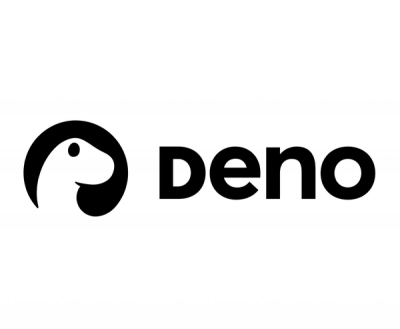
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
literate-ts
Advanced tools
Literate TS helps you verify TypeScript code samples in written text (blog posts, books, etc.). It was developed and used to type check Effective TypeScript (O'Reilly 2019).
It does this by letting you assert three things:
Expected errors. To assert the existence of an error, use a comment with a squiggly underscore in it:
let str = 'not a number';
let num: number = str;
// ~~~ Type 'string' is not assignable to type 'number'.
Literate TS will verify that this error occurs in your code sample, and no others.
Types. To assert that the type of an expression is what you expect, use a comment starting with "type is":
'four score'.split(' '); // type is string[]
Literate TS will verify that the type is precisely what you specify textually, ala dtslint.
Output. To assert the output of a code sample, give it an ID and include a paired one ending
with -output
. For example:
[[sample]]
[source,ts]
----
console.log('Hello');
----
[[sample-output]]
....
Hello
....
Literate TS will convert your code sample to JavaScript, run it and diff the output.
$ yarn
$ yarn ts-node index.ts examples/asciidoc/sample.asciidoc
Logging details to /var/folders/st/8n5l6s0139x5dwpxfhl0xs3w0000gn/T/tmp-96270LdwL51L23N9D/log.txt
Verifying with TypeScript 3.6.2
examples/asciidoc/sample.asciidoc 5/5 passed
✓ sample-6
✓ sample-17
✓ sample-25
✓ sample-32
✓ sample-41
✓ All samples passed!
✨ Done in 9.24s.
In Asciidoc, directives begin with // verifier
:
// verifier:reset
// verifier:skip
// verifier:prepend-to-following
// verifier:prepend-subset-to-following:A-B
// verifier:prepend-id-to-following:ID
// verifier:tsconfig:setting=value
FAQs
Code samples that scale
The npm package literate-ts receives a total of 2 weekly downloads. As such, literate-ts popularity was classified as not popular.
We found that literate-ts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.