wrappers
Advanced tools
Readme
The sodium crypto library compiled to pure JavaScript using Emscripten, with automatically generated wrappers to make it easy to use in web applications.
The complete library weights 115 Kb (minified, gzipped) and can run in a web browser as well as server-side.
Supported browsers/JS engines:
Ready-to-use files based on libsodium 1.0.13 can be directly copied to your project.
Use Bower:
$ bower install libsodium.js
or directly include a copy of the sodium.min.js file.
Alternatively, for better performance and to avoid including a local copy, libsodium.js is available on cdnjs.
Including the sodium.min.js
file will add a sodium
object to the
global namespace.
If a sodium
object is already present in the global namespace, and
the sodium.onload
function is defined, this function will be called
right after the library has been loaded and initialized.
<script>
window.sodium = { onload: function(sodium) {
alert(sodium.to_hex(sodium.crypto_generichash(64, 'test')));
}};
</script>
...
<script src="sodium.js" async defer></script>
As an alternative, use a module loader or Browserify as described below.
Copy the .js
files for libsodium and libsodium-wrappers
to your project and load the libsodium-wrappers
module.
Alternatively, use yarn. The Yarn package is
called libsodium-wrappers
and includes a dependency on the raw
libsodium
module.
$ yarn add libsodium-wrappers
var sodium = require('libsodium-wrappers');
console.log(sodium.to_hex(sodium.crypto_generichash(64, 'test')));
crypto_aead
(XChaCha20-Poly1305)crypto_auth
(HMAC-SHA-512-256)crypto_box
(X25519, XSalsa20)crypto_box_seal
(X25519, XSalsa20)crypto_generichash
(BLAKE2b)crypto_hash
(SHA-512-256)crypto_kdf
(BLAKE2b)crypto_kx
(X25519, BLAKE2b)crypto_onetimeauth
(Poly1305)crypto_pwhash
(Argon2, Scrypt)crypto_scalarmult
(X25519)crypto_secretbox
(Salsa20-Poly1305)crypto_shorthash
(SipHash, SipHash-128)crypto_sign
(Ed25519, Ed25519ph)crypto_stream
(Salsa20, XSalsa20, ChaCha20, XChaCha20)randombytes
from_hex()
, to_hex()
from_string()
, to_string()
memcmp()
(constant-time check for equality, returns true
or false
)compare() (constant-time comparison. Values must have the same size. Returns
-1,
0or
1`)memzero()
(applies to Uint8Array
objects)increment()
(increments an arbitrary-long number stored as a
little-endian Uint8Array
- typically to increment nonces)add()
(adds two arbitrary-long numbers stored as little-endian
Uint8Array
vectors)is_zero()
(constant-time, checks Uint8Array
objects for all zeros)The API exposed by the wrappers is identical to the one of the C library, except that buffer lengths never need to be explicitly given.
Binary input buffers should be Uint8Array
objects. However, if a string
is given instead, the wrappers will automatically convert the string
to an array containing a UTF-8 representation of the string.
Example:
var key = sodium.randombytes_buf(sodium.crypto_shorthash_KEYBYTES),
hash1 = sodium.crypto_shorthash(new Uint8Array([1, 2, 3, 4]), key),
hash2 = sodium.crypto_shorthash('test', key);
If the output is a unique binary buffer, it is returned as a
Uint8Array
object.
However, an extra parameter can be given to all wrapped functions, in order to specify what format the output should be in. Valid options are `uint8array' (default), 'text' and 'hex'.
Example (shorthash):
var key = sodium.randombytes_buf(sodium.crypto_shorthash_KEYBYTES),
hash_hex = sodium.crypto_shorthash('test', key, 'hex');
Example (secretbox):
// Load your secret key from a safe place and reuse it across multiple
// secretbox calls. (Obviously don't use this example key for anything
// real.)
//
var secret = Buffer.from('724b092810ec86d7e35c9d067702b31ef90bc43a7b598626749914d6a3e033ed', 'hex');
// Given a message as a string, return a Buffer containing the
// nonce (in the first 24 bytes) and the encrypted content.
var encrypt = function(message) {
// You must use a different nonce for each message you encrypt.
var nonce = Buffer.from(sodium.randombytes_buf(sodium.crypto_box_NONCEBYTES));
var buf = Buffer.from(message);
return Buffer.concat([nonce, Buffer.from(sodium.crypto_secretbox_easy(buf, nonce, secret))]);
},
// Decrypt takes a Buffer and returns the decrypted message as plain text.
var decrypt = function(encryptedBuffer) {
var nonce = encryptedBuffer.slice(0, sodium.crypto_box_NONCEBYTES);
var encryptedMessage = encryptedBuffer.slice(sodium.crypto_box_NONCEBYTES);
return sodium.crypto_secretbox_open_easy(encryptedMessage, nonce, secret, 'text');
}
In addition, the from_hex
, to_hex
, from_string
, and to_string
functions are available to explicitly convert hexadecimal, and
arbitrary string representations from/to Uint8Array
objects.
Functions returning more than one output buffer are returning them as
an object. For example, the sodium.crypto_box_keypair()
function
returns the following object:
{ keyType: 'curve25519', privateKey: (Uint8Array), publicKey: (Uint8Array) }
The standard version (in the dist/browsers
and dist/modules
directories) contains the high-level functions, and is the recommended
one for most projects.
Alternatively, the "sumo" version, available in the
dist/browsers-sumo
and dist/modules-sumo
directories contains all
the symbols from the original library. This includes undocumented,
untested, deprecated, low-level and easy to misuse functions.
The crypto_pwhash_*
function set is also only included in the Sumo
version. The high amount of heap memory (allocated after loading)
required by these functions may not be desirable when they are not
being used.
The sumo version is slightly larger than the standard version, and should be used only if you really need the extra symbols it provides.
If you want to compile the files yourself, the following dependencies need to be installed on your system:
yarn global add node-zopfli
)yarn global add uglify-es
)Running make
will clone libsodium, build it, test it, build the
wrapper, and create the modules and minified distribution files.
The build available in this repository does not contain all the functions available in the original libsodium library.
Providing that you have all the build dependencies installed, here is how you can build libsodium.js to include the functions you need :
git clone https://github.com/jedisct1/libsodium.js
cd libsodium.js
# Get the original C version of libsodium and configure it
make libsodium/configure
# Modify the emscripten.sh
# Specifically, add the name of the missing functions and constants in the "EXPORTED_FUNCTIONS" array.
# Ensure that the name begins with an underscore and that it is between double quotes.
nano libsodium/dist-build/emscripten.sh
# Build libsodium, and then libsodium.js with your chosen functions
make
NOTE: for each of the functions/constants you add, make sure that
the corresponding symbol files exist in the wrapper/symbols
folder
and that the constants are listed in the wrapper/constants.json
file.
Built by Ahmad Ben Mrad and Frank Denis.
This wrapper is distributed under the ISC License.
FAQs
The Sodium cryptographic library compiled to pure JavaScript (wrappers)
The npm package libsodium-wrappers receives a total of 498,916 weekly downloads. As such, libsodium-wrappers popularity was classified as popular.
We found that libsodium-wrappers demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.