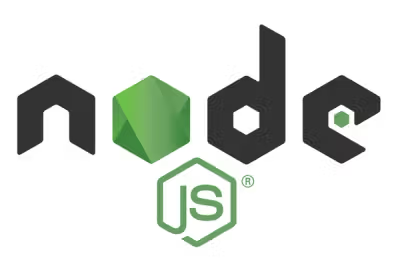
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
loopback-connector-mysql
Advanced tools
loopback-connector-mysql
is the MySQL connector module for loopback-datasource-juggler.
npm install loopback-connector-mysql --save
To use it you need loopback-datasource-juggler
.
Setup dependencies in package.json
:
{
...
"dependencies": {
"loopback-datasource-juggler": "latest",
"loopback-connector-mysql": "latest"
},
...
}
Use:
var DataSource = require('loopback-datasource-juggler').DataSource;
var dataSource = new DataSource('mysql', {
host: 'localhost',
port: 3306,
database: 'mydb',
username: 'myuser',
password: 'mypass'
});
You can optionally pass a few additional parameters supported by node-mysql
,
most particularly password
and collation
. Collation
currently defaults
to utf8_general_ci
. The collation
value will also be used to derive the
connection charset.
loopback-connector-mysql
uses the following rules to map between JSON types and MySQL data types.
dataType
field/column option with MySQLloopback-connector-mysql
allows mapping of LoopBack model properties to MYSQL columns using the 'mysql' property of the
property definition. For example,
"locationId":{
"type":"String",
"required":true,
"length":20,
"mysql":
{
"columnName":"LOCATION_ID",
"dataType":"VARCHAR2",
"dataLength":20,
"nullable":"N"
}
}
loopback-connector-mysql
also supports using the dataType
column/property attribute to specify what MySQL column
type is used for many loopback-datasource-juggler types.
The following type-dataType combinations are supported:
Number
integer
Use the limit
option to alter the display width.
Example:
{ count : { type: Number, dataType: 'smallInt' }}
floating point types
Use the precision
and scale
options to specify custom precision. Default is (16,8).
Example:
{ average : { type: Number, dataType: 'float', precision: 20, scale: 4 }}
fixed-point exact value types
Use the precision
and scale
options to specify custom precision. Default is (9,2).
These aren't likely to function as true fixed-point.
Example:
{ stdDev : { type: Number, dataType: 'decimal', precision: 12, scale: 8 }}
String / DataSource.Text / DataSource.JSON
Example:
{ userName : { type: String, dataType: 'char', limit: 24 }}
Example:
{ biography : { type: String, dataType: 'longtext' }}
Date
Example:
{ startTime : { type: Date, dataType: 'timestamp' }}
var MOOD = dataSource.EnumFactory('glad', 'sad', 'mad');
MOOD.SAD; // 'sad'
MOOD(2); // 'sad'
MOOD('SAD'); // 'sad'
MOOD('sad'); // 'sad'
{ mood: { type: MOOD }}
{ choice: { type: dataSource.EnumFactory('yes', 'no', 'maybe'), null: false }}
MySQL data sources allow you to discover model definition information from existing mysql databases. See the following APIs:
MySQL.prototype.discoverModelDefinitions = function (options, cb)
Get a list of table/view names, for example:
{type: 'table', name: 'INVENTORY', owner: 'STRONGLOOP' } {type: 'table', name: 'LOCATION', owner: 'STRONGLOOP' } {type: 'view', name: 'INVENTORY_VIEW', owner: 'STRONGLOOP' }
MySQL.prototype.discoverModelProperties = function (table, options, cb)
Get a list of model property definitions, for example:
{ owner: 'STRONGLOOP',
tableName: 'PRODUCT',
columnName: 'ID',
dataType: 'VARCHAR2',
dataLength: 20,
nullable: 'N',
type: 'String' }
{ owner: 'STRONGLOOP',
tableName: 'PRODUCT',
columnName: 'NAME',
dataType: 'VARCHAR2',
dataLength: 64,
nullable: 'Y',
type: 'String' }
MySQL.prototype.discoverPrimaryKeys= function(table, options, cb)
Get a list of primary key definitions, for example:
{ owner: 'STRONGLOOP', tableName: 'INVENTORY', columnName: 'PRODUCT_ID', keySeq: 1, pkName: 'ID_PK' } { owner: 'STRONGLOOP', tableName: 'INVENTORY', columnName: 'LOCATION_ID', keySeq: 2, pkName: 'ID_PK' }
MySQL.prototype.discoverForeignKeys= function(table, options, cb)
Get a list of foreign key definitions, for example:
{ fkOwner: 'STRONGLOOP', fkName: 'PRODUCT_FK', fkTableName: 'INVENTORY', fkColumnName: 'PRODUCT_ID', keySeq: 1, pkOwner: 'STRONGLOOP', pkName: 'PRODUCT_PK', pkTableName: 'PRODUCT', pkColumnName: 'ID' }
MySQL.prototype.discoverExportedForeignKeys= function(table, options, cb)
Get a list of foreign key definitions that reference the primary key of the given table, for example:
{ fkName: 'PRODUCT_FK', fkOwner: 'STRONGLOOP', fkTableName: 'INVENTORY', fkColumnName: 'PRODUCT_ID', keySeq: 1, pkName: 'PRODUCT_PK', pkOwner: 'STRONGLOOP', pkTableName: 'PRODUCT', pkColumnName: 'ID' }
Data sources backed by the MySQL connector can discover LDL models from the database using the discoverSchema
API. For
example,
dataSource.discoverSchema('INVENTORY', {owner: 'STRONGLOOP'}, function (err, schema) {
...
}
Here is the sample result. Please note there are 'mysql' properties in addition to the regular LDL model options and properties. The 'mysql' objects contain the MySQL specific mappings.
{
"name":"Inventory",
"options":{
"idInjection":false,
"mysql":{
"schema":"STRONGLOOP",
"table":"INVENTORY"
}
},
"properties":{
"productId":{
"type":"String",
"required":false,
"length":60,
"precision":null,
"scale":null,
"id":1,
"mysql":{
"columnName":"PRODUCT_ID",
"dataType":"varchar",
"dataLength":60,
"dataPrecision":null,
"dataScale":null,
"nullable":"NO"
}
},
"locationId":{
"type":"String",
"required":false,
"length":60,
"precision":null,
"scale":null,
"id":2,
"mysql":{
"columnName":"LOCATION_ID",
"dataType":"varchar",
"dataLength":60,
"dataPrecision":null,
"dataScale":null,
"nullable":"NO"
}
},
"available":{
"type":"Number",
"required":false,
"length":null,
"precision":10,
"scale":0,
"mysql":{
"columnName":"AVAILABLE",
"dataType":"int",
"dataLength":null,
"dataPrecision":10,
"dataScale":0,
"nullable":"YES"
}
},
"total":{
"type":"Number",
"required":false,
"length":null,
"precision":10,
"scale":0,
"mysql":{
"columnName":"TOTAL",
"dataType":"int",
"dataLength":null,
"dataPrecision":10,
"dataScale":0,
"nullable":"YES"
}
}
}
}
We can also discover and build model classes in one shot. The following example uses discoverAndBuildModels
to discover,
build and try the models:
dataSource.discoverAndBuildModels('INVENTORY', { owner: 'STRONGLOOP', visited: {}, associations: true},
function (err, models) {
// Show records from the models
for(var m in models) {
models[m].all(show);
};
// Find one record for inventory
models.Inventory.findOne({}, function(err, inv) {
console.log("\nInventory: ", inv);
// Follow the foreign key to navigate to the product
inv.product(function(err, prod) {
console.log("\nProduct: ", prod);
console.log("\n ------------- ");
});
});
}
FAQs
MySQL connector for loopback-datasource-juggler
The npm package loopback-connector-mysql receives a total of 5,550 weekly downloads. As such, loopback-connector-mysql popularity was classified as popular.
We found that loopback-connector-mysql demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.