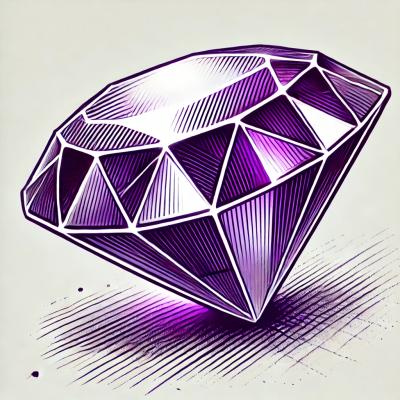
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
markdownlint
Advanced tools
markdownlint is a Node.js package that provides a linter for Markdown files. It helps ensure that Markdown files adhere to a consistent style and are free from common errors. The package can be used programmatically or via the command line, and it supports custom rules and configurations.
Linting Markdown Files
This feature allows you to lint Markdown files to ensure they follow specified rules. The code sample demonstrates how to lint a file named 'README.md' with a custom configuration that disables the 'MD013' rule.
const markdownlint = require('markdownlint');
const options = {
files: [ 'README.md' ],
config: {
'default': true,
'MD013': false
}
};
markdownlint(options, function callback(err, result) {
if (!err) {
console.log(result.toString());
}
});
Custom Rules
This feature allows you to define and use custom rules for linting. The code sample shows how to include custom rules from a file named 'custom-rules.js' and apply them to 'README.md'.
const markdownlint = require('markdownlint');
const customRules = require('./custom-rules');
const options = {
files: [ 'README.md' ],
customRules: customRules
};
markdownlint(options, function callback(err, result) {
if (!err) {
console.log(result.toString());
}
});
Command Line Interface
markdownlint can be used via the command line to lint files. The code sample demonstrates how to lint 'README.md' using a configuration file named '.markdownlint.json'.
markdownlint README.md --config .markdownlint.json
remark-lint is a Markdown code style linter built on the remark processor. It is highly customizable and can be extended with plugins. Compared to markdownlint, remark-lint offers a more modular approach and integrates well with the unified ecosystem.
markdown-it is a Markdown parser that can be extended with plugins to add linting capabilities. While it is primarily a parser, its extensibility allows for custom linting rules. It is more flexible but requires additional setup compared to markdownlint.
markdownlint-cli is a command-line interface for markdownlint. It provides similar functionality to markdownlint but is specifically designed for use in the command line. It is a good choice if you prefer a CLI tool over a programmatic API.
A Node.js style checker and lint tool for Markdown files.
npm install markdownlint --save-dev
The Markdown markup language is designed to be easy to read, write, and understand. It succeeds - and its flexibility is both a benefit and a drawback. Many styles are possible, so formatting can be inconsistent. Some constructs don't work well in all parsers and should be avoided.
markdownlint
is a static analysis
tool for Node.js and io.js with a
library of rules to enforce standards and consistency for Markdown files. It
was inspired by - and heavily influenced by - Mark Harrison's
markdownlint for
Ruby. The rules, rule documentation, and test
cases come directly from that project.
markdownlint
demo, an interactive, in-browser
playground for learning and exploring.
See Rules.md for more details.
Rules can be enabled, disabled, and configured via options.config
(described
below) to define the expected behavior for a set of inputs. To enable or disable
rules within a file, add one of these markers to the appropriate place (HTML
comments don't appear in the final markup):
<!-- markdownlint-disable -->
<!-- markdownlint-enable -->
<!-- markdownlint-disable MD001 MD002 -->
<!-- markdownlint-enable MD001 MD002 -->
For example:
<!-- markdownlint-disable MD037 -->
deliberate space * in * emphasis
<!-- markdownlint-enable MD037 -->
Changes take effect starting with the line a comment is on, so the following has no effect:
space * in * emphasis <!-- markdownlint-disable --> <!-- markdownlint-enable -->
Standard asynchronous interface:
/**
* Lint specified Markdown files according to configurable rules.
*
* @param {Object} options Configuration options.
* @param {Function} callback Callback (err, result) function.
* @returns {void}
*/
function markdownlint(options, callback) { ... }
Synchronous interface (for build scripts, etc.):
/**
* Lint specified Markdown files according to configurable rules.
*
* @param {Object} options Configuration options.
* @returns {Object} Result object.
*/
function markdownlint.sync(options) { ... }
Type: Object
Configures the function.
Type: Array
of String
List of files to lint.
Each array element should be a single file (via relative or absolute path); globbing is the caller's responsibility.
Example: [ "one.md", "dir/two.md" ]
Type: Object
mapping String
to String
Map of identifiers to strings for linting.
When Markdown content is not available as files, it can be passed as strings.
The keys of the strings
object are used to identify each input value in the
result
summary.
Example:
{
"readme": "# README\n...",
"changelog": "# CHANGELOG\n..."
}
Type: RegExp
Matches any front matter found at the beginning of a file.
Some Markdown content begins with metadata; the default RegExp
for this option
ignores common forms of "front matter". To match differently, specify a custom
RegExp
or use the value null
to disable the feature.
Note: Matches must occur at the start of the file.
Default:
/^---$[^]*?^---$(\r\n|\r|\n)/m
Ignores:
---
layout: post
title: Title
---
Type: Object
mapping String
to Boolean | Object
Configures the rules to use.
Object keys are rule names or aliases and values are the rule's configuration.
The value false
disables a rule, true
enables its default configuration,
and passing an object customizes its settings. Setting the special default
rule to true
or false
includes/excludes all rules by default. Enabling or
disabling a tag name (ex: whitespace
) affects all rules having that tag.
The default
rule is applied first, then keys are processed in order from top
to bottom with later values overriding earlier ones. Keys (including rule names,
aliases, tags, and default
) are not case-sensitive.
Example:
{
"default": true,
"MD003": { "style": "atx_closed" },
"MD007": { "indent": 4 },
"no-hard-tabs": false,
"whitespace": false
}
Sets of rules (known as a "style") can be stored separately and loaded as JSON.
Example:
var options = {
"files": [ "..." ],
"config": require("style/relaxed.json")
};
See the style directory for more samples.
Type: Function
taking (Error
, Object
)
Standard completion callback.
Type: Object
Call result.toString()
for convenience or see below for an example of the
structure of the result
object. Passing the value true
to toString()
uses rule aliases (ex: no-hard-tabs
) instead of names (ex: MD010
).
Invoke markdownlint
and use the result
object's toString
method:
var markdownlint = require("markdownlint");
var options = {
"files": [ "good.md", "bad.md" ],
"strings": {
"good.string": "# good.string\n\nThis string passes all rules.",
"bad.string": "#bad.string\n\n#This string fails\tsome rules."
}
};
markdownlint(options, function callback(err, result) {
if (!err) {
console.log(result.toString());
}
});
Output:
bad.string: 3: MD010 Hard tabs
bad.string: 1: MD018 No space after hash on atx style header
bad.string: 3: MD018 No space after hash on atx style header
bad.md: 3: MD010 Hard tabs
bad.md: 1: MD018 No space after hash on atx style header
bad.md: 3: MD018 No space after hash on atx style header
Or invoke markdownlint.sync
for a synchronous call:
var result = markdownlint.sync(options);
console.log(result.toString(true));
Output:
bad.string: 3: no-hard-tabs Hard tabs
bad.string: 1: no-missing-space-atx No space after hash on atx style header
bad.string: 3: no-missing-space-atx No space after hash on atx style header
bad.md: 3: no-hard-tabs Hard tabs
bad.md: 1: no-missing-space-atx No space after hash on atx style header
bad.md: 3: no-missing-space-atx No space after hash on atx style header
To examine the result
object directly:
markdownlint(options, function callback(err, result) {
if (!err) {
console.dir(result, { "colors": true });
}
});
Output:
{
"good.string": {},
"bad.string": {
"MD010": [ 3 ],
"MD018": [ 1, 3 ]
},
"good.md": {},
"bad.md": {
"MD010": [ 3 ],
"MD018": [ 1, 3 ]
}
}
Integration with the gulp build system is straightforward:
var gulp = require("gulp");
var through2 = require("through2");
var markdownlint = require("markdownlint");
gulp.task("markdownlint", function task() {
return gulp.src("*.md", { "read": false })
.pipe(through2.obj(function obj(file, enc, next) {
markdownlint(
{ "files": [ file.relative ] },
function callback(err, result) {
var resultString = (result || "").toString();
if (resultString) {
console.log(resultString);
}
next(err, file);
});
}));
});
Output:
[00:00:00] Starting 'markdownlint'...
bad.md: 3: MD010 Hard tabs
bad.md: 1: MD018 No space after hash on atx style header
bad.md: 3: MD018 No space after hash on atx style header
[00:00:00] Finished 'markdownlint' after 10 ms
Integration with the Grunt build system is similar:
var markdownlint = require("markdownlint");
module.exports = function wrapper(grunt) {
grunt.initConfig({
"markdownlint": {
"example": {
"src": [ "*.md" ]
}
}
});
grunt.registerMultiTask("markdownlint", function task() {
var done = this.async();
markdownlint(
{ "files": this.filesSrc },
function callback(err, result) {
var resultString = err || ((result || "").toString());
if (resultString) {
grunt.fail.warn("\n" + resultString + "\n");
}
done(!err || !resultString);
});
});
};
Output:
Running "markdownlint:example" (markdownlint) task
Warning:
bad.md: 3: MD010 Hard tabs
bad.md: 1: MD018 No space after hash on atx style header
bad.md: 3: MD018 No space after hash on atx style header
Use --force to continue.
markdownlint
also works in the browser.
Generate normal and minified scripts with:
npm run build-demo
Then reference markdown-it
and markdownlint
:
<script src="demo/markdown-it.min.js"></script>
<script src="demo/markdownlint-browser.min.js"></script>
And call it like so:
var options = {
"strings": {
"content": "Some Markdown to lint."
}
};
var results = window.markdownlint.sync(options).toString();
strings
option to enable file-less scenarios, add in-browser demo.0.1.0
FAQs
A Node.js style checker and lint tool for Markdown/CommonMark files.
The npm package markdownlint receives a total of 487,239 weekly downloads. As such, markdownlint popularity was classified as popular.
We found that markdownlint demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.