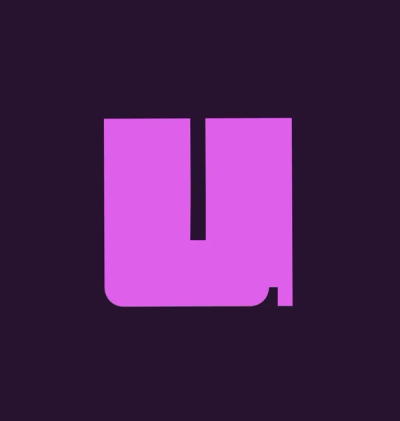
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
makeup-active-descendant
Advanced tools
Implements ARIA active descendant keyboard navigation.
This CommonJS module is still in an experimental state, until it reaches v1.0.0 you must consider all minor releases as breaking changes. Patch releases may introduce new features, but will be backwards compatible.
// via npm
npm install makeup-active-descendant
// via yarn
yarn add makeup-active-descendant
In this example the focusable element is not an ancestor of the "descendant" elements. The module will add aria-owns
to create a programmatic relationship between the two elements. This is typical of a combobox or date-picker type pattern.
Starting markup:
<div class="widget">
<input type="text">
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
</div>
// require the module
const ActiveDescendant = require('makeup-active-descendant');
// get the widget root element reference
const widgetEl = document.querySelector('.widget');
// get the focus element reference
const focusEl = widgetEl.querySelector('input');
// get the element that contains the "descendant" items.
// This element will be programmatically "owned" by the focus element.
const containerEl = widgetEl.querySelector('ul');
// create an activeDescendant widget instance on the element
const activeDescendant = ActiveDescendant.createLinear(widgetEl, focusEl, containerEl, 'li');
// listen for events (optional)
widgetEl.addEventListener('activeDescendantChange', function(e) {
console.log(e.detail);
});
Markup after instantiation:
<div class="widget" id="widget-0">
<input type="text" aria-owns="widget-0-list-0">
<ul id="widget-0-list-0">
<li id="widget-0-item-0" data-makeup-index="0">Item 1</li>
<li id="widget-0-item-1" data-makeup-index="1">Item 2</li>
<li id="widget-0-item-2" data-makeup-index="2">Item 3</li>
</ul>
</div>
Markup after pressing down arrow key on focusable element:
<div class="widget" id="widget-0">
<input type="text" aria-activedescendant="widget-0-item-0" aria-owns="widget-0-list-0">
<ul id="widget-0-list-0">
<li class="active-descendant" id="widget-0-item-0" data-makeup-index="0">Item 1</li>
<li id="widget-0-item-1" data-makeup-index="1">Item 2</li>
<li id="widget-0-item-2" data-makeup-index="2">Item 3</li>
</ul>
</div>
Use CSS to style the active descendant however you wish:
.widget .active-descendant {
font-weight: bold;
}
In this example the focusable element is an ancestor of the list items and therefore the "descendant" relationship can be automatically determined from the DOM hierarchy. This is typical of a standalone listbox or grid widget.
NOTE: this module does not add any ARIA roles; only states and properties.
Starting markup:
<div class="widget">
<ul tabindex="0">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
</div>
// require the module
const ActiveDescendant = require('makeup-active-descendant');
// get the widget root element reference
const widgetEl = document.querySelector('.widget');
// get the focus element reference
const focusEl = widgetEl.querySelector('ul');
// in this scenario the container element is the same as the focusable element
const containerEl = focusEL;
// create an activeDescendant widget instance on the element
const activeDescendant = ActiveDescendant.createLinear(widgetEl, focusEl, containerEl, 'li');
// listen for events (optional)
widgetEl.addEventListener('activeDescendantChange', function(e) {
console.log(e.detail);
});
Markup after instantiation:
<div class="widget" id="widget-0">
<ul id="widget-0-list-0" tabindex="0">
<li id="widget-0-item-0" data-makeup-index="0">Item 1</li>
<li id="widget-0-item-1" data-makeup-index="1">Item 2</li>
<li id="widget-0-item-2" data-makeup-index="2">Item 3</li>
</ul>
</div>
Markup after pressing down arrow key on focusable element:
<div class="widget" id="widget-0">
<ul id="widget-0-list-0" aria-activedescendant="widget-0-item-0" tabindex="0">
<li class="active-descendant" id="widget-0-item-0" data-makeup-index="0">Item 1</li>
<li id="widget-0-item-1" data-makeup-index="1">Item 2</li>
<li id="widget-0-item-2" data-makeup-index="2">Item 3</li>
</ul>
</div>
Use CSS to style the active descendant however you wish:
.widget .active-descendant {
font-weight: bold;
}
activeDescendantClassName
: the HTML class name that will be applied to the ARIA active descendant element (default: 'active-descendant')autoInit
: specify an integer or -1 for initial index (default: 0) (see makeup-navigation-emitter
)autoReset
: specify an integer or -1 for index position when focus exits widget (default: null) (see makeup-navigation-emitter
)autoScroll
: Specify true to scroll the container as activeDescendant changes (default: false)axis
: specify 'x' for left/right arrow keys, 'y' for up/down arrow keys, or 'both' (default: 'both')ignoreButtons
: if set to true, nested button elements will not trigger navigationModelChange events. This is useful in a combobox + button scenario, where only the textbox should trigger navigationModelChange events (default: false)activeDescendantChange
filteredItems
: returns filtered items (e.g. non-hidden items)index
: the index position of the current active descendantitems
: returns all items that match item selectordestroy
: destroys all event listenersreset
: will force a reset to the value specified by autoReset
FAQs
Implements ARIA active descendant keyboard navigation
The npm package makeup-active-descendant receives a total of 104 weekly downloads. As such, makeup-active-descendant popularity was classified as not popular.
We found that makeup-active-descendant demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.