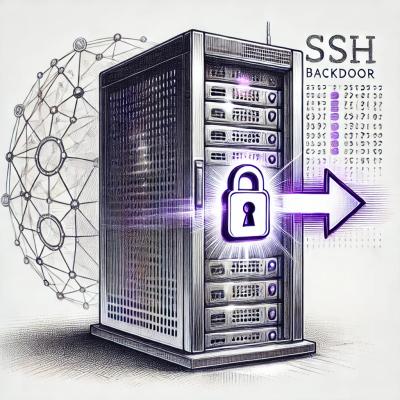
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
linkedin-js
Advanced tools
Easy peasy linkedin client for connect.
npm install linkedin-js
linkedin-js has two methods.
Params are sent as javascript objects and parsed to XML. Params must contain the token.
var express = require('express'),
connect = require('connect');
var linkedinClient = require('./../')(
'key',
'secret',
'http://localhost:3003/'
),
app = express.createServer(
connect.cookieDecoder(),
connect.session()
);
app.get('/', function (req, res) {
linkedinClient.getAccessToken(req, res, function (error, token) {
res.render('auth.jade');
});
});
app.post('/message', function (req, res) {
linkedinClient.apiCall('POST', '/people/~/shares',
{ token: { oauth_token_secret: req.param('oauth_token_secret'), oauth_token: req.param('oauth_token') },
share: {comment: req.param('message'), visibility: {code: 'anyone'}}},
function (error, result) {
res.render('message_sent.jade');
}
);
});
app.listen(3003);
FAQs
Minimalistic linkedin API client
We found that linkedin-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.