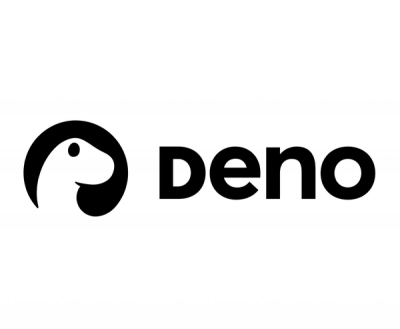
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
matrix-bot-sdk
Advanced tools
A lightweight version of the matrix-js-sdk intended for bots. For help and support, visit #matrix-bot-sdk:t2bot.io
This package can be found on npm:
npm install matrix-bot-sdk
const MatrixClient = require("matrix-bot-sdk").MatrixClient;
const AutojoinRoomsMixin = require("matrix-bot-sdk").AutojoinRoomsMixin;
const client = new MatrixClient("https://matrix.org", "your_access_token_here");
AutojoinRoomsMixin.setupOnClient(client);
client.on("room.message", (event) => {
if (!event["content"]) return;
console.log(event["sender"] + " says " + event["content"]["body"]);
client.sendMessage(event["room_id"], {
"msgtype": "m.notice",
"body": "hello!",
});
// or...
client.sendNotice(event["room_id"], "hello!");
});
client.start().then(() => console.log("Client started!"));
To automatically process rich replies coming into the client:
const MatrixClient = require("matrix-bot-sdk").MatrixClient;
const RichRepliesPreprocessor = require("matrix-bot-sdk").RichRepliesPreprocessor;
const IRichReplyMetadata = require("matrix-bot-sdk").IRichReplyMetadata;
const client = new MatrixClient("https://matrix.org", "your_access_token_here");
// Set fetchRealEventContents to true to have the preprocessor get the real event
client.addPreprocessor(new RichRepliesPreprocessor(fetchRealEventContents: false));
// regular client usage here. When you encounter an event:
const event = {/* from somewhere, such as on a room message */};
if (event["mx_richreply"]) {
const reply = <IRichReplyMetadata>event["mx_richreply"];
console.log("The original event was " + reply.parentEventId + " and the text was " + reply.fallbackPlainBody);
}
To send a rich reply to an event:
const MatrixClient = require("matrix-bot-sdk").MatrixClient;
const AutojoinRoomsMixin = require("matrix-bot-sdk").AutojoinRoomsMixin;
const RichReply = require("matrix-bot-sdk").RichReply;
const client = new MatrixClient("https://matrix.org", "your_access_token_here");
AutojoinRoomsMixin.setupOnClient(client);
client.on("room.message", (event) => {
if (!event["content"]) return;
const newEvent = RichReply.createFor(event, "Hello!", "<b>Hello!</b>");
newEvent["msgtype"] = "m.notice";
client.sendMessage(event["room_id"], newEvent);
});
client.start().then(() => console.log("Client started!"));
FAQs
TypeScript/JavaScript SDK for Matrix bots and appservices
We found that matrix-bot-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.