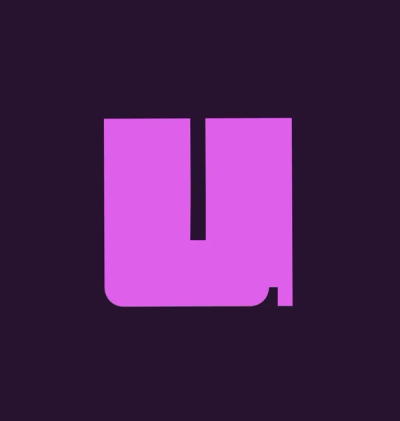
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
memcache-client-memoizer
Advanced tools
A function memoizer using a get/set cache client.
Install:
npm i memcache-client-memoizer
memoizer(options)
options
: object
. Required. An object with the following keys:
client
: { get: (anything) => Promise, set: (anything, value, options) }
. A cache client instance, must have a get
and set
method. The get
method must return a promise.clientProviderFn
: () => client
A function which returns a client
(defined above);
(Either a client
or clientProviderFn
must be passed.)fn
: Function
. Required. The function to memoize, must return a Promise.keyFn
: (args to fn) => anything
. Required. A function which returns a cache-key (can be anything) for caching. This
function is called with the same arguments as fn
, allowing you to create a dynamic cache-key, for example:
const exampleKeyFn = ({ name, color }) => `${name}:${color}` // can be anything
setOptions
: anything
. Optional. For memcached-client
this can be
command options.cacheResultTransformFn
. (result-from-cache) => transformed-result
. Function to transform cache-result, defaults
to (x) => x
. This is useful if your cache service sends along the value in a different form than is returned by your fn
.skipCacheFn
: (args to fn) => Boolean
. Optional. A function which indicates that the call to fn
should skip the
cache.Rejected promises are not memoized - since that's probably not what you want :)
const MemcacheClient = require('memcache-client')
const { memoizer } = require('memcache-client-memoizer')
const fnToMemoize = ({ name, color }) => Promise.resolve({ name, color })
const memoizedFn = memoizer({
clientProviderFn: () => new MemcacheClient({ server: 'localhost:11211' }),
fn: fnToMemoize,
keyFn: ({ name, color }) => `${name}:${color}`, // this can return anything
cacheResultTransformFn: ({value}) => value,
skipCacheFn: ({ name, color }) => false,
})
memoizedFn({name: 'Max', color: 'blue'})
.then((result) => { ... }) // cache miss, fill cache, returns {name: 'Max', color: 'blue'}
// later on...
memoizedFn({name: 'Max', color: 'blue'})
.then((result) => { ... }) // cache hit, returns {name: 'Max', color: 'blue'}
const Catbox = require('catbox');
const Memory = require('catbox-memory');
const cacheTtlMilliseconds = 1000 * 60 * 5; // 5 min
const client = new Catbox.Client(Memory);
await client.start();
const fnToMemoize = ({ name, color }) => Promise.resolve({ name, color })
const memoizedFn = memoizer({
client,
fn: fnToMemoize,
keyFn: ({ name, color }) => ({ segment: 'test', id: 'test-cache' }), // this can return anything
setOptions: cacheTtlMilliseconds,
cacheResultTransformFn: ({ item }) => item,
skipCacheFn: ({ name, color }) => false,
})
memoizedFn({name: 'Max', color: 'blue'})
.then((result) => { ... }) // cache miss, fill cache, returns {name: 'Max', color: 'blue'}
// later on...
memoizedFn({name: 'Max', color: 'blue'})
.then((result) => { ... }) // cache hit, returns {name: 'Max', color: 'blue'}
FAQs
Memoizes promise-returning functions via memcache-client
The npm package memcache-client-memoizer receives a total of 6 weekly downloads. As such, memcache-client-memoizer popularity was classified as not popular.
We found that memcache-client-memoizer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.