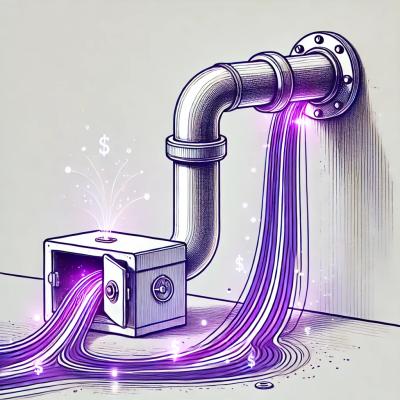
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
send text/html emails and attachments (files, streams and strings) from node.js to any smtp server
send emails, html and attachments (files, streams and strings) from node.js to any smtp server
npm install emailjs
import { SMTPClient } from 'emailjs';
const client = new SMTPClient({
user: 'user',
password: 'password',
host: 'smtp.your-email.com',
ssl: true,
});
// send the message and get a callback with an error or details of the message that was sent
client.send(
{
text: 'i hope this works',
from: 'you <username@your-email.com>',
to: 'someone <someone@your-email.com>, another <another@your-email.com>',
cc: 'else <else@your-email.com>',
subject: 'testing emailjs',
},
(err, message) => {
console.log(err || message);
}
);
// assuming top-level await for brevity
import { SMTPClient } from 'emailjs';
const client = new SMTPClient({
user: 'user',
password: 'password',
host: 'smtp.your-email.com',
ssl: true,
});
try {
const message = await client.sendAsync({
text: 'i hope this works',
from: 'you <username@your-email.com>',
to: 'someone <someone@your-email.com>, another <another@your-email.com>',
cc: 'else <else@your-email.com>',
subject: 'testing emailjs',
});
console.log(message);
} catch (err) {
console.error(err);
}
import { SMTPClient } from 'emailjs';
const client = new SMTPClient({
user: 'user',
password: 'password',
host: 'smtp.your-email.com',
ssl: true,
});
const message = {
text: 'i hope this works',
from: 'you <username@your-email.com>',
to: 'someone <someone@your-email.com>, another <another@your-email.com>',
cc: 'else <else@your-email.com>',
subject: 'testing emailjs',
attachment: [
{ data: '<html>i <i>hope</i> this works!</html>', alternative: true },
{ path: 'path/to/file.zip', type: 'application/zip', name: 'renamed.zip' },
],
};
// send the message and get a callback with an error or details of the message that was sent
client.send(message, function (err, message) {
console.log(err || message);
});
// you can continue to send more messages with successive calls to 'client.send',
// they will be queued on the same smtp connection
// or instead of using the built-in client you can create an instance of 'smtp.SMTPConnection'
import { SMTPClient, Message } from 'emailjs';
const client = new SMTPClient({
user: 'user',
password: 'password',
host: 'smtp-mail.outlook.com',
tls: {
ciphers: 'SSLv3',
},
});
const message = new Message({
text: 'i hope this works',
from: 'you <username@outlook.com>',
to: 'someone <someone@your-email.com>, another <another@your-email.com>',
cc: 'else <else@your-email.com>',
subject: 'testing emailjs',
attachment: [
{ data: '<html>i <i>hope</i> this works!</html>', alternative: true },
{ path: 'path/to/file.zip', type: 'application/zip', name: 'renamed.zip' },
],
});
// send the message and get a callback with an error or details of the message that was sent
client.send(message, (err, message) => {
console.log(err || message);
});
import { SMTPClient, Message } from 'emailjs';
const client = new SMTPClient({
user: 'user',
password: 'password',
host: 'smtp-mail.outlook.com',
tls: {
ciphers: 'SSLv3',
},
});
const message = new Message({
text: 'i hope this works',
from: 'you <username@outlook.com>',
to: 'someone <someone@your-email.com>, another <another@your-email.com>',
cc: 'else <else@your-email.com>',
subject: 'testing emailjs',
attachment: [
{
data:
'<html>i <i>hope</i> this works! here is an image: <img src="cid:my-image" width="100" height ="50"> </html>',
},
{ path: 'path/to/file.zip', type: 'application/zip', name: 'renamed.zip' },
{
path: 'path/to/image.jpg',
type: 'image/jpg',
headers: { 'Content-ID': '<my-image>' },
},
],
});
// send the message and get a callback with an error or details of the message that was sent
client.send(message, (err, message) => {
console.log(err || message);
});
// options is an object with the following recognized schema:
const options = {
user, // username for logging into smtp
password, // password for logging into smtp
host, // smtp host (defaults to 'localhost')
port, // smtp port (defaults to 25 for unencrypted, 465 for `ssl`, and 587 for `tls`)
ssl, // boolean or object (if true or object, ssl connection will be made)
tls, // boolean or object (if true or object, starttls will be initiated)
timeout, // max number of milliseconds to wait for smtp responses (defaults to 5000)
domain, // domain to greet smtp with (defaults to os.hostname)
authentication, // array of preferred authentication methods ('PLAIN', 'LOGIN', 'CRAM-MD5', 'XOAUTH2')
logger, // override the built-in logger (useful for e.g. Azure Function Apps, where console.log doesn't work)
};
// ssl/tls objects are an abbreviated form of [`tls.connect`](https://nodejs.org/dist/latest-v14.x/docs/api/tls.html#tls_tls_connect_options_callback)'s options
// the missing items are: `port`, `host`, `path`, `socket`, `timeout` and `secureContext`
// NOTE: `host` is trimmed before being used to establish a connection;
// however, the original untrimmed value will still be visible in configuration.
// message can be a smtp.Message (as returned by email.message.create)
// or an object identical to the first argument accepted by email.message.create
// callback will be executed with (err, message)
// either when message is sent or an error has occurred
// headers is an object with the following recognized schema:
const headers = {
from, // sender of the format (address or name <address> or "name" <address>)
to, // recipients (same format as above), multiple recipients are separated by a comma
cc, // carbon copied recipients (same format as above)
bcc, // blind carbon copied recipients (same format as above)
text, // text of the email
subject, // string subject of the email
attachment, // one attachment or array of attachments
};
// the `from` field is required.
// at least one `to`, `cc`, or `bcc` header is also required.
// you can also add whatever other headers you want.
Can be called multiple times, each adding a new attachment.
// options is an object with the following recognized schema:
const options = {
// one of these fields is required
path, // string to where the file is located
data, // string of the data you want to attach
stream, // binary stream that will provide attachment data (make sure it is in the paused state)
// better performance for binary streams is achieved if buffer.length % (76*6) == 0
// current max size of buffer must be no larger than Message.BUFFERSIZE
// optionally these fields are also accepted
type, // string of the file mime type
name, // name to give the file as perceived by the recipient
charset, // charset to encode attatchment in
method, // method to send attachment as (used by calendar invites)
alternative, // if true, will be attached inline as an alternative (also defaults type='text/html')
inline, // if true, will be attached inline
encoded, // set this to true if the data is already base64 encoded, (avoid this if possible)
headers, // object containing header=>value pairs for inclusion in this attachment's header
related, // an array of attachments that you want to be related to the parent attachment
};
Synchronously validate that a Message is properly formed.
const message = new Message(options);
const { isValid, validationError } = message.checkValidity();
if (isValid) {
// ...
} else {
// first error encountered
console.error(validationError);
}
// options is an object with the following recognized schema:
const options = {
user, // username for logging into smtp
password, // password for logging into smtp
host, // smtp host (defaults to 'localhost')
port, // smtp port (defaults to 25 for unencrypted, 465 for `ssl`, and 587 for `tls`)
ssl, // boolean or object (if true or object, ssl connection will be made)
tls, // boolean or object (if true or object, starttls will be initiated)
timeout, // max number of milliseconds to wait for smtp responses (defaults to 5000)
domain, // domain to greet smtp with (defaults to os.hostname)
authentication, // array of preferred authentication methods ('PLAIN', 'LOGIN', 'CRAM-MD5', 'XOAUTH2')
logger, // override the built-in logger (useful for e.g. Azure Function Apps, where console.log doesn't work)
};
// ssl/tls objects are an abbreviated form of [`tls.connect`](https://nodejs.org/dist/latest-v14.x/docs/api/tls.html#tls_tls_connect_options_callback)'s options
// the missing items are: `port`, `host`, `path`, `socket`, `timeout` and `secureContext`
// NOTE: `host` is trimmed before being used to establish a connection;
// however, the original untrimmed value will still be visible in configuration.
To target a Message Transfer Agent (MTA), omit all options.
associative array of currently supported SMTP authentication mechanisms
eleith zackschuster
npm install -d
npm test
issues and pull requests are welcome
FAQs
send text/html emails and attachments (files, streams and strings) from node.js to any smtp server
We found that emailjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.