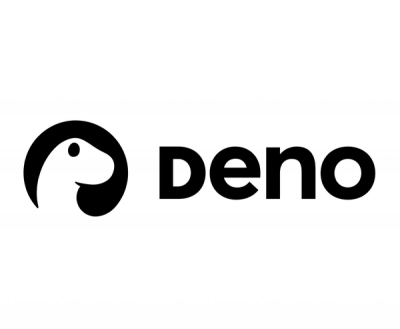
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
lodash.forown
Advanced tools
The lodash.forown package is a utility function from the Lodash library that iterates over own enumerable string keyed properties of an object and invokes a function for each property. This is useful for object manipulation and iteration tasks.
Iterate over object properties
This feature allows you to iterate over the own enumerable string keyed properties of an object. The provided function is invoked for each property, receiving the value and key as arguments.
const _ = require('lodash.forown');
const object = { 'a': 1, 'b': 2, 'c': 3 };
_.forOwn(object, function(value, key) {
console.log(key, value);
});
Early exit from iteration
This feature allows you to exit the iteration early by returning `false` from the iteratee function. This can be useful when you need to stop processing once a certain condition is met.
const _ = require('lodash.forown');
const object = { 'a': 1, 'b': 2, 'c': 3 };
_.forOwn(object, function(value, key) {
if (key === 'b') {
return false;
}
console.log(key, value);
});
The object-foreach package provides similar functionality to lodash.forown by iterating over the own properties of an object. It is a lightweight alternative but lacks the extensive utility functions provided by Lodash.
The modern build of lodash’s _.forOwn
exported as a Node.js/io.js module.
Using npm:
$ {sudo -H} npm i -g npm
$ npm i --save lodash.forown
In Node.js/io.js:
var forOwn = require('lodash.forown');
See the documentation or package source for more details.
FAQs
The lodash method `_.forOwn` exported as a module.
The npm package lodash.forown receives a total of 189,474 weekly downloads. As such, lodash.forown popularity was classified as popular.
We found that lodash.forown demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.