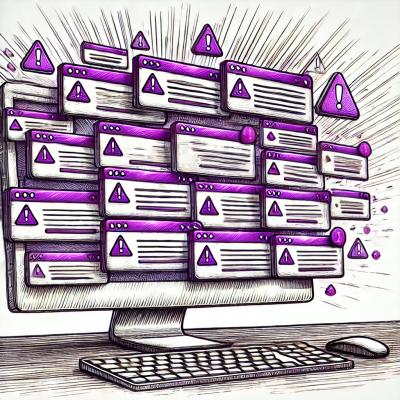
Security News
Combatting Alert Fatigue by Prioritizing Malicious Intent
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
mailcomposer
Advanced tools
The mailcomposer npm package is a tool for creating email messages with various features such as attachments, HTML content, and custom headers. It is useful for generating email content that can be sent using an SMTP client or other email transport methods.
Basic Email Composition
This feature allows you to compose a basic email with a sender, receiver, subject, and plain text content.
const MailComposer = require('mailcomposer');
const mail = new MailComposer({
from: 'sender@example.com',
to: 'receiver@example.com',
subject: 'Hello',
text: 'Hello world!'
});
mail.build((err, message) => {
if (err) {
console.error('Error:', err);
} else {
console.log('Email message:', message.toString());
}
});
HTML Email Composition
This feature allows you to compose an email with HTML content, which can include rich text formatting and images.
const MailComposer = require('mailcomposer');
const mail = new MailComposer({
from: 'sender@example.com',
to: 'receiver@example.com',
subject: 'Hello',
html: '<h1>Hello world!</h1>'
});
mail.build((err, message) => {
if (err) {
console.error('Error:', err);
} else {
console.log('Email message:', message.toString());
}
});
Attachments
This feature allows you to add attachments to your email, such as files or images.
const MailComposer = require('mailcomposer');
const mail = new MailComposer({
from: 'sender@example.com',
to: 'receiver@example.com',
subject: 'Hello',
text: 'Hello world!',
attachments: [
{
filename: 'text.txt',
content: 'Hello world!'
}
]
});
mail.build((err, message) => {
if (err) {
console.error('Error:', err);
} else {
console.log('Email message:', message.toString());
}
});
Custom Headers
This feature allows you to add custom headers to your email, which can be useful for various purposes such as tracking or custom processing.
const MailComposer = require('mailcomposer');
const mail = new MailComposer({
from: 'sender@example.com',
to: 'receiver@example.com',
subject: 'Hello',
text: 'Hello world!',
headers: {
'X-Custom-Header': 'Custom header value'
}
});
mail.build((err, message) => {
if (err) {
console.error('Error:', err);
} else {
console.log('Email message:', message.toString());
}
});
Nodemailer is a module for Node.js applications to allow easy email sending. It supports various transport methods, including SMTP, and provides a rich set of features for composing and sending emails. Compared to mailcomposer, Nodemailer is more comprehensive as it includes both email composition and transport functionalities.
EmailJS is a library for sending emails from JavaScript applications. It supports SMTP and provides features for composing and sending emails. While it offers similar functionalities to mailcomposer, it also includes built-in transport methods, making it a more complete solution for email handling.
Sendmail is a simple way to send emails from your Node.js application without needing a dedicated SMTP server. It is lightweight and easy to use, but it lacks some of the advanced composition features provided by mailcomposer.
Node.JS module for generating e-mail messages that can be streamed to SMTP or file.
See mailcomposer homepage for documentation and terms.
FAQs
Compose E-Mail messages
The npm package mailcomposer receives a total of 227,806 weekly downloads. As such, mailcomposer popularity was classified as popular.
We found that mailcomposer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.