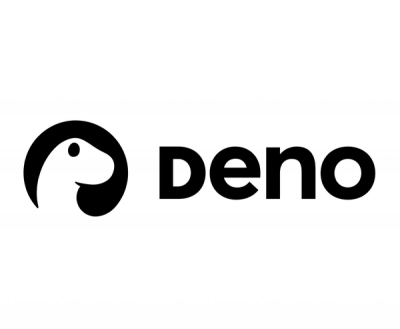
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
markup-it
is a JavaScript library to parse and modify markuped content (for example Markdown) using an intermediate format backed by an immutable model.
$ npm i markup-it --save
Initialize a syntax:
var MarkupIt = require('markup-it');
var markdownSyntax = require('markup-it/syntaxes/markdown');
var htmlSyntax = require('markup-it/syntaxes/html');
var markdown = new MarkupIt(markdownSyntax);
var html = new MarkupIt(htmlSyntax);
var content = markdown.toContent('Hello **World**');
// Render back to markdown:
var textMd = markdown.toText(content);
// Render to HTML
var textHtml = html.toText(content);
var content = html.toContent('Hello <b>World</b>');
var textMd = markdown.toText(content);
const Slate = require('slate');
var rawJson = MarkupIt.SlateUtils.encode(content);
var state = Slate.Raw.deserialize(rawJson);
And output markdown from a State using SlateUtils.decode
:
var rawJson = Slate.Raw.serialize(state);
var content = MarkupIt.SlateUtils.decode(rawJson);
var text = markdown.toText(content);
This module contains the markdown syntax, but you can write your custom syntax or extend the existing ones.
var myRule = MarkupIt.Rule(DraftMarkup.BLOCKS.HEADING_1)
.regExp(/^<h1>(\S+)<\/h1>/, function(state, match) {
return {
tokens: state.parseAsInline(match[1])
};
})
.toText(function(state, token) {
return '<h1>' + state.renderAsInline(token) + '</h1>';
});
Create a new syntax inherited from the markdown one:
var mySyntax = markdownSyntax.addBlockRules(myRule);
Checkout the GitBook syntax as an example of custom rules extending a syntax.
FAQs
Pipeline for working with markup input (for example Markdown)
The npm package markup-it receives a total of 420 weekly downloads. As such, markup-it popularity was classified as not popular.
We found that markup-it demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.