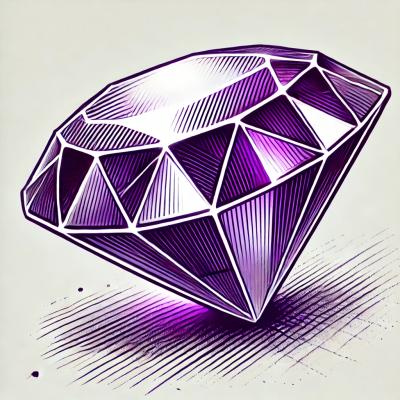
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Memoize functions - An optimization used to speed up consecutive function calls by caching the result of calls with identical input
The 'mem' npm package is a utility for memoizing functions, which means it caches the result of function calls based on the arguments provided. This can significantly improve performance for expensive or frequently called functions by avoiding redundant computations.
Basic Memoization
This feature allows you to memoize a function so that it caches the result of function calls based on the arguments. Subsequent calls with the same arguments will return the cached result instead of recalculating.
const mem = require('mem');
const expensiveFunction = (input) => {
console.log('Function called with', input);
return input * 2;
};
const memoizedFunction = mem(expensiveFunction);
console.log(memoizedFunction(2)); // Function called with 2, 4
console.log(memoizedFunction(2)); // 4 (cached result)
Custom Cache Key
This feature allows you to define a custom cache key function, which determines how the cache key is generated based on the function arguments. This can be useful for more complex caching strategies.
const mem = require('mem');
const expensiveFunction = (input) => {
console.log('Function called with', input);
return input * 2;
};
const customCacheKey = (args) => args[0] % 2; // Cache based on even/odd
const memoizedFunction = mem(expensiveFunction, { cacheKey: customCacheKey });
console.log(memoizedFunction(2)); // Function called with 2, 4
console.log(memoizedFunction(4)); // 4 (cached result)
console.log(memoizedFunction(3)); // Function called with 3, 6
Cache Expiration
This feature allows you to set a maximum age for cache entries. After the specified time, the cache entry will expire, and the function will be called again to recalculate the result.
const mem = require('mem');
const expensiveFunction = (input) => {
console.log('Function called with', input);
return input * 2;
};
const memoizedFunction = mem(expensiveFunction, { maxAge: 1000 });
console.log(memoizedFunction(2)); // Function called with 2, 4
setTimeout(() => {
console.log(memoizedFunction(2)); // Function called with 2, 4 (after 1 second, cache expired)
}, 1500);
Lodash's memoize function provides similar functionality to 'mem' by caching the result of function calls. It is part of the larger Lodash utility library, which offers a wide range of utility functions for JavaScript. Compared to 'mem', lodash.memoize is more lightweight but lacks some advanced features like cache expiration.
Memoizee is a full-featured memoization library that offers a wide range of options, including cache expiration, custom cache keys, and more. It is more feature-rich compared to 'mem' but also comes with a larger footprint.
Moize is another memoization library that offers a balance between performance and features. It supports cache expiration, custom cache keys, and other advanced options. It is similar to 'mem' in terms of functionality but aims to provide better performance and more configuration options.
Memoize functions - An optimization used to speed up consecutive function calls by caching the result of calls with identical input
Requires at least Node.js 0.12, unless you provide your own cache
store.
$ npm install --save mem
const mem = require('mem');
let i = 0;
const counter = () => ++i;
const memoized = mem(counter);
memoized('foo');
//=> 1
// cached as it's the same arguments
memoized('foo');
//=> 1
// not cached anymore as the arguments changed
memoized('bar');
//=> 2
memoized('bar');
//=> 2
const mem = require('mem');
let i = 0;
const counter = () => Promise.resolve(++i);
const memoized = mem(counter);
memoized().then(a => {
console.log(a);
//=> 1
memoized().then(b => {
// the return value didn't increase as it's cached
console.log(b);
//=> 1
});
});
const mem = require('mem');
const got = require('got');
const memGot = mem(got, {maxAge: 1000});
memGot('sindresorhus.com').then(() => {
// this call is cached
memGot('sindresorhus.com').then(() => {
setTimeout(() => {
// this call is not cached as the cache has expired
memGot('sindresorhus.com').then(() => {});
}, 2000);
});
});
Type: function
Function to be memoized.
Type: number
Default: Infinity
Milliseconds until the cache expires.
Type: function
Determines the cache key for storing the result based on the function arguments. By default, if there's only one argument and it's a primitive it's used directly as a key, otherwise it's all the function arguments JSON stringified as an array.
You could for example change it to only cache on the first argument x => JSON.stringify(x)
.
Type: object
Default: new Map()
Use a different cache storage. Must implement the following methods: .has(key)
, .get(key)
, .set(key, value)
. You could for example use a WeakMap
instead.
MIT © Sindre Sorhus
FAQs
Memoize functions - An optimization used to speed up consecutive function calls by caching the result of calls with identical input
The npm package mem receives a total of 3,365,217 weekly downloads. As such, mem popularity was classified as popular.
We found that mem demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.