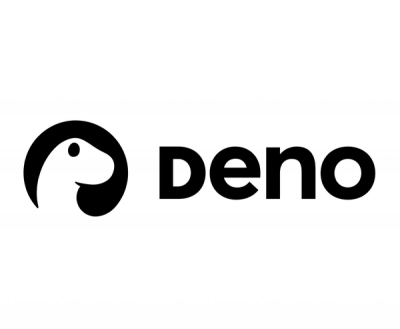
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
mendixplatformsdk
Advanced tools
The Mendix Platform SDK can be used to call Mendix Platform APIs. It also integrates with the Mendix Model SDK for working on temporary online working copies of Mendix projects.
At the moment, the Platform SDK implements the following functionality:
To use the Mendix Platform SDK, you need a Personal Access Token with the right scopes. Go to Warden to create a Personal Access Token.
Warning: Do not put the Personal Access Token inside your source code! Instead, pass it to your script using an environment variable. The Platform SDK automatically tries to get the Personal Access Token from the environment variable MENDIX_TOKEN.
TODO
npm install mendixplatformsdk mendixmodelsdk
.
Here is a small example script that shows the main APIs of the Platform SDK.
import { MendixPlatformClient } from "mendixplatformsdk";
async function main() {
// Create a Platform SDK client. This will automatically read
// your Personal Access Token from the environment variable MENDIX_TOKEN.
const client = new MendixPlatformClient();
// Create a new app in the Mendix Developer Portal. This will also create
// a new Team Server repository based on the Blank App template.
//
// If you want to use an existing app, you can use the following:
// const app = client.getApp("existing-app-id");
const app = await client.createNewApp("My First App");
// Create a temporary working copy on the Model Server based on the main
// line of the Team Server repository.
//
// If you want to use an existing working copy, you can use the following:
// const workingCopy = app.getOnlineWorkingCopy("existing-working-copy-id");
const workingCopy = await app.createTemporaryWorkingCopy("main");
// Open the working copy using the Model SDK.
const model = await workingCopy.openModel();
// Show the names of all the modules in the app model.
console.log("All modules in the model:");
for (const module of model.allModules()) {
console.log(` * ${module.name}`);
}
// Rename the first module.
model.allModules()[0].name = "RenamedModule";
await model.flushChanges();
// Commit the changes back to the Team Server.
await workingCopy.commitToTeamServer();
// Delete the app.
await app.delete();
}
// Run the 'main' function and report any errors.
main().catch(error => {
console.log("ERROR: An error occurred.", error);
process.exit(1);
})
FAQs
Mendix Platform SDK
The npm package mendixplatformsdk receives a total of 66 weekly downloads. As such, mendixplatformsdk popularity was classified as not popular.
We found that mendixplatformsdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.