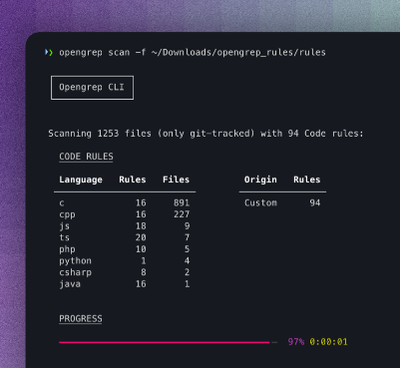
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
logagent-js
Advanced tools
Smart Log Parser and Log Shipper written in Node.
The parser detects log formats based on a pattern library (yaml file) and converts it to a JSON Object:
To test patterns or convert logs from text to JSON use the command line tool 'logagent'. It reads from stdin and outputs line delimited JSON (or pretty JSON or YAML) to the console. In addtion it can forward the parsed objects directly to Logsene.
The file format is based on JS-YAML, in short:
Properties:
Example:
# Sensitive data can be replaced with a hashcode (sha1)
# it applies to fields matching the field names by a regular expression
# Note: this function is not optimized (yet) and might take 10-15% of performance
# autohash: !!js/regexp /user|client_ip|password|email|credit_card_number|payment_info/i
patterns:
- # APACHE Web Logs
sourceName: httpd
match:
# Common Log Format
- regex: !!js/regexp /([0-9a-f.:]+)\s+(-|.+?)\s+(-|.+?)\s+\[([0-9]{2}\/[a-z]{3}\/[0-9]{4}\:[0-9]{2}:[0-9]{2}:[0-9]{2}[^\]]*)\] \"(\S+?)\s(\S*?)\s{0,1}(\S+?)\" ([0-9|\-]+) ([0-9|\-]+)/i
type: apache_access_common
fields: [client_ip,remote_id,user,ts,method,path,http_version,status_code,size]
dateFormat: DD/MMM/YYYY:HH:mm:ss ZZ
transform: !!js/function >
function (p) {
p.message = p.method + ' ' + p.path
}
The default patterns are here - contributions are welcome.
npm i logagent-js --save
var Logparser = require('logagent-js')
var lp = new Logparser('./patterns.yml')
lp.parseLine('log message', 'source name', function (err, data) {
if(err) {
console.log('line did not match with any pattern')
}
console.log(JSON.stringify(data))
})
Test your patterns:
cat some.log | bin/logagent -y -f mypatterns.yml
# Note the new setup script name for Node.js v0.12
curl -sL https://deb.nodesource.com/setup_0.12 | sudo bash -
# Then install with:
sudo apt-get install -y nodejs
npm i -g logagent-js
# ship all your logs to logsene, parsed, timestamped - displyed on console in YAML format (-y)
logagent -t LOGSENE_TOKEN -y /var/log/*.log
Parameters:
The default output is line delimited JSON.
Examples:
# Be Evil: parse all logs
# stream logs to Logsene 1-Click ELK stack
logagent -t LOGSENE_TOKEN /var/log/*.log
# Act as syslog server on UDP and forward messages to Logsene
logagent -t LOGSENE_TOKEN -u 1514
# Act as syslog server on UDP and write YAML formated messages to console
logagent -u 1514 -y
Use a glob pattern to build the file list
logagent -t LOGSENE_TOKEN -g "{/var/log/*.log,/opt/myapp/*.log}"
Watch selective log output on console by passing logs via stdin and format in YAML
tail -f /var/log/access.log | logagent -y
tail -f /var/log/system.log | logagent -f my_own_patterns.yml -y
Modify this script and place it in /etc/init/logagent.conf
description "Upstart Logagent"
start on (local-filesystems and net-device-up IFACE=eth0)
stop on runlevel [!12345]
respawn
setuid syslog
setgid syslog
env NODE_ENV=production
env LOGSENE_TOKEN=YOUR_LOGSENE_TOKEN
chdir /var/log
exec /usr/local/bin/logagent -s /var/log/*.log
Start the service:
sudo service logagent start
Create a service file for the logagent, in /etc/systemd/system/logagent.service Set the Logsene Token and file list in "ExecStart" directive.
[Service]
Description=Sematext logagent-js
Environment=NODE_ENV=production
ExecStart=/usr/local/bin/logagent -s -t YOUR_LOGSENE_TOKEN /var/log/*.log
Restart=always
StandardOutput=syslog
StandardError=syslog
SyslogIdentifier=logagent
User=syslog
Group=syslog
[Install]
WantedBy=multi-user.target
Start the service
systemctl start logagent
FAQs
Smart log parser written in Node
The npm package logagent-js receives a total of 31 weekly downloads. As such, logagent-js popularity was classified as not popular.
We found that logagent-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.