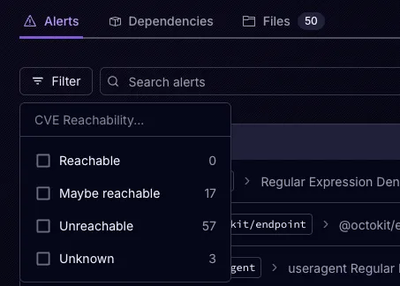
Product
Announcing Precomputed Reachability Analysis in Socket
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
mail-confirm
Advanced tools
A Node Js API for three stage email validation including, pattern, MX, and mailbox existence validation.
A Node Js API for three stage email validation including the following;
NOTE: True mailbox existence may only be performed, with certainty, by sending a verification email and having the user verify their email.
# yarn
yarn add mail-confirm
# npm
npm i mail-confirm
import MailConfirm from 'mail-confirm'
// promises
const email = new MailConfirm({
emailAddress: 'test@gmail.com',
timeout: 2000,
mailFrom: 'my@email.com',
invalidMailboxKeywords: ['noreply', 'noemail']
})
email.check().then(console.log).catch(console.log)
// async/await
const check = async (emailAddress) => {
try{
const email = new MailConfirm({ emailAddress })
const result = await email.check()
return result
}catch(err){
throw new Error(err)
}
}
check('test@gmail.com')
/*
output =>
{ emailAddress: 'test@gmail.com',
timeout: 2000,
invalidMailboxKeywords: [],
mailFrom: 'my@email.com',
mailbox: 'test',
hostname: 'gmail.com',
mxRecords:
[ { exchange: 'gmail-smtp-in.l.google.com', priority: 5 },
{ exchange: 'alt1.gmail-smtp-in.l.google.com', priority: 10 },
{ exchange: 'alt2.gmail-smtp-in.l.google.com', priority: 20 },
{ exchange: 'alt3.gmail-smtp-in.l.google.com', priority: 30 },
{ exchange: 'alt4.gmail-smtp-in.l.google.com', priority: 40 } ],
smtpMessages:
[ { command: 'HELO gmail-smtp-in.l.google.com',
message: '220 mx.google.com ESMTP n6si1346674qtk.310 - gsmtp\r\n',
status: 220 },
{ command: 'MAIL FROM: <email@example.org>',
message: '250 mx.google.com at your service\r\n',
status: 250 },
{ command: 'RCPT TO: <test@gmail.com>',
message: '250 2.1.0 OK n6si1346674qtk.310 - gsmtp\r\n',
status: 250 } ],
isValidPattern: true,
isValidMx: true,
isValidMailbox: true,
result: 'Mailbox is valid.' }
*/
Kind: global class
Object
boolean
Array.<Object>
Array.<object>
Email address validation and SMTP verification API.
Param | Type | Description |
---|---|---|
config | Object | The email address you want to validate. |
config.emailAddress | string | The email address you want to validate. |
[config.mailFrom] | string | The email address used for the mail from during SMTP mailbox validation. |
[config.invalidMailboxKeywords] | Array.<string> | Keywords you want to void, i.e. noemail, noreply etc. |
[config.timeout] | number | The timeout parameter for SMTP mailbox validation. |
Object
Runs the email validation routine and supplies a final result.
Kind: instance method of MailConfirm
Returns: Object
- - The instance state object containing all of the isValid* boolean checks, MX Records, and SMTP Messages.
boolean
Determines if the email address pattern is valid based on regex and invalid keyword check.
Kind: static method of MailConfirm
Param | Type | Default | Description |
---|---|---|---|
emailAddress | string | The full email address ypu want to check. | |
[invalidMailboxKeywords] | Array.<string> | [] | An array of keywords to invalidate your check, ie. noreply |
, noemail, etc. |
Array.<Object>
Wrap of dns.resolveMx native method.
Kind: static method of MailConfirm
Returns: Array.<Object>
- - Returns MX records array { priority, exchange }
Param | Type | Description |
---|---|---|
hostname | string | The hostname you want to resolve, i.e. gmail.com |
Array.<object>
Runs the SMTP mailbox check. Commands for HELO/EHLO, MAIL FROM, RCPT TO.
Kind: static method of MailConfirm
Returns: Array.<object>
- - Object of SMTP responses [ {command, status, message} ]
Param | Type | Description |
---|---|---|
config | Object | Object of parameters for Smtp Mailbox resolution. |
config.emailAddress | string | The email address you want to check. |
config.mxRecords | Array.<object> | The MX Records array supplied from resolveMx. |
config.timeout | number | Timeout parameter for the SMTP routine. |
config.mailFrom | string | The email address supplied to the MAIL FROM SMTP command. |
Title: MailConfirm
Author: Elias Hussary eliashussary@gmail.com
License: MIT
Copyright: (C) 2017 Elias Hussary
FAQs
A Node Js API for three stage email validation including, pattern, MX, and mailbox existence validation.
The npm package mail-confirm receives a total of 3 weekly downloads. As such, mail-confirm popularity was classified as not popular.
We found that mail-confirm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
Product
Socket is launching experimental protection for Chrome extensions, scanning for malware and risky permissions to prevent silent supply chain attacks.
Product
Add secure dependency scanning to Claude Desktop with Socket MCP, a one-click extension that keeps your coding conversations safe from malicious packages.