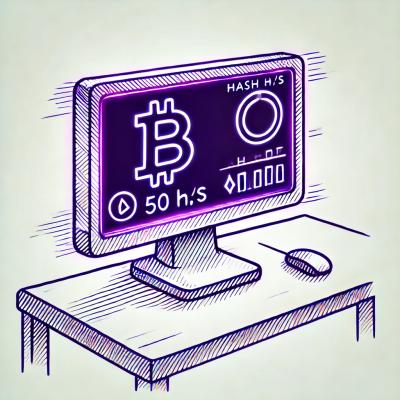
Security News
Research
Supply Chain Attack on Rspack npm Packages Injects Cryptojacking Malware
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
lodash.mergewith
Advanced tools
The lodash.mergewith package is a utility library that provides a method for deep merging objects. It allows for custom merge functions to be specified, enabling more complex merging strategies than the default deep merge.
Deep Merging Objects
This feature allows for deep merging of objects with a custom merge function. In this example, arrays are concatenated instead of being replaced.
const _ = require('lodash.mergewith');
const object = { 'a': [{ 'b': 2 }, { 'd': 4 }] };
const other = { 'a': [{ 'c': 3 }, { 'e': 5 }] };
function customizer(objValue, srcValue) {
if (Array.isArray(objValue)) {
return objValue.concat(srcValue);
}
}
const result = _.mergeWith(object, other, customizer);
console.log(result); // { 'a': [{ 'b': 2, 'c': 3 }, { 'd': 4, 'e': 5 }] }
The deepmerge package is another utility for deep merging objects. It provides a simple API for merging objects and arrays, but does not support custom merge functions out of the box. It is useful for straightforward deep merges without the need for custom logic.
The merge-options package allows for deep merging of objects with options for customizing the merge behavior. It is similar to lodash.mergewith in that it supports custom merge functions, but it provides a different API and additional options for controlling the merge process.
The Lodash method _.mergeWith
exported as a Node.js module.
Using npm:
$ {sudo -H} npm i -g npm
$ npm i --save lodash.mergewith
In Node.js:
var mergeWith = require('lodash.mergewith');
See the documentation or package source for more details.
FAQs
The Lodash method `_.mergeWith` exported as a module.
The npm package lodash.mergewith receives a total of 3,847,982 weekly downloads. As such, lodash.mergewith popularity was classified as popular.
We found that lodash.mergewith demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.
Security News
Sonar’s acquisition of Tidelift highlights a growing industry shift toward sustainable open source funding, addressing maintainer burnout and critical software dependencies.