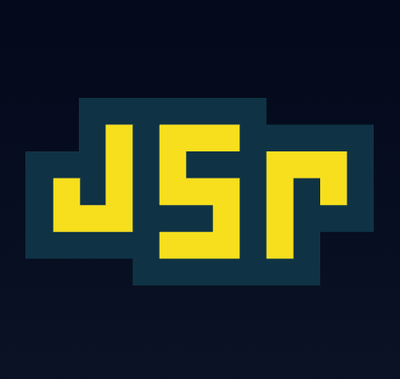
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The lit npm package is a simple and fast library for building web components and web applications. It allows developers to create reusable, encapsulated, and interactive elements using modern JavaScript and Web Component standards. Lit is designed to be lightweight and efficient, focusing on speed and minimal overhead.
Creating Web Components
This feature allows developers to create custom web components using the LitElement base class. The example demonstrates defining a new element with a simple style and rendering method.
import { LitElement, html, css } from 'lit';
class MyElement extends LitElement {
static styles = css`p { color: blue; }`;
render() {
return html`<p>Hello, world!</p>`;
}
}
customElements.define('my-element', MyElement);
Reactive Properties
This feature showcases Lit's reactive property system. The example includes a counter component that updates its display whenever the 'count' property changes.
import { LitElement, html, css, property } from 'lit';
class MyCounter extends LitElement {
@property({ type: Number }) count = 0;
render() {
return html`
<button @click=${this._increment}>Increment</button>
<span>${this.count}</span>
`;
}
_increment() {
this.count++;
}
}
customElements.define('my-counter', MyCounter);
Styling Components
This feature demonstrates how to apply CSS styles to a Lit component. The example shows defining styles for the component itself and its internal elements.
import { LitElement, html, css } from 'lit';
class StyledElement extends LitElement {
static styles = css`
:host {
display: block;
border: 1px solid black;
padding: 16px;
max-width: 200px;
}
.highlight {
color: red;
}
`;
render() {
return html`<div class='highlight'>Styled Content</div>`;
}
}
customElements.define('styled-element', StyledElement);
React is a popular library for building user interfaces. It focuses on a component-based architecture similar to Lit but uses a virtual DOM for updates, which differs from Lit's direct DOM manipulation approach.
Vue is a progressive framework for building UIs. Like Lit, it emphasizes component-based development and reactivity. However, Vue offers a more comprehensive framework experience with options for routing and state management.
Svelte is a compiler that generates efficient JavaScript code from component declarations. Unlike Lit, which runs in the browser, Svelte shifts much of the work to compile time, resulting in smaller bundles and faster runtime execution.
Lit is a simple library for building fast, lightweight web components.
At Lit's core is a boilerplate-killing component base class that provides reactive state, scoped styles, and a declarative template system that's tiny, fast and expressive.
See the full documentation for Lit at lit.dev
This is a stable release of Lit 2.0. If upgrading from previous versions of lit-element
or lit-html
, please see the Upgrade Guide for a step-by-step guide on upgrading.
Lit provides developers with just the right tools to build fast web components:
Lit builds on top of standard web components, and makes them easier to write:
import {LitElement, html, css} from 'lit';
import {customElement, property} from 'lit/decorators.js';
// Registers the element
@customElement('my-element')
export class MyElement extends LitElement {
// Styles are applied to the shadow root and scoped to this element
static styles = css`
span {
color: green;
}
`;
// Creates a reactive property that triggers rendering
@property()
mood = 'great';
// Render the component's DOM by returning a Lit template
render() {
return html`Web Components are <span>${this.mood}</span>!`;
}
}
Once you've defined your component, you can use it anywhere you use HTML:
<my-element mood="awesome"></my-element>
Please see CONTRIBUTING.md.
FAQs
A library for building fast, lightweight web components
The npm package lit receives a total of 1,730,573 weekly downloads. As such, lit popularity was classified as popular.
We found that lit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.