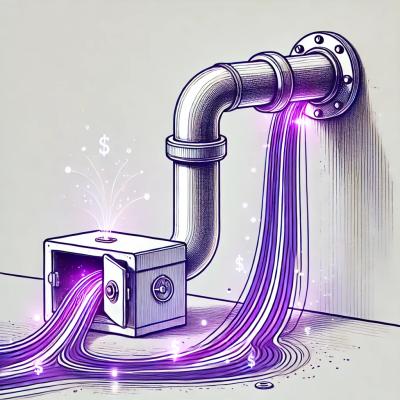
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
lightning-pool
Advanced tools
High performance resource pool for NodeJS.
npm install lightning-pool --save
const lightningPool = require('lightning-pool');
const dbDriver = require('some-db-driver');
/**
* Step 1 - Create a factory object
*/
const factory = {
create: function(callback){
DbDriver.createClient(callback);
},
destroy: function(client, callback){
client.disconnect(callback);
},
reset: function(client, callback){
client.rollback(callback);
},
validate: function(client, callback){
client.execute('some test sql', callback);
}
};
/**
* Step 1 - Create a the `Pool` object
*/
var opts = {
max: 10, // maximum size of the `Pool`
min: 2, // minimum size of the `Pool`
minIdle: 2 // minimum idle resources
}
var pool = lightningPool.createPool(factory, opts)
/**
* Step 3 - Use `Pool` in your code to acquire/release resources
*/
// acquire connection - Promise is resolved
// once a resource becomes available
pool.acquire(function(err, client) {
// handle error
if (err)
return console.error(err);
// Use resource
client.query("select * from foo", [], function() {
// return object back to pool
pool.release(client);
});
});
/**
* Step 3 - Shutdown pool (optional)
* Call stop(force, callback) when you need to shutdown the pool
*/
process.on('SIGINT', function() {
pool.stop(true);
});
const lightningPool = require('lightning-pool');
const dbDriver = require('some-db-driver');
/**
* Step 1 - Create a factory object
*/
const factory = {
create: function(){
return new Promise(function(resolve, reject) {
DbDriver.createClient(function(err, client) {
if (err)
return reject(err);
resolve(client);
});
});
},
destroy: function(client){
return new Promise(function(resolve, reject) {
client.destroy(function(err) {
if (err)
return reject(err);
resolve();
});
});
},
reset: function(client){
return new Promise(function(resolve, reject) {
client.rollback(function(err) {
if (err)
return reject(err);
resolve();
});
});
},
validate: function(client){
return new Promise(function(resolve, reject) {
client.execute('some test sql', function(err) {
if (err)
return reject(err);
resolve();
});
});
}
};
/**
* Step 1 - Create a the pool object
*/
var opts = {
max: 10, // maximum size of the pool
min: 2, // minimum size of the pool
minIdle: 2 // minimum idle resources
}
var pool = lightningPool.createPool(factory, opts)
/**
* Step 3 - Use pool in your code to acquire/release resources
*/
// acquire connection - Promise is resolved
// once a resource becomes available
pool.acquire().then(client => {
// Use resource
client.query("select * from foo", [], function() {
// return object back to pool
pool.release(client);
});
}).catch(err => {
console.error(err);
});
/**
* Step 3 - Shutdown pool (optional)
* Call stop(force, callback) when you need to shutdown the pool
*/
process.on('SIGINT', function() {
pool.stop(true);
});
Pool
instancelightning-pool module exports createPool() method and Pool class. Both can be used to instantiate a Pool.
const lightningPool = require('lightning-pool');
const pool = lightningPool.createPool(factory, options);
const {Pool} = require('lightning-pool');
const pool = new Pool(factory, options);
Can be any object/instance with the following properties:
create
: The function that the Pool
will call when it needs a new resource. It can return a Promise
or callback
argument can be used to when create process done.destroy
: The function that the Pool
will call when it wants to destroy a resource
. It should accept first argument as resource
, where resource
is whatever factory.create made. It can return a Promise
or callback
argument can be used to when destroy process done.reset
(optional) : The function that the Pool
will call before any resource
back to the Pool
. It should accept first argument as resource
, where resource
is whatever factory.create made. It can return a Promise
or callback
argument can be used to when reset process done. Pool
will destroy and remove the resource from the Pool
on any error.validate
(optional) : The function that the Pool
will call when any resource needs to be validated. It should accept first argument as resource
, where resource
is whatever factory.create made. It can return a Promise
or callback
argument can be used to when reset process done. Pool
will destroy and remove the resource from the Pool
on any error.acquireMaxRetries
: Maximum number that Pool
will try to create a resource before returning the error. (Default 0)acquireRetryWait
: Time in millis that Pool
will wait after each tries. (Default 2000)acquireTimeoutMillis
: Time in millis an acquire call will wait for a resource before timing out. (Default 0 - no limit)fifo
: If true resources will be allocated first-in-first-out order. resources will be allocated last-in-first-out order. (Default true)idleTimeoutMillis
: The minimum amount of time in millis that an resource
may sit idle in the Pool
. (Default 30000)houseKeepInterval
: Time period in millis that Pool
will make a cleanup. (Default 1000)min
: Minimum number of resources that Pool
will keep. (Default 0)minIdle
: Minimum number of resources that Pool
will keep in idle state. (Default 0)max
: Maximum number of resources that Pool
will create. (Default 10)maxQueue
: Maximum number of request that Pool
will accept. (Default 1000)resetOnReturn
: If true Pool
will call reset()
function of factory before moving it idle state. (Default true)validation
: If true Pool
will call validation()
function of factory when it needs it. If false, validation()
never been called. (Default true)Acquires a resource
from the Pool
or create a new one.
pool.acquire(callback)
callback: function(error, resource)
: A function with two arguments.pool.acquire(function(error, resource) {
if (error)
return console.error(error);
// Do what ever you want with resource
});
pool.acquire()
var promise = pool.acquire();
promise.then(resource => {
// Do what ever you want with resource
}).catch(err =>{
// Handle Error
});
Returns if a resource has been acquired from the Pool
and not yet released or destroyed.
pool.isAcquired(resource)
resource
: A previously acquired resourceif (pool.isAcquired(resource)) {
// Do any thing
}
Returns if the Pool
contains a resource
pool.includes(resource)
resource
: A resource objectPool
, else Falseif (pool.includes(resource)) {
// Do any thing
}
Releases an allocated resource
and let it back to pool.
pool.release(resource)
resource
: A previously acquired resourcepool.release(resource);
Releases, destroys and removes any resource
from Pool
.
pool.destroy(resource)
resource
: A previously acquired resourcepool.destroy(resource);
Starts the Pool
and begins creating of resources, starts house keeping and any other internal logic.
Note: This method is not need to be called. Pool
instance will automatically be started when acquire() method is called
pool.start()
pool.start();
Shuts down the Pool
and destroys all resources.
pool.stop([force], callback)
force
(optional): If true, Pool
will immediately destroy resources instead of waiting to be released (Default false)callback
: A function with one argument.pool.stop(function(error) {
if (error)
return console.error(error);
console.log('Pool has been shut down')
});
pool.stop([force])
force
(optional): If true, Pool
will immediately destroy resources instead of waiting to be released (Default false)var promise = pool.stop();
promise.then(() => {
console.log('Pool has been shut down')
}).catch(err => {
console.error(err);
});
acquired
(Number): Returns number of acquired resources.available
(Number): Returns number of idle resources.creating
(Number): Returns number of resources currently been created.pending
(Number): Returns number of acquire request waits in the Pool
queue.size
(Number): Returns number of total resources.state
(PoolState): Returns current state of the Pool
.options
(PoolOptions): Returns object instance that holds configuration properties
acquireMaxRetries
(Get/Set): Maximum number that Pool
will try to create a resource before returning the error. (Default 0)acquireRetryWait
(Get/Set): Time in millis that Pool
will wait after each tries. (Default 2000)acquireTimeoutMillis
(Get/Set): Time in millis an acquire call will wait for a resource before timing out. (Default 0 - no limit)fifo
(Get/Set): If true resources will be allocated first-in-first-out order. resources will be allocated last-in-first-out order. (Default true)idleTimeoutMillis
(Get/Set): The minimum amount of time in millis that an resource
may sit idle in the Pool
. (Default 30000)houseKeepInterval
(Get/Set): Time period in millis that Pool
will make a cleanup. (Default 1000)min
(Get/Set): Minimum number of resources that Pool
will keep. (Default 0)minIdle
(Get/Set): Minimum number of resources that Pool
will keep in idle state. (Default 0)max
(Get/Set): Maximum number of resources that Pool
will create. (Default 10)maxQueue
(Get/Set): Maximum number of request that Pool
will acceps. (Default 1000)resetOnReturn
(Get/Set): If true Pool
will call reset()
function of factory before moving it idle state. (Default true)validation
(Get/Set): If true Pool
will call validation()
function of factory when it needs it. If false, validation()
never been called. (Default true)Pool derives from EventEmitter and produce the following events:
acquire
: Emitted when a resource acquired.pool.on('acquire', function(resource){
//....
})
create
: Emitted when a new resource is added to the Pool
.pool.on('create', function(resource){
//....
})
create-error
: Emitted when a factory.create informs any error.pool.on('create-error', function(error){
//Log stuff maybe
})
destroy
: Emitted when a resource is destroyed and removed from the Pool
.destroy-error
: Emitted when a factory.destroy informs any error.pool.on('destroy-error', function(error, resource){
//Log stuff maybe
})
return
: Emitted when an acquired resource returns to the Pool
.pool.on('start', function(resource){
//...
})
start
: Emitted when the Pool
started.pool.on('start', function(){
//...
})
stopping
: Emitted when before stopping the Pool
.pool.on('stopping', function(){
//...
})
stop
: Emitted when after stopping the Pool
.pool.on('stop', function(){
//...
})
validate-error
: Emitted when a factory.validate informs any error.pool.on('validate-error', function(error, resource){
//Log stuff maybe
})
Pool.PoolState (Number):
IDLE: 0, // Pool has not been started
STARTED: 1, // Pool has been started
STOPPING: 2, // Pool shutdown in progress
STOPPED: 3 // Pool has been shut down
>= 4.0
;FAQs
Fastest generic Pool written with TypeScript
The npm package lightning-pool receives a total of 33,080 weekly downloads. As such, lightning-pool popularity was classified as popular.
We found that lightning-pool demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.