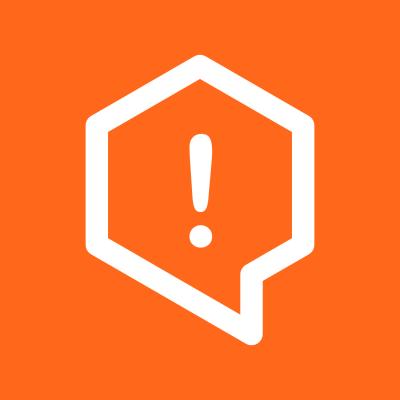
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
lifx-http-api
Advanced tools
A thin Node.js API wrapper of the Lifx HTTP protocol.
This library is not, in any way, affiliated or related to LiFi Labs, Inc.. Use at your own risk.
$ npm install http-lifx-api --save
Node.js 4.2.6+ is tested and supported on Mac, Linux and Windows.
A bearer token is mandatory to use the API. A new token can be obtain in the Lifx Cloud Settings.
The thin API wrapper uses a client for network communication. This client handles all requests against the Lifx API.
var lifx = require('lifx-http-api'),
client;
client = new lifx({
bearerToken: '<your api token>'
});
The Client
object provides promises by the great Q library. You can either use callbacks or promises.
// Using callbacks
client.listLights('all', function(err, data) {
if(err) {
console.error(err);
return;
}
console.log(data)
});
// Using promises
client.listLights('all').then(console.log, console.error);
client.listLights(selector, [cb])
Gets lights belonging to the authenticated account. Filter the lights using selectors.
Option | Type | Default | Description |
---|---|---|---|
selector | string | Selector for the light bulb you want to get. See the Selector section to get more information. | |
cb | function | null | function(err, data) {} Callback function which will be called when the HTTP request to the API was processed. |
Usage example:
// Using callbacks
client.listLights('all', function(err, data) {
if(err) {
console.error(err);
return;
}
console.log(data)
});
// Using promises
client.listLights('all').then(console.log, console.error);
client.listScenes([cb])
Lists all the scenes available in the users account.
Option | Type | Default | Description |
---|---|---|---|
cb | function | null | function(err, data) {} Callback function which will be called when the HTTP request to the API was processed. |
Usage example:
// Using callbacks
client.listScene(function(err, data) {
if(err) {
console.error(err);
return;
}
console.log(data)
});
// Using promises
client.listScenes().then(console.log, console.error);
client.setState(selector, settings, [cb])
Sets the state of the lights within the selector.
Option | Type | Default | Description |
---|---|---|---|
selector | string | Selector for the light bulb you want to get. See the Selector section to get more information. | |
settings | object | {} | State configuration object. See the official documentation for further information. |
cb | function | null | function(err, data) {} Callback function which will be called when the HTTP request to the API was processed. |
Usage example:
// Using callbacks
client.setState('all', {
power: 'on',
color: 'blue saturation:0.5',
brightness: 0.5,
duration: 5
}, function(err, data) {
if(err) {
console.error(err);
return;
}
console.log(data)
});
// Using promises
client.setState('all', {
power: 'on',
color: 'blue saturation:0.5',
brightness: 0.5,
duration: 5
}).then(console.log, console.error);
client.setStates(settings, [cb])
This endpoint allows you to set different states on multiple selectors in a single request.
Option | Type | Default | Description |
---|---|---|---|
settings | Mixed | {} | Multiple State configuration object. See the official documentation for further information. |
cb | function | null | function(err, data) {} Callback function which will be called when the HTTP request to the API was processed. |
Usage example:
// Using callbacks
client.setState('all', {
"states": [ {
"selector": "all",
"power": "on"
}, {
"selector": "group:test",
"brightness": 0.5
} ],
"defaults": {
"duration": 5.0
}
}, function (err, data) {
if (err) {
console.error(err);
return;
}
console.log(data)
});
client.togglePower(selector, [duration], [cb])
Turn off lights if they are on, or turn them on if they are off. Physically powered off lights are ignored.
Option | Type | Default | Description |
---|---|---|---|
selector | string | all | Selector for the light bulb you want to get. See the Selector section to get more information. |
duration | int | 0 | Turning on or off will be faded over the time (in seconds). |
cb | function | null | function(err, data) {} Callback function which will be called when the HTTP request to the API was processed. |
Usage example:
// Using callbacks
client.togglePower('all', 1.5, function (err, data) {
if (err) {
console.error(err);
return;
}
console.log(data)
});
client.breathe(selector, [settings], [cb])
Performs a breathe effect by slowly fading between the given colors. Use the parameters to tweak the effect.
Option | Type | Default | Description |
---|---|---|---|
selector | string | all | Selector for the light bulb you want to get. See the Selector section to get more information. |
settings | Object | {} | Breathe effect object, see the official documentation for all available parameter. |
cb | function | null | function(err, data) {} Callback function which will be called when the HTTP request to the API was processed. |
Usage example:
// Using callbacks
client.breathe('all', {
color: '#006633',
from_color: '#00AF33',
period: 1,
cycles: 10,
persist: true,
power_on: true,
peak: 0.8
}, function (err, data) {
if (err) {
console.error(err);
return;
}
console.log(data)
});
client.pulse(selector, [settings], [cb])
Performs a pulse effect by quickly flashing between the given colors. Use the parameters to tweak the effect.
Option | Type | Default | Description |
---|---|---|---|
selector | string | all | Selector for the light bulb you want to get. See the Selector section to get more information. |
settings | Object | {} | Pulse effect object, see the official documentation for all available parameter. |
cb | function | null | function(err, data) {} Callback function which will be called when the HTTP request to the API was processed. |
Usage example:
// Using callbacks
client.pulse('all', {
color: '#006633',
from_color: '#00AF33',
period: 1,
cycles: 10,
persist: true,
power_on: true,
peak: 0.8
}, function (err, data) {
if (err) {
console.error(err);
return;
}
console.log(data)
});
client.cycle(selector, [settings], [cb])
Make the light(s) cycle to the next or previous state in a list of states.
Option | Type | Default | Description |
---|---|---|---|
selector | string | all | Selector for the light bulb you want to get. See the Selector section to get more information. |
settings | Object | {} | Cycle states object, see the official documentation for all available parameter. |
cb | function | null | function(err, data) {} Callback function which will be called when the HTTP request to the API was processed. |
Usage example:
// Using callbacks
client.cycle('all', {
"states": [{
"brightness": 1.0
}, {
"brightness": 0.5
}, {
"brightness": 0.1
}, {
"power": "off"
}],
"defaults": {
"power": "on", // all states default to on
"saturation": 0, // every state is white
"duration": 2.0 // all transitions will be applied over 2 seconds
}
}, function (err, data) {
if (err) {
console.error(err);
return;
}
console.log(data)
});
client.activateScene(selector, [duration], [cb])
Activates a scene from the users account.
Option | Type | Default | Description |
---|---|---|---|
selector | string | all | Scene selector. See the Selector section to get more information. |
duration | int | 0 | Fades to the scene (in seconds). |
cb | function | null | function(err, data) {} Callback function which will be called when the HTTP request to the API was processed. |
Usage example:
// Using callbacks
client.activateScene('scene_id:d073d501cf2c', 1.2, function (err, data) {
if (err) {
console.error(err);
return;
}
console.log(data)
});
client.validateColor(color, [cb])
This method lets you validate a user's color string and return the hue, saturation, brightness and kelvin values that the API will interpret as.
Option | Type | Default | Description |
---|---|---|---|
color | string | Color string you'd like to validate. See the Color section to get more information. | |
cb | function | null | function(err, data) {} Callback function which will be called when the HTTP request to the API was processed. |
Usage example:
// Using callbacks
client.validateColor('#0198E1', function (err, data) {
if (err) {
console.error(err);
return;
}
console.log(data)
});
The Client
object can be configured at initialization:
var lifx = require('lifx-http-api'),
client;
client = new lifx({
bearerToken: '<your api token>', // Authentication token
version: 'v2beta', // API version
url: 'https://api.lifx.com' // API endpoint
});
FAQs
Thin wrapper around the Lifx HTTP API
The npm package lifx-http-api receives a total of 30 weekly downloads. As such, lifx-http-api popularity was classified as not popular.
We found that lifx-http-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.