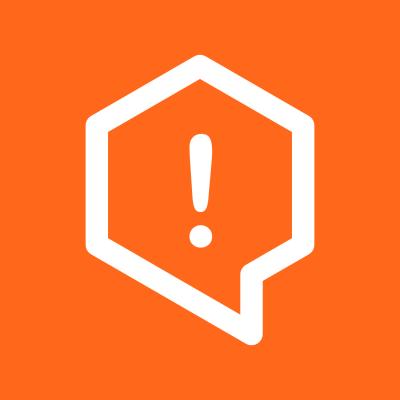
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
memlab is a framework that analyzes memory and finds memory leaks in JavaScript applications.
memlab is an E2E testing and analysis framework for finding JavaScript memory leaks in Chromium. The CLI Toolbox and library provide extensible interfaces for analyzing heap snapshots taken from Chrome/Chromium, Node.js, Hermes, and Electron.js.
Install the CLI
npm install -g memlab
To find memory leaks in Google Maps, you can create a scenario file defining how
to interact with the Google Maps, let's name it test-google-maps.js
:
// Visit Google Maps
function url() {
return 'https://www.google.com/maps/@37.386427,-122.0428214,11z';
}
// action where we want to detect memory leaks: click the Hotels button
async function action(page) {
await page.click('button[aria-label="Hotels"]');
}
// action where we want to go back to the step before: click clear search
async function back(page) {
await page.click('[aria-label="Clear search"]');
}
module.exports = {action, back, url};
Now run memlab with the scenario file, memlab will interact with the web page and show detected memory leaks:
memlab run --scenario test-google-maps.js
View which object keeps growing in size during interaction in the previous run:
memlab analyze unbound-object
Analyze pre-fetched v8/hermes .heapsnapshot
files:
memlab analyze unbound-object --snapshot-dir <DIR_OF_SNAPSHOT_FILES>
Use memlab analyze
to view all built-in memory analyses. For extension, view the doc site.
View retainer trace of a particular object:
memlab report --nodeId <HEAP_OBJECT_ID>
Use memlab help
to view all CLI commands.
Use the memlab
package to start a E2E run in browser and detect memory leaks:
const memlab = require('memlab');
const scenario = {
// initial page load url
url: () => 'https://www.google.com/maps/@37.386427,-122.0428214,11z',
// action where we want to detect memory leaks
action: async (page) => await page.click('button[aria-label="Hotels"]'),
// action where we want to go back to the step before
back: async (page) => await page.click('[aria-label="Clear search"]'),
}
memlab.run({scenario});
FAQs
memlab is a framework that analyzes memory and finds memory leaks in JavaScript applications.
The npm package memlab receives a total of 64,905 weekly downloads. As such, memlab popularity was classified as popular.
We found that memlab demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.