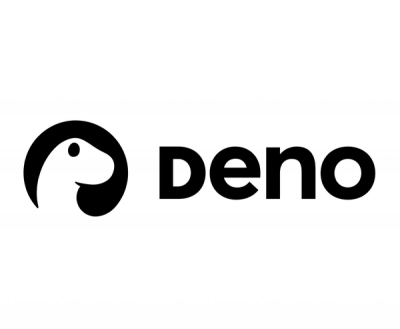
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Fast linked list with live iterator. The list can be modified while iterating over the items.
Repository: https://github.com/mantoni/live-list.js
npm install live-list
To use this module in a browser, download the npm package and then use Browserify to create a standalone version.
var List = require('live-list').List;
var l = new List();
l.push(3);
l.unshift(1);
l.insert(2, 3);
var v, i = l.iterator();
while ((v = i.next()) !== undefined) {
console.log(v);
}
length
: Reflects the number of items in the listpush(value)
: Appends a value to the listunshift(value)
: Prepends a value to the listinsert(value, before)
: Inserts a value before another valueremove(value)
: Removes the given value from the listremoveAll()
: Removes all values from the listtoArray()
: Returns a new array with all values in the listiterator()
: Returns a new Iterator
The iterator is derived from min-iterator and extends the API with these functions:
insert(value)
: Inserts a value into the list before the value
returned by the last call to next()
remove()
: Removes the value from the list that was returned
by the last call to next()
MIT
FAQs
Fast linked list with live iterator
The npm package live-list receives a total of 9 weekly downloads. As such, live-list popularity was classified as not popular.
We found that live-list demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.