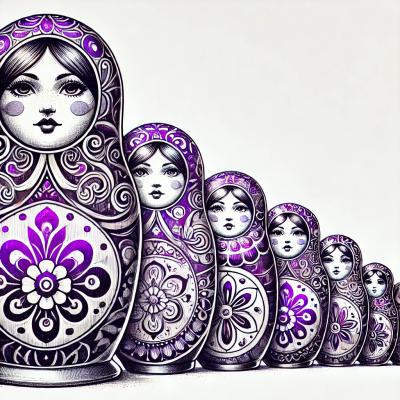
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
mem-fs-editor
Advanced tools
The mem-fs-editor package is a utility for working with the file system in memory. It provides a way to perform file operations such as reading, writing, and modifying files without directly interacting with the disk until you decide to commit the changes. This is particularly useful for scaffolding tools and generators.
Reading Files
This feature allows you to read the content of a file from the file system into memory. The code sample demonstrates how to read a file using mem-fs-editor.
const memFs = require('mem-fs');
const editor = require('mem-fs-editor');
const store = memFs.create();
const fs = editor.create(store);
const content = fs.read('path/to/file.txt');
console.log(content);
Writing Files
This feature allows you to write content to a file in memory and then commit the changes to the disk. The code sample demonstrates how to write to a file and commit the changes.
const memFs = require('mem-fs');
const editor = require('mem-fs-editor');
const store = memFs.create();
const fs = editor.create(store);
fs.write('path/to/file.txt', 'Hello, World!');
fs.commit(() => {
console.log('File written to disk');
});
Modifying Files
This feature allows you to modify files in memory, such as copying or moving files. The code sample demonstrates how to copy a file from one location to another and commit the changes.
const memFs = require('mem-fs');
const editor = require('mem-fs-editor');
const store = memFs.create();
const fs = editor.create(store);
fs.copy('path/to/source.txt', 'path/to/destination.txt');
fs.commit(() => {
console.log('File copied');
});
fs-extra is a package that extends the native Node.js fs module with additional methods for working with the file system. It provides similar functionalities such as reading, writing, and copying files, but it operates directly on the disk rather than in memory. This makes it less suitable for scenarios where you want to batch file operations before committing them.
vinyl-fs is a file system utility for working with streams in Gulp. It allows you to read, write, and modify files using a stream-based approach. While it provides similar functionalities to mem-fs-editor, it is more focused on stream processing and is commonly used in build systems.
graceful-fs is a drop-in replacement for the native fs module that improves handling of file system operations, especially in environments with high concurrency. It provides similar file operation methods but focuses on enhancing the reliability and performance of file system interactions.
File edition helpers working on top of mem-fs
var memFs = require('mem-fs');
var editor = require('mem-fs-editor');
var store = memFs.create();
var fs = editor.create(store);
fs.write('somefile.js', 'var a = 1;');
#read(filepath, [options])
Read a file and return its contents as a string.
You can alternatively get the raw contents buffer if you pass options.raw = true
.
#readJSON(filepath)
Read a file and parse its contents as JSON.
#write(filepath, contents)
Replace the content of a file (existing or new) with a string or a buffer.
#delete(filepath)
Delete a file or a directory.
#copy(from, to, [options])
Copy a file from the from
path to the to
path.
Optionally, pass an options.process
function (process(contents)
) returning a string or a buffer who'll become the new file content. The process function will take a single contents argument who is the copied file contents as a Buffer
.
from
can be a glob pattern that'll be match against the file system. If that's the case, then to
must be an output directory.
#copyTpl(from, to, context, [settings])
Copy the from
file and parse its content as an underscore template where context
is the template context.
Optionnally pass a template settings
object.
#commit([filters,] callback)
Persist every changes made to files in the mem-fs store to disk.
If provided, filters
is an array of TransformStream to be applied on a stream of vinyl files (like gulp plugins).
callback
is called once the files are updated on disk.
FAQs
File edition helpers working on top of mem-fs
The npm package mem-fs-editor receives a total of 1,382,886 weekly downloads. As such, mem-fs-editor popularity was classified as popular.
We found that mem-fs-editor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.