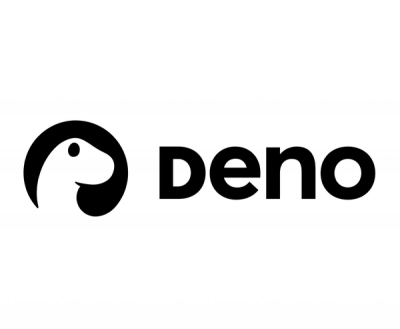
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
material-ui-confirm
Advanced tools
Higher order component for straightforward use of @material-ui/core confirmation dialogs.
Higher order component for straightforward use of @material-ui/core confirmation dialogs.
npm install --save material-ui-confirm
import React from 'react';
import Button from '@material-ui/core/Button';
import withConfirm from 'material-ui-confirm';
const Item = ({ confirm }) => {
const handleDelete = () => { /* ... */ };
return (
<Button onClick={confirm(handleDelete, { description: 'This action is permanent!' })}>
Click
</Button>
);
};
export default withConfirm(Item);
withConfirm(Component)
Returns Component adding the confirm
function to its props.
confirm(onConfirm, options)
Returns a function that opens the confirmation dialog once called.
If the user confirms the action, the onConfirm
callback is fired.
Name | Type | Default | Description |
---|---|---|---|
title | string | 'Are you sure?' | Dialog title. |
description | string | '' | Dialog content. |
confirmationText | string | 'Ok' | Confirmation button caption. |
cancellationText | string | 'Cancel' | Cancellation button caption. |
dialogProps | object | {} | Material-UI Dialog props. |
onClose | function | () => {} | Callback fired before the dialog closes. |
onCancel | function | () => {} | Callback fired when the user cancels the action. |
FAQs
Simple confirmation dialogs built on top of @mui/material
We found that material-ui-confirm demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.