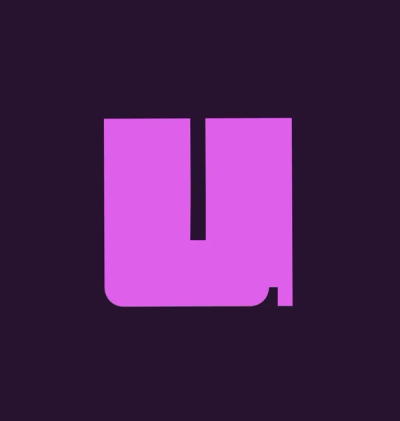
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
mailparser
Advanced tools
The mailparser npm package is a powerful tool for parsing email messages. It can handle various email formats and extract useful information such as headers, attachments, and the email body. This package is particularly useful for applications that need to process and analyze email content programmatically.
Parsing Email from a String
This feature allows you to parse an email from a raw string. The `simpleParser` function takes the raw email string and a callback function, and it returns a parsed email object containing various parts of the email such as headers, text, and attachments.
const { simpleParser } = require('mailparser');
const email = `From: sender@example.com
To: receiver@example.com
Subject: Test email
This is a test email.`;
simpleParser(email, (err, parsed) => {
if (err) {
console.error(err);
} else {
console.log(parsed);
}
});
Parsing Email from a Stream
This feature allows you to parse an email from a stream, such as a file stream. The `simpleParser` function can take a stream as input and parse the email content, making it useful for processing large email files.
const { simpleParser } = require('mailparser');
const fs = require('fs');
const emailStream = fs.createReadStream('path/to/email.eml');
simpleParser(emailStream, (err, parsed) => {
if (err) {
console.error(err);
} else {
console.log(parsed);
}
});
Extracting Attachments
This feature allows you to extract attachments from an email. The parsed email object contains an `attachments` array, where each attachment object includes properties like `filename` and `content`.
const { simpleParser } = require('mailparser');
const email = `From: sender@example.com
To: receiver@example.com
Subject: Test email
This is a test email with an attachment.`;
simpleParser(email, (err, parsed) => {
if (err) {
console.error(err);
} else {
parsed.attachments.forEach(attachment => {
console.log(attachment.filename);
console.log(attachment.content);
});
}
});
Nodemailer is a module for Node.js applications to allow easy email sending. While it focuses on sending emails rather than parsing them, it can be used in conjunction with mailparser for a complete email handling solution.
The imap package is used to connect to and interact with IMAP email servers. It allows you to fetch emails from an IMAP server, which can then be parsed using mailparser. It is more focused on email retrieval rather than parsing.
Mail-listener2 is a library for listening to incoming emails using IMAP. It can be used to monitor an email account and retrieve new emails, which can then be parsed using mailparser. It is useful for real-time email processing.
NB! This version of MailParser is incompatible with pre 0.2.0, do not upgrade from 0.1.x without updating your code, the API is totally different. Also if the source is coming directly from SMTP you need to unescape dots in the beginning of the lines yourself!
MailParser is an asynchronous and non-blocking parser for node.js to parse mime encoded e-mail messages. Handles even large attachments with ease - attachments can be parsed in chunks and streamed if needed.
MailParser parses raw source of e-mail messages into a structured object.
No need to worry about charsets or decoding quoted-printable or base64 data, MailParser (with the help of node-iconv) does all of it for you. All the textual output from MailParser (subject line, addressee names, message body) is always UTF-8.
npm install mailparser
Require MailParser module
var MailParser = require("mailparser").MailParser;
Create a new MailParser object
var mailparser = new MailParser();
MailParser object is a writable Stream - you can pipe directly files etc. to it
or you can send new chunks with mailparser.write
When the parsing ends an 'end' event is emitted which has an object with parsed e-mail.
mailparser.on("end", function(mail){
mail; // object structure for parsed e-mail
});
[{key: "key", value: "value"}]
From
addresses - [{address:'sender@example.com',name:'Sender Name'}]
To
addresseshtml
and text
- [{contentType:"text/plain", content: "..."}]
This example decodes an e-mail from a string
var MailParser = require("mailparser").MailParser,
mailparser = new MailParser();
var email = "From: 'Sender Name' <sender@example.com>\r\n"+
"To: 'Receiver Name' <receiver@example.com>\r\n"+
"Subject: Hello world!\r\n"+
"\r\n"+
"How are you today?";
// setup an event listener when the parsing finishes
mailparser.on("end", function(mail_object){
console.log("From:", mail_object.from); //[{address:'sender@example.com',name:'Sender Name'}]
console.log("Subject:", mail_object.subject); // Hello world!
console.log("Text body:", mail_object.text); // How are you today?
});
// send the email source to the parser
mailparser.write(email);
mailparser.end();
This example pipes a readableStream
file to MailParser
var MailParser = require("mailparser").MailParser,
mailparser = new MailParser(),
fs = require("fs");
mailparser.on("end", function(mail_object){
console.log("Subject:", mail_object.subject);
});
fs.createReadStream("email.eml").pipe(mailparser);
By default any attachment found from the e-mail will be included fully in the final mail structure object as Buffer objects. With large files this might not be desirable so optionally it is possible to redirect the attachments to a Stream and keep only the metadata about the file in the mail structure.
mailparser.on("end", function(mail_object){
for(var i=0; i<mail_object.attachments.length; i++){
console.log(mail_object.attachments[i].fileName);
}
});
By default attachments will be included in the attachment objects as Buffers.
attachments = [{
contentType: 'image/png',
fileName: 'image.png',
contentDisposition: 'attachment',
contentId: '5.1321281380971@localhost',
transferEncoding: 'base64',
length: 126,
generatedFileName: 'image.png',
checksum: 'e4cef4c6e26037bcf8166905207ea09b',
content: <Buffer ...>
}];
The property generatedFileName
is usually the same but if several different
attachments with the same name exist, the latter ones names will be modified and
the generated name will be saved to generatedFileName
file.txt
file-1.txt
file-2.txt
...
Property content
is always a Buffer object (or SlowBuffer on some occasions)
Attachment streaming can be used when providing an optional options parameter
to the MailParser
constructor.
var mp = new MailParser({
streamAttachments: true
}
This way there will be no content
property on final attachment objects (but the other fields will remain).
To catch the streams you should listen for attachment
events on the MailParser
object. The parameter provided includes file information (contentType
,
fileName
, contentId
) and a readable Stream object stream
.
var mp = new MailParser({
streamAttachments: true
}
mp.on("attachment", function(attachment){
var output = fs.createWriteStream(attachment.fileName);
attachment.stream.pipe(output);
});
In this case the fileName
parameter is equal to generatedFileName
property
on the main attachment object, you match attachment streams to the main attachment
objects through these values.
Attachment objects include length
property which is the length of the attachment
in bytes and checksum
property which is md5 hash of the file.
MIT
FAQs
Parse e-mails
The npm package mailparser receives a total of 575,465 weekly downloads. As such, mailparser popularity was classified as popular.
We found that mailparser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.