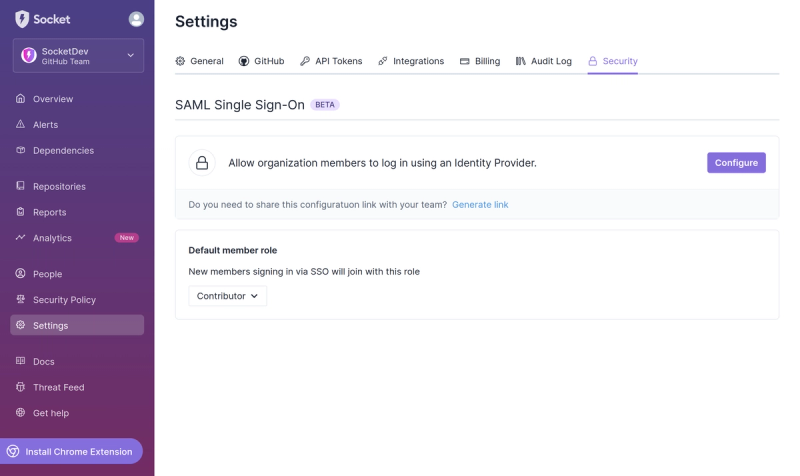
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
lodash.template
Advanced tools
Package description
The lodash.template package is a part of the Lodash library which provides utility functions for common programming tasks using the functional programming paradigm. It is specifically designed for creating compiled templates which can be used to interpolate values into strings.
Template Compilation
Compiles templates into functions that can be used to interpolate values. The 'template' function takes a string and returns a compiled template function.
const _ = require('lodash.template');
const compiled = _.template('hello <%= user %>!');
console.log(compiled({ 'user': 'fred' }));
// => 'hello fred!'
HTML Escaping
Supports HTML escaping. The '<%-' sequence in the template string is used to escape values that are interpolated, preventing XSS attacks.
const _ = require('lodash.template');
const compiled = _.template('<b><%- value %></b>');
console.log(compiled({ 'value': '<script>' }));
// => '<b><script></b>'
Use of JavaScript Expressions
Allows the use of JavaScript expressions inside templates. This enables iteration and conditional logic within the template string.
const _ = require('lodash.template');
const compiled = _.template('<% _.forEach(users, function(user) { %><li><%- user %></li><% }); %>');
console.log(compiled({ 'users': ['fred', 'barney'] }));
// => '<li>fred</li><li>barney</li>'
Custom Delimiters
Supports custom delimiters. Users can define their own delimiters for interpolation, evaluation, and escaping.
const _ = require('lodash.template');
const compiled = _.template('hello ${ user }!', { 'interpolate': /\${([\s\S]+?)}/g });
console.log(compiled({ 'user': 'pebbles' }));
// => 'hello pebbles!'
Handlebars is a popular templating engine that is more feature-rich than lodash.template. It supports helpers, partials, and complex expressions, making it suitable for more complex templating tasks.
Mustache is a logic-less templating syntax that can be used for HTML, config files, source code, etc. It is simpler than lodash.template and does not allow for logic within the templates, focusing on simple tag replacement.
EJS, or Embedded JavaScript templates, is a templating engine that allows developers to generate HTML markup with plain JavaScript. It offers similar functionality to lodash.template but with different syntax and additional features like partials and layouts.
Formerly known as Jade, Pug is a high-performance template engine heavily influenced by Haml and implemented with JavaScript for Node.js and browsers. It offers a robust feature set including includes, inheritance, and mixins, which are not present in lodash.template.
Readme
The Lodash method _.template
exported as a Node.js module.
Using npm:
$ {sudo -H} npm i -g npm
$ npm i --save lodash.template
In Node.js:
var template = require('lodash.template');
See the documentation or package source for more details.
FAQs
The Lodash method `_.template` exported as a module.
The npm package lodash.template receives a total of 2,658,695 weekly downloads. As such, lodash.template popularity was classified as popular.
We found that lodash.template demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.