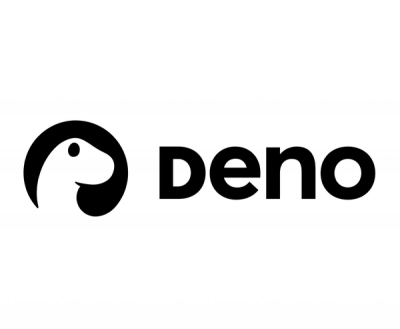
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@version 0.10.1
@date 2016-08-30
@stability 2 - Unstable
A small JavaScript framework for building single-page applications with declarative programming and less code.
Weighing in at just 25KB (11KB gzipped), it includes
No dependencies
See a working example with a source code in 60 lines.
It also supports SVG elements,
so it is possible to build full SVG single-page applications.
See a example
with a source code
in 60 lines.
It has a template engine with CSS selectors syntax for describing elements and attributes.
ul
li Item A
li Item B
becomes
<ul>
<li>Item A</li>
<li>Item B</li>
</ul>
a#123.link.bold[href="#A"][title=go] My link
button:disabled
becomes
<a id="123" class="link bold" href="#A" title="go">My link</a>
<button disabled="disabled"></button>
Child combinators can be used and you can leave off the tag to get a div.
.my-class
a>i text
becomes
<div class="my-class">
<a><i>text</i></a>
</div>
ul
@template row
li.my-row
a > b Row
row.first
row.last
becomes
<ul>
<li class="my-row first"><a><b>Row</b></a></li>
<li class="my-row last"><a><b>Row</b></a></li>
</ul>
Organize views into a hierarchy with built-in router.
View(name, element, parent)
String
- A name for a view that is also a route to the view.
Accepts Level 1 URI Templates RFC 6570String
or Function
or DOM Node
-
View representation as DOM tree.
String and Function will be transformed to DOM Node on first call.String
- Parent view name.// Optional path for views, default = ""
View.base = "/js/views/"
// Define starting point in DOM
View("body", document.body)
// Create first simple view
View("home", ".home", "body")
// Create another view
View("work", ".work", "body")
// Start
View.main = "home"
history.start(View.route)
// call manualy
View("home").show()
All code in any code-base should look like a single person typed it, no matter how many people contributed.
Arguments over style are pointless.
There should be a style guide, and you should follow it.
-- Rebecca Murphey
\n
,
and a newline character as the last character of a file.-
where you would normally use spaces.[
, {
or /
in the beginning of line.that
to refer to an outer this
.{
, after ;
in for loops, around binary operators 1 + 2
.i++
.switch
and its subordinate case
in the same level
instead of double-indenting the case
statements.lowerCamelCase
for method and variable names.UpperCamelCase
for constructor names.CAPITAL_SNAKE_CASE
for symbolic constants._
.var foo, bar
, MAX_AGE = 120
, baz = "baz"
, quux = 123
function Person(name, sex) {
var person = this
person.name = name
for (var i = 100; --i; ) {
person.sing(i + " bottles of beer on the wall")
}
switch (sex) {
case "male":
person.wear("bow tie")
break
case "female":
person.do("makeup")
break
default:
person
.be("confused")
.do("makeup")
}
;["healthy", "pretty", "wise"].map(person._setFlags, person)
}
Person.prototype._setFlags = function(flag) {
this[flag] = true
}
// Bad
var a = function() {}
// Good
function a() {}
{
and after :
.{
on the same line as last selector.}
on it's own line at same indentation level as selector.;
after last declaration.[<namespace>-]<ComponentName>[-descendentName][--modifierName]
.nav
not .navigation
./* icon.css */
.icon {
width: 16px;
height: 16px;
background-image: url(sprite.png);
box-shadow:
1px 1px 1px #000,
2px 2px 1px 1px #ccc inset;
-webkit-transition: all 4s ease;
-moz-transition: all 4s ease;
-ms-transition: all 4s ease;
-o-transition: all 4s ease;
transition: all 4s ease;
}
.icon--person { background-position: -16px 0 ; }
.icon--files { background-position: 0 -16px; }
.icon--settings { background-position: -16px -16px; }
Certain tags are used in comments to assist in indexing common issues:
There is a risk that tags accumulate over time; it is advisable to include the date and the tag owner in the comment to ease tracking.
// TODO:2008-12-06:johnc: Add support for negative offsets.
// While it is unlikely that we get a negative offset, it can
// occur if the garbage collector runs out of space.
// THANKS: Ralf Holly - TODO or not TODO [http://www.approxion.com/?p=39]
Please clean up logs when they are no longer helpful. In particular, logging the same object over and over again is not helpful. Logs should report what's happening so that it's easier to track down where a fault occurs.
git config --global user.name 'Real Name'
git config --global user.email <me>@<email>
# To prevent accidental pushes to branches which you’re not ready to push yet
git config --global push.default tracking
# Do not conflict with compiled files specified in .gitattributes
git config --global merge.ours.driver true
# setup rebase for every tracking branch
git config --global branch.autosetuprebase always
# Store passwords permanently in plaintext to ~/.git-credentials file
git config --global credential.helper store
# ... or cache for an hour
git config --global credential.helper 'cache --timeout=3600'
Copyright (c) 2013-2016 Lauri Rooden <lauri@rooden.ee>
The MIT License
FAQs
Full-stack web framework in a tiny package
The npm package litejs receives a total of 321 weekly downloads. As such, litejs popularity was classified as not popular.
We found that litejs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.