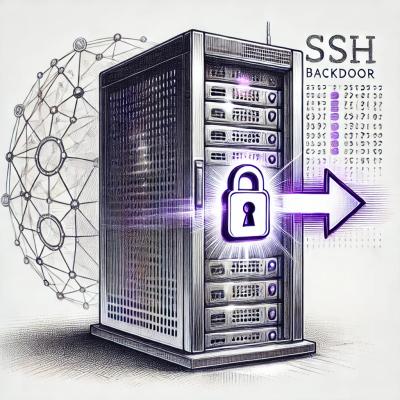
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
$ microbundle
Build output to build:
1.13 kB: index.js
1.14 kB: index.m.js
1.19 kB: index.umd.js
mounted
and unmounted
lifecycle functions using built-in JavaScript MutationObservers
.API Function Index
$ npm install literaljs
$ yarn add literaljs
const Literal = require('literaljs');
// OR
import Literal from 'literaljs';
// OR
import { component, render } from 'literaljs';
import { component, render } from 'literaljs';
const foo = component({
render: ({ getState, setState }) => ({
element: 'div',
class: 'container',
text: `This is the Count: ${getState().count}`,
children: [
{
element: 'button',
text: 'Click Me!',
events: {
type: 'click',
action: () => {
var state = getState();
setState({ count: state.count + 1 });
}
}
}
]
})
});
render(foo, 'app', { count: 0 });
At the moment, all components are created with just objects. There is also more succinct markup and use for those who have access to ES6+ features like object destructuring.
In the near future regular html markup will be also possible as well!
The component
function accepts objects with the following keys:
props
The props
of a component
is an object which can be passed with anything that is normally valid in a JavaScript Object. This can include any data or functions.
The only component that can not recieve props
is the root component that is passed to the Literal.render
function.
Example:
const bar = component({
render: ({ getState, setState, props }) => ({
element: 'p',
text: `Total: ${props.total}`
})
});
const foo = component({
render: ({ getState, setState }) => ({
element: 'div',
children: [
bar({ props: { total: 9 } })
]
})
});
methods
The methods
key of a component
will accept a function which returns an object of other functions. Within the methods
, you also have access to a component
s getState
and setState
functions which can be used accordingly. You also, have access to created methods directly within the render
function.
Example:
component({
methods: ({ getState, setState }) => ({
increase: () => {
const count = getState().count;
setState({ count: count + 1 });
}
}),
render: ({ getState, setState, methods: { increase }}) => ({
element: 'button',
text: `Current Count: ${getState().count}`,
events: {
type: 'click',
action: () => increase()
}
})
});
render
The render
key of a component
accepts a function that returns an JavaScript Object.
Markup is currently written with Objects with the following specified keys:
* = Required Key
The element
key in the render
function markup is the HTML node tag name as a String
. For example, an element
with the value
The element
key is required when generating a new DOM node.
Example:
component({
render: () => ({
element: 'div',
})
});
// Outputs: <div></div>
The text
key in the render
function markup accepts a String an will generate a text inside the element.
Example:
component({
render: () => ({
element: 'div',
text: 'Hello World!'
})
});
// Outputs: <div>Hello World!</div>
The class
key in the render
function markup is the HTML node class name as a String
.
Example:
component({
render: () => ({
element: 'div',
class: 'title'
})
});
// Outputs: <div class="title"></div>
The id
key in the render
function markup is the HTML node class name as a String
.
Example:
component({
render: () => ({
element: 'div',
id: '2'
})
});
// Outputs: <div id="2"></div>
The children
key in the render
function markup is the childNodes
of a given element
or DOM Node. This key only accepts an Array of Objects. Each Object in this Array can be another Object that represents a new DOM Node.
Example:
component({
render: () => ({
element: 'div',
children: [
{
element: 'span'
}
]
})
});
// Outputs: <div id="2"></div>
The events
key in the render
function markup accepts either an Array of events or a single event Object.
An event object has two keys:
type
(Event Name)action
(Function To Trigger)Example:
// An Array Of Events
component({
render: () => ({
element: 'button',
text: 'Click Me!',
events: [
{
type: 'click'
action: () => console.log('Hello World!')
}
]
})
});
// Output in Console: Hello World!
// OR
component({
render: () => ({
element: 'button',
text: 'Click Me!',
events: {
type: 'click'
action: () => console.log('Hello World!')
}
})
});
Besides the common event handlers like click
, there are two lifecycle events that LiteralJS
includes that can be attached to any DOM node.
To do so, just name the type
value unmounted or
mounted` to trigger functions when a DOM node is first placed in the DOM or removed from the DOM.
Example: Example:
component({
render: () => ({
element: 'div',
text: 'I have a life!',
events: [
{
type: 'mounted'
action: () => console.log('I\'m on the page!')
},
{
type: 'unmounted'
action: () => console.log('I\'m off the page!')
}
]
})
});
Other keys are also accepted in a DOM object, such as data
and other custom keys.
Within the render
function of a component, you have access to other functions and objects:
getState
The getState
function of the component
s render
method will allow you to access the global store of your application.
setState
The setState
function of the component
s render
method accepts an object which will allow you to set new values for particular pieces of state in the global store
props
The props
object of the component
s render method can contain any number of data types and functions. The only
componentthat cannot contain
propsis the root
componentthat is passed to the
Literal.render` method.
methods
The methods
object allow you to access other functions defined directly in the component
. You can use this as a way to organize other pieces of logic and keep the render
function much cleaner.
The Literal.render
function is responsible for parsing the AST that is provided with component
s, injecting the generated markup into the specific DOM node, diffing any new changes based on state, and setting the default application state.
The Literal.render
function can accept 3 arguments, one of which is optional.
id
of the DOM node to inject generated markup with.Example:
Literal.render(component, 'app', { count: 0 });
// or
render(component, 'app');
FAQs
A small JavaScript library for building reactive user interfaces.
The npm package literaljs receives a total of 6 weekly downloads. As such, literaljs popularity was classified as not popular.
We found that literaljs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.