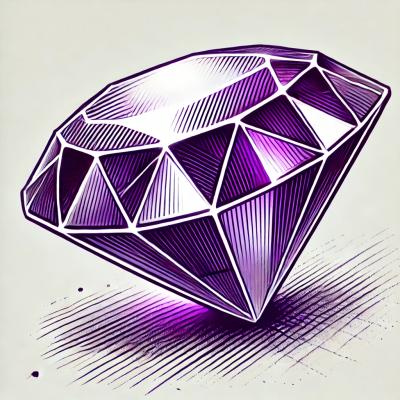
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
mcl ; A portable and fast pairing-based cryptography library for Node.js by WebAssembly
The mcl-wasm package is a WebAssembly (WASM) implementation of the MCL (Multiprecision Complex Library) which provides cryptographic functionalities, particularly focusing on pairing-based cryptography. It is designed to be used in web applications and Node.js environments.
Pairing-based Cryptography
This code demonstrates the use of pairing-based cryptography with the mcl-wasm package. It initializes the BLS12-381 curve, generates random elements in the field, computes points on the elliptic curve, and then performs a pairing operation.
const mcl = require('mcl-wasm');
(async () => {
await mcl.init(mcl.BLS12_381);
const a = new mcl.Fr();
a.setByCSPRNG();
const b = new mcl.Fr();
b.setByCSPRNG();
const P = mcl.mul(mcl.g1(), a);
const Q = mcl.mul(mcl.g2(), b);
const ePQ = mcl.pairing(P, Q);
console.log('e(P, Q) =', ePQ.getStr());
})();
Elliptic Curve Operations
This code demonstrates basic elliptic curve operations such as point addition. It initializes the BLS12-381 curve, creates points on the curve, and adds them together.
const mcl = require('mcl-wasm');
(async () => {
await mcl.init(mcl.BLS12_381);
const P = mcl.g1();
const Q = mcl.g1();
Q.setStr('1 2');
const R = mcl.add(P, Q);
console.log('P + Q =', R.getStr());
})();
Field Arithmetic
This code demonstrates field arithmetic operations such as addition. It initializes the BLS12-381 curve, generates random field elements, and adds them together.
const mcl = require('mcl-wasm');
(async () => {
await mcl.init(mcl.BLS12_381);
const a = new mcl.Fr();
a.setByCSPRNG();
const b = new mcl.Fr();
b.setByCSPRNG();
const c = mcl.add(a, b);
console.log('a + b =', c.getStr());
})();
The elliptic package is a JavaScript library for elliptic curve cryptography. It provides a wide range of elliptic curve operations and is widely used in various cryptographic applications. Compared to mcl-wasm, elliptic does not focus on pairing-based cryptography but offers a broader range of elliptic curve algorithms.
The noble-bls12-381 package is a JavaScript implementation of the BLS12-381 curve, which is used for pairing-based cryptography. It is similar to mcl-wasm in that it focuses on the BLS12-381 curve, but it is implemented purely in JavaScript without relying on WebAssembly.
The bls-signatures package provides an implementation of BLS (Boneh-Lynn-Shacham) signatures, which are based on pairing-based cryptography. It is similar to mcl-wasm in that it focuses on BLS signatures, but it is more specialized and does not provide the broader range of cryptographic functionalities that mcl-wasm offers.
see mcl
{G1,G2}::isValidOrder()
mulVec(xVec, yVec)
where xVec is an array of G1 or G2 and yVec is an array of Fr, which returns sum of xVec[i] yVec[i]
.The version v0.6.0
breaks backward compatibility of the entry point.
const mcl = require('mcl-wasm')
const mcl = require('mcl-wasm')
<script src="https://herumi.github.io/mcl-wasm/browser/mcl.js"></script>
node test/test.js
// Ethereum 2.0 spec mode
mcl.init(mcl.BLS12_381)
.then(() => {
mcl.setETHserialization(true) // Ethereum serialization
mcl.setMapToMode(mcl.IRTF) // for G2.setHashOf(msg)
...
})
a = new mcl.Fr()
a.setStr('255') // set 255
a.setStr('0xff') // set 0xff = 255
a.setStr('ff', 16) // set ff as hex-string
a.getStr() // '255'
a.getStr(16) // 'ff'
// byte array serialization
b.deserialize(a.serialize()) // b.isEqualTo(a)
// hex string of serialization()
b.deserializeHexStr(a.serializeToHexStr())
// serialization like Ethereum 2.0 only for BLS12-381
mcl.setETHserialization(true)
/*
it is big cost to to verify the order
call once after init() if you want to disable it
cf. sub group problem
*/
mcl.verifyOrderG1(false)
mcl.verifyOrderG2(false)
see test.js
modified new BSD License http://opensource.org/licenses/BSD-3-Clause
2019/Jan/31 add Fp.mapToG1
MITSUNARI Shigeo(herumi@nifty.com)
FAQs
mcl ; A portable and fast pairing-based cryptography library for Node.js by WebAssembly
We found that mcl-wasm demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.