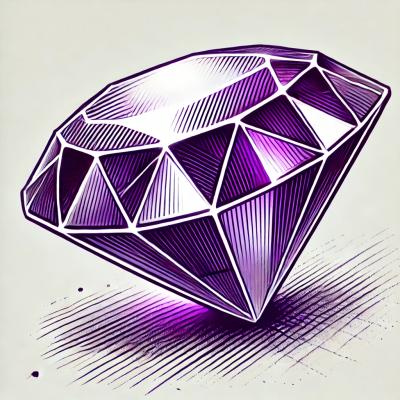
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
mdast-util-to-hast
Advanced tools
The mdast-util-to-hast package is a utility that allows for the transformation of MDAST (Markdown Abstract Syntax Tree) to HAST (Hypertext Abstract Syntax Tree). This is particularly useful for applications that need to convert markdown content into HTML or other formats that can be more easily manipulated or displayed in web environments.
Convert MDAST to HAST
This feature allows for the conversion of a markdown document represented as an MDAST into a HAST. The code sample demonstrates how to use unified with the remark-parse plugin to parse markdown into MDAST, and then convert that MDAST into HAST using mdast-util-to-hast.
const unified = require('unified')
const markdown = require('remark-parse')
const toHAST = require('mdast-util-to-hast')
unified()
.use(markdown)
.use(() => tree => toHAST(tree))
.process('# Hello world!', function (err, file) {
if (err) throw err
console.log(file)
})
remark-html is a plugin for the remark processor that compiles markdown to HTML. It is similar to mdast-util-to-hast in that it also deals with the conversion of markdown content to a more web-friendly format. However, remark-html is more of an end-to-end solution for converting markdown directly to HTML, whereas mdast-util-to-hast provides a lower-level conversion to HAST, which can then be further manipulated or converted to HTML.
rehype is a processor powered by plugins part of the unified ecosystem that manipulates HTML documents. It is similar to mdast-util-to-hast in the sense that both deal with HAST nodes. However, rehype operates directly on HTML content or HAST, focusing on the manipulation and processing of HTML, whereas mdast-util-to-hast is specifically designed for converting MDAST to HAST.
mdast utility to transform to hast.
Note: You probably want to use
remark-rehype
.
npm:
npm install mdast-util-to-hast
npm with TypeScript support:
npm install mdast-util-to-hast @types/mdast
Say we have the following example.md
:
## Hello **World**!
…and next to it, example.js
:
var inspect = require('unist-util-inspect')
var unified = require('unified')
var parse = require('remark-parse')
var vfile = require('to-vfile')
var toHast = require('mdast-util-to-hast')
var tree = unified()
.use(parse)
.parse(vfile.readSync('example.md'))
console.log(inspect(toHast(tree)))
Which when running with node example
yields:
root[1] (1:1-2:1, 0-20)
└─ element[3] (1:1-1:20, 0-19) [tagName="h2"]
├─ text: "Hello " (1:4-1:10, 3-9)
├─ element[1] (1:10-1:19, 9-18) [tagName="strong"]
│ └─ text: "World" (1:12-1:17, 11-16)
└─ text: "!" (1:19-1:20, 18-19)
toHast(node[, options])
Transform the given mdast tree to a hast tree.
options.allowDangerousHtml
Whether to allow html
nodes and inject them as raw HTML
(boolean
, default: false
).
Only do this when using hast-util-to-html
(rehype-stringify
) or hast-util-raw
(rehype-raw
) later: raw
nodes are not a standard part of
hast.
options.commonmark
Set to true
(default: false
) to prefer the first when duplicate definitions
are found.
The default behavior is to prefer the last duplicate definition.
options.handlers
Object mapping mdast nodes to functions handling them.
Take a look at lib/handlers/
for examples.
options.unknownHandler
Handler for all unknown nodes.
Default behavior:
children
are transformed to div
elementsvalue
are transformed to text
nodesyaml
and toml
nodes are ignored (created by
remark-frontmatter
)html
nodes are ignored if allowDangerousHtml
is false
position
s are properly patchednode.data.hName
configures the hast element’s tag-namenode.data.hProperties
is mixed into the hast element’s
propertiesnode.data.hChildren
configures the hast element’s childrenhName
node.data.hName
sets the tag-name of an element.
The following mdast:
{
type: 'strong',
data: {hName: 'b'},
children: [{type: 'text', value: 'Alpha'}]
}
Yields, in hast:
{
type: 'element',
tagName: 'b',
properties: {},
children: [{type: 'text', value: 'Alpha'}]
}
hProperties
node.data.hProperties
in sets the properties of an element.
The following mdast:
{
type: 'image',
src: 'circle.svg',
alt: 'Big red circle on a black background',
title: null
data: {hProperties: {className: ['responsive']}}
}
Yields, in hast:
{
type: 'element',
tagName: 'img',
properties: {
src: 'circle.svg',
alt: 'Big red circle on a black background',
className: ['responsive']
},
children: []
}
hChildren
node.data.hChildren
sets the children of an element.
The following mdast:
{
type: 'code',
lang: 'js',
data: {
hChildren: [
{
type: 'element',
tagName: 'span',
properties: {className: ['hljs-meta']},
children: [{type: 'text', value: '"use strict"'}]
},
{type: 'text', value: ';'}
]
},
value: '"use strict";'
}
Yields, in hast (note: the pre
and language-js
class are normal
mdast-util-to-hast
functionality):
{
type: 'element',
tagName: 'pre',
properties: {},
children: [{
type: 'element',
tagName: 'code',
properties: {className: ['language-js']},
children: [
{
type: 'element',
tagName: 'span',
properties: {className: ['hljs-meta']},
children: [{type: 'text', value: '"use strict"'}]
},
{type: 'text', value: ';'}
]
}]
}
Use of mdast-util-to-hast
can open you up to a
cross-site scripting (XSS) attack.
Embedded hast properties (hName
, hProperties
, hChildren
), custom handlers,
and the allowDangerousHtml
option all provide openings.
The following example shows how a script is injected where a benign code block is expected with embedded hast properties:
var code = {type: 'code', value: 'alert(1)'}
code.data = {hName: 'script'}
Yields:
<script>alert(1)</script>
The following example shows how an image is changed to fail loading and therefore run code in a browser.
var image = {type: 'image', url: 'existing.png'}
image.data = {hProperties: {src: 'missing', onError: 'alert(2)'}}
Yields:
<img src="missing" onerror="alert(2)">
The following example shows the default handling of embedded HTML:
# Hello
<script>alert(3)</script>
Yields:
<h1>Hello</h1>
Passing allowDangerousHtml: true
to mdast-util-to-hast
is typically still
not enough to run unsafe code:
<h1>Hello</h1>
<script>alert(3)</script>
If allowDangerousHtml: true
is also given to hast-util-to-html
(or
rehype-stringify
), the unsafe code runs:
<h1>Hello</h1>
<script>alert(3)</script>
Use hast-util-sanitize
to make the hast tree safe.
mdast-util-to-nlcst
— transform mdast to nlcsthast-util-to-mdast
— transform hast to mdasthast-util-to-xast
— transform hast to xasthast-util-sanitize
— sanitize hast nodesremark-rehype
— rehype support for remarkrehype-remark
— remark support for rehypeSee contributing.md
in syntax-tree/.github
for ways to get
started.
See support.md
for ways to get help.
This project has a code of conduct. By interacting with this repository, organization, or community you agree to abide by its terms.
FAQs
mdast utility to transform to hast
The npm package mdast-util-to-hast receives a total of 5,162,399 weekly downloads. As such, mdast-util-to-hast popularity was classified as popular.
We found that mdast-util-to-hast demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.