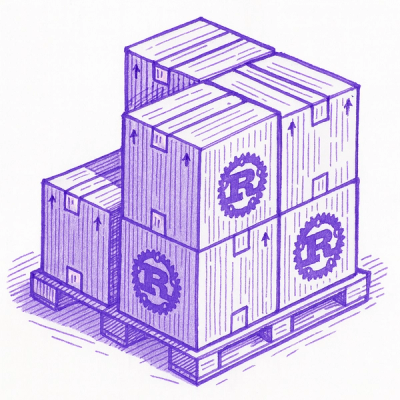
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
messaging-api-messenger
Advanced tools
Messaging API client for Messenger
npm i --save messaging-api-messenger
or
yarn add messaging-api-messenger
const { MessengerClient } = require('messaging-api-messenger');
// get accessToken from facebook developers website
const client = MessengerClient.connect(accessToken);
You can specify version of Facebook Graph API using second argument:
const client = MessengerClient.connect(accessToken, '2.9');
If it is not specified, version 2.11
will be used as default.
All methods return a Promise.
sendRawBody(body)
Send request raw body using the Send API.
Param | Type | Description |
---|---|---|
body | Object | Raw body to be sent. |
Example:
client.sendRawBody({
recipient: {
id: USER_ID,
},
message: {
text: 'Hello!',
},
});
sendMessage(userId, message [, options])
Send messages to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
message | Object | message object. |
options | Object | Other optional parameters. For example, messaging types or tags. |
Example:
client.sendMessage(USER_ID, {
text: 'Hello!',
});
You can specifiy messaging type using options. If messaging_type
and tag
is not provided, UPDATE
will be used as default messaging type.
Example:
client.sendMessage(
USER_ID,
{
text: 'Hello!',
},
{
messaging_type: 'RESPONSE',
}
);
Available messaging types:
UPDATE
as defaultRESPONSE
using { messaging_type: 'RESPONSE' }
optionsMESSAGE_TAG
using { tag: 'ANY_TAG' }
optionsNON_PROMOTIONAL_SUBSCRIPTION
using { messaging_type: 'NON_PROMOTIONAL_SUBSCRIPTION' }
optionssendText(userId, text [, options])
Send plain text messages to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
text | String | Text of the message to be sent. |
options | Object | Other optional parameters. For example, messaging types or tags. |
Example:
client.sendText(USER_ID, 'Hello!', { tag: 'ISSUE_RESOLUTION' });
sendAttachment(userId, attachment [, options])
Send attachment messages to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
attachment | Object | attachment object. |
options | Object | Other optional parameters. For example, messaging types or tags. |
Example:
client.sendAttachment(USER_ID, {
type: 'image',
payload: {
url: 'https://example.com/pic.png',
},
});
sendAudio(userId, audio [, options])
Send sounds to specified user by uploading them or sharing a URL using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
audio | String | Buffer | ReadStream | AttachmentPayload | The audio to be sent. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendAudio(USER_ID, 'https://example.com/audio.mp3');
AttachmentPayload
to send cached attachment:client.sendAudio(USER_ID, { attachment_id: '55688' });
ReadStream
created from local file:const fs = require('fs');
client.sendAudio(USER_ID, fs.createReadStream('audio.mp3'));
sendImage(userId, image [, options])
Send images to specified user by uploading them or sharing a URL using the Send API. Supported formats are jpg, png and gif.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
image | String | Buffer | ReadStream | AttachmentPayload | The image to be sent. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendImage(USER_ID, 'https://example.com/vr.jpg');
AttachmentPayload
to send cached attachment:client.sendImage(USER_ID, { attachment_id: '55688' });
ReadStream
created from local file:const fs = require('fs');
client.sendImage(USER_ID, fs.createReadStream('vr.jpg'));
sendVideo(userId, video [, options])
Send videos to specified user by uploading them or sharing a URL using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
video | String | Buffer | ReadStream | AttachmentPayload | The video to be sent. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendVideo(USER_ID, 'https://example.com/video.mp4');
AttachmentPayload
to send cached attachment:client.sendVideo(USER_ID, { attachment_id: '55688' });
ReadStream
created from local file:const fs = require('fs');
client.sendVideo(USER_ID, fs.createReadStream('video.mp4'));
sendFile(userId, file [, options])
Send files to specified user by uploading them or sharing a URL using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
file | String | Buffer | ReadStream | AttachmentPayload | The file to be sent. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendFile(USER_ID, 'https://example.com/receipt.pdf');
AttachmentPayload
to send cached attachment:client.sendFile(USER_ID, { attachment_id: '55688' });
ReadStream
created from local file:const fs = require('fs');
client.sendFile(USER_ID, fs.createReadStream('receipt.pdf'));
sendTemplate(userId, template [, options])
Send structured message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
template | Object | Object of the template. |
options | Object | Other optional parameters. For example, messaging types or tags. |
Example:
client.sendTemplate(USER_ID, {
template_type: 'button',
text: 'title',
buttons: [
{
type: 'postback',
title: 'Start Chatting',
payload: 'USER_DEFINED_PAYLOAD',
},
],
});
sendButtonTemplate(userId, title, buttons [, options])
- Official DocsSend button message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
title | String | Text that appears above the buttons. |
buttons | Array<Object> | Array of button. Set of 1-3 buttons that appear as call-to-actions. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendButtonTemplate(USER_ID, 'What do you want to do next?', [
{
type: 'web_url',
url: 'https://petersapparel.parseapp.com',
title: 'Show Website',
},
{
type: 'postback',
title: 'Start Chatting',
payload: 'USER_DEFINED_PAYLOAD',
},
]);
sendGenericTemplate(userId, elements [, options])
- Official DocsSend generic message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
elements | Array<Object> | Array of element. Data for each bubble in message. |
options | Object | Other optional parameters, such as image_aspect_ratio , messaging types and tags. |
Example:
client.sendGenericTemplate(
USER_ID,
[
{
title: "Welcome to Peter's Hats",
image_url: 'https://petersfancybrownhats.com/company_image.png',
subtitle: "We've got the right hat for everyone.",
default_action: {
type: 'web_url',
url: 'https://peterssendreceiveapp.ngrok.io/view?item=103',
messenger_extensions: true,
webview_height_ratio: 'tall',
fallback_url: 'https://peterssendreceiveapp.ngrok.io/',
},
buttons: [
{
type: 'postback',
title: 'Start Chatting',
payload: 'DEVELOPER_DEFINED_PAYLOAD',
},
],
},
],
{ image_aspect_ratio: 'square' }
);
Adding a tag to a message allows you to send it outside the 24+1 window, for a limited number of use cases, per Messenger Platform policy.
Example:
client.sendGenericTemplate(
USER_ID,
[
{
// ...
},
],
{ tag: 'ISSUE_RESOLUTION' }
);
Available tags:
PAIRING_UPDATE
APPLICATION_UPDATE
ACCOUNT_UPDATE
PAYMENT_UPDATE
PERSONAL_FINANCE_UPDATE
SHIPPING_UPDATE
RESERVATION_UPDATE
ISSUE_RESOLUTION
APPOINTMENT_UPDATE
GAME_EVENT
TRANSPORTATION_UPDATE
FEATURE_FUNCTIONALITY_UPDATE
TICKET_UPDATE
sendListTemplate(userId, items, buttons [, options])
- Official DocsSend list message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
items | Array<Object> | Array of element. List view elements. |
buttons | Array<Object> | Array of button. List of buttons associated on the list template message (maximum of 1 button). |
options | Object | Other optional parameters, such as top_element_style and messaging types. |
Example:
client.sendListTemplate(
USER_ID,
[
{
title: 'Classic T-Shirt Collection',
image_url: 'https://peterssendreceiveapp.ngrok.io/img/collection.png',
subtitle: 'See all our colors',
default_action: {
type: 'web_url',
url: 'https://peterssendreceiveapp.ngrok.io/shop_collection',
messenger_extensions: true,
webview_height_ratio: 'tall',
fallback_url: 'https://peterssendreceiveapp.ngrok.io/',
},
buttons: [
{
title: 'View',
type: 'web_url',
url: 'https://peterssendreceiveapp.ngrok.io/collection',
messenger_extensions: true,
webview_height_ratio: 'tall',
fallback_url: 'https://peterssendreceiveapp.ngrok.io/',
},
],
},
],
[
{
type: 'postback',
title: 'View More',
payload: 'USER_DEFINED_PAYLOAD',
},
],
{ top_element_style: 'compact' }
);
sendOpenGraphTemplate(userId, elements [, options])
- Official DocsSend open graph message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
elements | Array<Object> | Array of element. Only one element is allowed. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendOpenGraphTemplate(USER_ID, [
{
url: 'https://open.spotify.com/track/7GhIk7Il098yCjg4BQjzvb',
buttons: [
{
type: 'web_url',
url: 'https://en.wikipedia.org/wiki/Rickrolling',
title: 'View More',
},
],
},
]);
sendMediaTemplate(userId, elements [, options])
- Official DocsSend media message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
elements | Array<Object> | Array of element. Only one element is allowed. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendMediaTemplate(USER_ID, [
{
media_type: 'image',
attachment_id: '1854626884821032',
buttons: [
{
type: 'web_url',
url: 'https://en.wikipedia.org/wiki/Rickrolling',
title: 'View Website',
},
],
},
]);
sendReceiptTemplate(userId, receipt [, options])
- Official DocsSend receipt message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
receipt | Object | payload of receipt template. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendReceiptTemplate(USER_ID, {
recipient_name: 'Stephane Crozatier',
order_number: '12345678902',
currency: 'USD',
payment_method: 'Visa 2345',
order_url: 'http://petersapparel.parseapp.com/order?order_id=123456',
timestamp: '1428444852',
elements: [
{
title: 'Classic White T-Shirt',
subtitle: '100% Soft and Luxurious Cotton',
quantity: 2,
price: 50,
currency: 'USD',
image_url: 'http://petersapparel.parseapp.com/img/whiteshirt.png',
},
{
title: 'Classic Gray T-Shirt',
subtitle: '100% Soft and Luxurious Cotton',
quantity: 1,
price: 25,
currency: 'USD',
image_url: 'http://petersapparel.parseapp.com/img/grayshirt.png',
},
],
address: {
street_1: '1 Hacker Way',
street_2: '',
city: 'Menlo Park',
postal_code: '94025',
state: 'CA',
country: 'US',
},
summary: {
subtotal: 75.0,
shipping_cost: 4.95,
total_tax: 6.19,
total_cost: 56.14,
},
adjustments: [
{
name: 'New Customer Discount',
amount: 20,
},
{
name: '$10 Off Coupon',
amount: 10,
},
],
});
sendAirlineBoardingPassTemplate(userId, attributes [, options])
- Official DocsSend airline boarding pass message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
attributes | Object | payload of boarding pass template. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendAirlineBoardingPassTemplate(RECIPIENT_ID, {
intro_message: 'You are checked in.',
locale: 'en_US',
boarding_pass: [
{
passenger_name: 'SMITH/NICOLAS',
pnr_number: 'CG4X7U',
travel_class: 'business',
seat: '74J',
auxiliary_fields: [
{
label: 'Terminal',
value: 'T1',
},
{
label: 'Departure',
value: '30OCT 19:05',
},
],
secondary_fields: [
{
label: 'Boarding',
value: '18:30',
},
{
label: 'Gate',
value: 'D57',
},
{
label: 'Seat',
value: '74J',
},
{
label: 'Sec.Nr.',
value: '003',
},
],
logo_image_url: 'https://www.example.com/en/logo.png',
header_image_url: 'https://www.example.com/en/fb/header.png',
qr_code: 'M1SMITH/NICOLAS CG4X7U nawouehgawgnapwi3jfa0wfh',
above_bar_code_image_url: 'https://www.example.com/en/PLAT.png',
flight_info: {
flight_number: 'KL0642',
departure_airport: {
airport_code: 'JFK',
city: 'New York',
terminal: 'T1',
gate: 'D57',
},
arrival_airport: {
airport_code: 'AMS',
city: 'Amsterdam',
},
flight_schedule: {
departure_time: '2016-01-02T19:05',
arrival_time: '2016-01-05T17:30',
},
},
},
{
passenger_name: 'JONES/FARBOUND',
pnr_number: 'CG4X7U',
travel_class: 'business',
seat: '74K',
auxiliary_fields: [
{
label: 'Terminal',
value: 'T1',
},
{
label: 'Departure',
value: '30OCT 19:05',
},
],
secondary_fields: [
{
label: 'Boarding',
value: '18:30',
},
{
label: 'Gate',
value: 'D57',
},
{
label: 'Seat',
value: '74K',
},
{
label: 'Sec.Nr.',
value: '004',
},
],
logo_image_url: 'https://www.example.com/en/logo.png',
header_image_url: 'https://www.example.com/en/fb/header.png',
qr_code: 'M1JONES/FARBOUND CG4X7U nawouehgawgnapwi3jfa0wfh',
above_bar_code_image_url: 'https://www.example.com/en/PLAT.png',
flight_info: {
flight_number: 'KL0642',
departure_airport: {
airport_code: 'JFK',
city: 'New York',
terminal: 'T1',
gate: 'D57',
},
arrival_airport: {
airport_code: 'AMS',
city: 'Amsterdam',
},
flight_schedule: {
departure_time: '2016-01-02T19:05',
arrival_time: '2016-01-05T17:30',
},
},
},
],
});
sendAirlineCheckinTemplate(userId, attributes [, options])
- Official DocsSend airline checkin message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
attributes | Object | payload of checkin template. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendAirlineCheckinTemplate(USER_ID, {
intro_message: 'Check-in is available now.',
locale: 'en_US',
pnr_number: 'ABCDEF',
flight_info: [
{
flight_number: 'f001',
departure_airport: {
airport_code: 'SFO',
city: 'San Francisco',
terminal: 'T4',
gate: 'G8',
},
arrival_airport: {
airport_code: 'SEA',
city: 'Seattle',
terminal: 'T4',
gate: 'G8',
},
flight_schedule: {
boarding_time: '2016-01-05T15:05',
departure_time: '2016-01-05T15:45',
arrival_time: '2016-01-05T17:30',
},
},
],
checkin_url: 'https://www.airline.com/check-in',
});
sendAirlineItineraryTemplate(userId, attributes [, options])
- Official DocsSend airline itinerary message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
attributes | Object | payload of itinerary template. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendAirlineItineraryTemplate(USER_ID, {
intro_message: "Here's your flight itinerary.",
locale: 'en_US',
pnr_number: 'ABCDEF',
passenger_info: [
{
name: 'Farbound Smith Jr',
ticket_number: '0741234567890',
passenger_id: 'p001',
},
{
name: 'Nick Jones',
ticket_number: '0741234567891',
passenger_id: 'p002',
},
],
flight_info: [
{
connection_id: 'c001',
segment_id: 's001',
flight_number: 'KL9123',
aircraft_type: 'Boeing 737',
departure_airport: {
airport_code: 'SFO',
city: 'San Francisco',
terminal: 'T4',
gate: 'G8',
},
arrival_airport: {
airport_code: 'SLC',
city: 'Salt Lake City',
terminal: 'T4',
gate: 'G8',
},
flight_schedule: {
departure_time: '2016-01-02T19:45',
arrival_time: '2016-01-02T21:20',
},
travel_class: 'business',
},
{
connection_id: 'c002',
segment_id: 's002',
flight_number: 'KL321',
aircraft_type: 'Boeing 747-200',
travel_class: 'business',
departure_airport: {
airport_code: 'SLC',
city: 'Salt Lake City',
terminal: 'T1',
gate: 'G33',
},
arrival_airport: {
airport_code: 'AMS',
city: 'Amsterdam',
terminal: 'T1',
gate: 'G33',
},
flight_schedule: {
departure_time: '2016-01-02T22:45',
arrival_time: '2016-01-03T17:20',
},
},
],
passenger_segment_info: [
{
segment_id: 's001',
passenger_id: 'p001',
seat: '12A',
seat_type: 'Business',
},
{
segment_id: 's001',
passenger_id: 'p002',
seat: '12B',
seat_type: 'Business',
},
{
segment_id: 's002',
passenger_id: 'p001',
seat: '73A',
seat_type: 'World Business',
product_info: [
{
title: 'Lounge',
value: 'Complimentary lounge access',
},
{
title: 'Baggage',
value: '1 extra bag 50lbs',
},
],
},
{
segment_id: 's002',
passenger_id: 'p002',
seat: '73B',
seat_type: 'World Business',
product_info: [
{
title: 'Lounge',
value: 'Complimentary lounge access',
},
{
title: 'Baggage',
value: '1 extra bag 50lbs',
},
],
},
],
price_info: [
{
title: 'Fuel surcharge',
amount: '1597',
currency: 'USD',
},
],
base_price: '12206',
tax: '200',
total_price: '14003',
currency: 'USD',
});
sendAirlineFlightUpdateTemplate(userId, attributes [, options])
- Official DocsSend airline flight update message templates to specified user using the Send API.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
attributes | Object | payload of update template. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
client.sendAirlineFlightUpdateTemplate(USER_ID, {
intro_message: 'Your flight is delayed',
update_type: 'delay',
locale: 'en_US',
pnr_number: 'CF23G2',
update_flight_info: {
flight_number: 'KL123',
departure_airport: {
airport_code: 'SFO',
city: 'San Francisco',
terminal: 'T4',
gate: 'G8',
},
arrival_airport: {
airport_code: 'AMS',
city: 'Amsterdam',
terminal: 'T4',
gate: 'G8',
},
flight_schedule: {
boarding_time: '2015-12-26T10:30',
departure_time: '2015-12-26T11:30',
arrival_time: '2015-12-27T07:30',
},
},
});
To send messages with quick replies to specified user using the Send API, pass quick_replies
option to send message methods, for example, with sendText
:
client.sendText(USER_ID, 'Pick a color:', {
quick_replies: [
{
content_type: 'text',
title: 'Red',
payload: 'DEVELOPER_DEFINED_PAYLOAD_FOR_PICKING_RED',
},
],
});
with sendImage
:
client.sendImage(USER_ID, 'https://example.com/vr.jpg', {
quick_replies: [
{
content_type: 'text',
title: 'Red',
payload: 'DEVELOPER_DEFINED_PAYLOAD_FOR_PICKING_RED',
},
],
});
It works with all of send message methods.
sendSenderAction(userId, action)
Send sender actions to specified user using the Send API, to let users know you are processing their request.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
action | String | Name of the action. |
Example:
client.sendSenderAction(USER_ID, 'typing_on');
markSeen(userId)
Mark last message as read for specified user.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
Example:
client.markSeen(USER_ID);
typingOn(userId)
Turn typing indicators on for specified user.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
Example:
client.typingOn(USER_ID);
typingOff(userId)
Turn typing indicators off for specified user.
Param | Type | Description |
---|---|---|
userId | String | Object | Page-scoped user ID of the recipient or recipient object. |
Example:
client.typingOff(USER_ID);
Note: Only attachments that were uploaded with the
is_reusable
property set totrue
can be sent to other message recipients.
uploadAttachment(type, attachment, options)
Upload specified type attachment using URL address, buffer, or stream.
Param | Type | Description |
---|---|---|
type | String | Must be one of `image |
attachment | String | Buffer | ReadStream | Attachment to be uploaded. |
options | Object | Other optional parameters. |
options.is_reusable | Set to true to make the saved asset sendable to other message recipients. Defaults to false . | |
options.filename | Required when upload from buffer. |
Example:
client.uploadAttachment('image', 'http://www.example.com/image.jpg', {
is_reusable: true,
});
Or using read stream:
const fs = require('fs');
client.uploadAttachment('image', fs.createReadStream('image.jpg'), {
is_reusable: true,
});
Or using buffer:
client.uploadAttachment('image', buffer, {
is_reusable: true,
filename: 'image.jpg',
});
uploadAudio(audio, options)
Upload audio attachment using URL address, buffer, or stream.
Param | Type | Description |
---|---|---|
audio | String | Buffer | ReadStream | The audio to be uploaded. |
options | Object | Other optional parameters. |
Example:
client.uploadAudio('http://www.example.com/audio.mp3', { is_reusable: true });
Or using read stream:
const fs = require('fs');
client.uploadAudio(fs.createReadStream('audio.mp3'), { is_reusable: true });
Or using buffer:
client.uploadAudio(buffer, {
is_reusable: true,
filename: 'audio.mp3',
});
uploadImage(image, options)
Upload image attachment using URL address, buffer, or stream.
Param | Type | Description |
---|---|---|
image | String | Buffer | ReadStream | The image to be uploaded. |
options | Object | Other optional parameters. |
Example:
client.uploadImage('http://www.example.com/image.jpg', { is_reusable: true });
Or using read stream:
const fs = require('fs');
client.uploadImage(fs.createReadStream('image.jpg'), { is_reusable: true });
Or using buffer:
client.uploadImage(buffer, {
is_reusable: true,
filename: 'image.jpg',
});
uploadVideo(video, options)
Upload video attachment using URL address, buffer, or stream.
Param | Type | Description |
---|---|---|
video | String | Buffer | ReadStream | The video to be uploaded. |
options | Object | Other optional parameters. |
Example:
client.uploadVideo('http://www.example.com/video.mp4', { is_reusable: true });
Or using read stream:
const fs = require('fs');
client.uploadVideo(fs.createReadStream('video.mp4'), { is_reusable: true });
Or using buffer:
client.uploadVideo(buffer, {
is_reusable: true,
filename: 'video.mp4',
});
uploadFile(file, options)
Upload file attachment using URL address, buffer, or stream.
Param | Type | Description |
---|---|---|
file | String | Buffer | ReadStream | The file to be uploaded. |
options | Object | Other optional parameters. |
Example:
client.uploadFile('http://www.example.com/file.pdf', { is_reusable: true });
Or using read stream:
const fs = require('fs');
client.uploadFile(fs.createReadStream('file.pdf'), { is_reusable: true });
Or using buffer:
client.uploadFile(buffer, {
is_reusable: true,
filename: 'file.pdf',
});
getMessageTags
Getting tags list via an API.
Example:
client.getMessageTags().then(tags => {
console.log(tags);
// [
// {
// tag: 'SHIPPING_UPDATE',
// description:
// 'The shipping_update tag may only be used to provide a shipping status notification for a product that has already been purchased. For example, when the product is shipped, in-transit, delivered, or delayed. This tag cannot be used for use cases beyond those listed above or for promotional content (ex: daily deals, coupons and discounts, or sale announcements).',
// },
// {
// tag: 'RESERVATION_UPDATE',
// description:
// 'The reservation_update tag may only be used to confirm updates to an existing reservation. For example, when there is a change in itinerary, location, or a cancellation (such as when a hotel booking is canceled, a car rental pick-up time changes, or a room upgrade is confirmed). This tag cannot be used for use cases beyond those listed above or for promotional content (ex: daily deals, coupons and discounts, or sale announcements).',
// },
// {
// tag: 'ISSUE_RESOLUTION',
// description:
// 'The issue_resolution tag may only be used to respond to a customer service issue surfaced in a Messenger conversation after a transaction has taken place. This tag is intended for use cases where the business requires more than 24 hours to resolve an issue and needs to give someone a status update and/or gather additional information. This tag cannot be used for use cases beyond those listed above or for promotional content (ex: daily deals, coupons and discounts, or sale announcements, nor can businesses use the tag to proactively message people to solicit feedback).',
// },
// {
// tag: 'APPOINTMENT_UPDATE',
// description:
// 'The appointment_update tag may only be used to provide updates about an existing appointment. For example, when there is a change in time, a location update or a cancellation (such as when a spa treatment is canceled, a real estate agent needs to meet you at a new location or a dental office proposes a new appointment time). This tag cannot be used for use cases beyond those listed above or for promotional content (ex: daily deals, coupons and discounts, or sale announcements).',
// },
// {
// tag: 'GAME_EVENT',
// description:
// 'The game_event tag may only be used to provide an update on user progression, a global event in a game or a live sporting event. For example, when a person’s crops are ready to be collected, their building is finished, their daily tournament is about to start or their favorite soccer team is about to play. This tag cannot be used for use cases beyond those listed above or for promotional content (ex: daily deals, coupons and discounts, or sale announcements).',
// },
// {
// tag: 'TRANSPORTATION_UPDATE',
// description:
// 'The transportation_update tag may only be used to confirm updates to an existing reservation. For example, when there is a change in status of any flight, train or ferry reservation (such as “ride canceled”, “trip started” or “ferry arrived”). This tag cannot be used for use cases beyond those listed above or for promotional content (ex: daily deals, coupons and discounts, or sale announcements).',
// },
// {
// tag: 'FEATURE_FUNCTIONALITY_UPDATE',
// description:
// 'The feature_functionality_update tag may only be used to provide an update on new features or functionality that become available in a bot. For example, announcing the ability to talk to a live agent in a bot, or that the bot has a new skill. This tag cannot be used for use cases beyond those listed above or for promotional content (ex: daily deals, coupons and discounts, or sale announcements).',
// },
// {
// tag: 'TICKET_UPDATE',
// description:
// 'The ticket_update tag may only be used to provide updates pertaining to an event for which a person already has a ticket. For example, when there is a change in time, a location update or a cancellation (such as when a concert is canceled, the venue has changed or a refund opportunity is available). This tag cannot be used for use cases beyond those listed above or for promotional content (ex: daily deals, coupons and discounts, or sale announcements).',
// },
// ]
});
sendBatch(requests)
Sends multiple requests in one batch.
Param | Type | Description |
---|---|---|
requests | Array<Object> | Subrequests in the batch. |
Example
const { MessengerBatch } = require('messaging-api-messenger');
client.sendBatch([
MessengerBatch.createText(USER_ID, '1'),
MessengerBatch.createText(USER_ID, '2'),
MessengerBatch.createText(USER_ID, '3'),
MessengerBatch.createText(USER_ID, '4'),
MessengerBatch.createText(USER_ID, '5'),
]);
To use the broadcast API, your Messenger bot must have the following permissions:
pages_messaging
pages_messaging_subscriptions
createMessageCreative(messages)
Param | Type | Description |
---|---|---|
messages | Array<Object> | The messages to send. |
Example
client
.createMessageCreative([
{
attachment: {
type: 'template',
payload: {
template_type: 'generic',
elements: [
{
title: 'Welcome to Our Marketplace!',
image_url: 'https://www.facebook.com/jaspers.png',
subtitle: 'Fresh fruits and vegetables. Yum.',
buttons: [
{
type: 'web_url',
url: 'https://www.jaspersmarket.com',
title: 'View Website',
},
],
},
],
},
},
},
])
.then(result => {
console.log(result);
// {
// message_creative_id: 938461089,
// }
});
The following message templates are not supported:
sendBroadcastMessage(messageCreativeId, options)
Param | Type | Description |
---|---|---|
messageCreativeId | Number | The message_creative_id of the message creative to send in the broadcast. |
options | Object | Other optional parameters. |
options.custom_label_id | Number | The id of custom label. |
Example
client.sendBroadcastMessage(938461089).then(result => {
console.log(result);
// {
// broadcast_id: 827,
// }
});
To send a broadcast message to the set of PSIDs associated with a label, pass label id as custom_label_id
option:
client.sendBroadcastMessage(938461089, { custom_label_id: LABEL_ID });
sendSponsoredMessage(adAccountId, args)
Param | Type | Description |
---|---|---|
adAccountId | String | The Ad account ID. See more |
message | Object | The Object to pass into request body. |
message.message_creative_id | Number | The ID of the Message Creative you want to send. |
message.daily_budget | Number | The maximum daily budget of the ad campaign for sending the sponsored message. |
message.bid_amount | Number | Maximum amount to bid for each message. |
message.targeting | JSON String | Option field for ads targeting |
Example
client
.sendSponsoredMessage('18910417349234', {
message_creative_id: 938461089,
daily_budget: 100,
bid_amount: 400,
targeting: "{'geo_locations': {'countries':['US']}}",
})
.then(result => {
console.log(result);
// {
// "ad_group_id": <AD_GROUP_ID>
// "broadcast_id": <BROADCAST_ID>
// "success": <RESPONSE_STATUS>
// }
});
createLabel(name)
Creating a Label.
Param | Type | Description |
---|---|---|
name | String | name of the custom label. |
Example:
client.createLabel('awesome').then(label => {
console.log(label);
// {
// id: 1712444532121303
// }
});
associateLabel(userId, labelId)
Associating a Label to a PSID.
Param | Type | Description |
---|---|---|
userId | String | Page-scoped user ID of the recipient. |
labelId | String | ID of the custom label. |
Example:
client.associateLabel(USER_ID, LABEL_ID);
dissociateLabel(userId, labelId)
Removing a Label From a PSID.
Param | Type | Description |
---|---|---|
userId | String | Page-scoped user ID of the recipient. |
labelId | String | ID of the custom label. |
Example:
client.dissociateLabel(USER_ID, LABEL_ID);
getAssociatedLabels(userId)
Retrieving Labels Associated with a PSID.
Param | Type | Description |
---|---|---|
userId | String | Page-scoped user ID of the recipient. |
Example:
client.getAssociatedLabels(USER_ID).then(result => {
console.log(result);
// {
// data: [
// {
// name: 'myLabel',
// id: '1001200005003',
// },
// {
// name: 'myOtherLabel',
// id: '1001200005002',
// },
// ],
// paging: {
// cursors: {
// before:
// 'QVFIUmx1WTBpMGpJWXprYzVYaVhabW55dVpycko4U2xURGE5ODNtNFZAPal94a1hTUnNVMUtoMVVoTzlzSDktUkMtQkUzWEFLSXlMS3ZALYUw3TURLelZAPOGVR',
// after:
// 'QVFIUmItNkpTbjVzakxFWGRydzdaVUFNNnNPaUl0SmwzVHN5ZAWZAEQ3lZANDAzTXFIM0NHbHdYSkQ5OG1GaEozdjkzRmxpUFhxTDl4ZAlBibnE4LWt1eGlTa3Bn',
// },
// },
// }
});
getLabelDetails(labelId, options)
Retrieving Label Details.
Param | Type | Description |
---|---|---|
labelId | String | ID of the custom label. |
options.fields | Array<String> | fields to retrieve with its ID. |
Example:
client.getLabelDetails(LABEL_ID, { fields: ['name'] }).then(result => {
console.log(result);
// {
// id: "1001200005002",
// name: "myLabel",
// }
});
getLabelList()
Retrieving a List of All Labels.
Example:
client.getLabelList().then(result => {
console.log(result);
// {
// data: [
// {
// name: 'myLabel',
// id: '1001200005003',
// },
// {
// name: 'myOtherLabel',
// id: '1001200005002',
// },
// ],
// paging: {
// cursors: {
// before:
// 'QVFIUmx1WTBpMGpJWXprYzVYaVhabW55dVpycko4U2xURGE5ODNtNFZAPal94a1hTUnNVMUtoMVVoTzlzSDktUkMtQkUzWEFLSXlMS3ZALYUw3TURLelZAPOGVR',
// after:
// 'QVFIUmItNkpTbjVzakxFWGRydzdaVUFNNnNPaUl0SmwzVHN5ZAWZAEQ3lZANDAzTXFIM0NHbHdYSkQ5OG1GaEozdjkzRmxpUFhxTDl4ZAlBibnE4LWt1eGlTa3Bn',
// },
// },
// }
});
deleteLabel(labelId)
Deleting a Label.
Param | Type | Description |
---|---|---|
labelId | String | ID of the custom label. |
Example:
client.deleteLabel(LABEL_ID);
startReachEstimation(customLabelId)
Param | Type | Description |
---|---|---|
customLabelId | Number | The custom label ID. |
Example
client.startReachEstimation(938461089).then(result => {
console.log(result);
// {
// "reach_estimation_id": <REACH_ESTIMATION_ID>
// }
});
getReachEstimate(reachEstimationId)
Param | Type | Description |
---|---|---|
reachEstimationId | Number | The reach estimate ID from startReachEstimation. |
Example
client.getReachEstimate(73450120243).then(result => {
console.log(result);
// {
// "reach_estimation": "<REACH_ESTIMATE>"
// "id": "<REACH_ESTIMATION_ID>"
// }
});
getBroadcastMessagesSent(broadcastId)
Param | Type | Description |
---|---|---|
broadcastId | Number | The broadcast ID. |
Example
client.getBroadcastMessagesSent(73450120243).then(result => {
console.log(result);
// [
// {
// "name": "messages_sent",
// "period": "lifetime",
// "values": [
// {
// "value": 1000,
// "end_time": "1970-01-02T00:00:00+0000"
// }
// ],
// "title": "Lifetime number of messages sent from the page broadcast",
// "description": "Lifetime: The total number of messages sent from a Page to people.",
// "id": "1301333349933076/insights/messages_sent"
// }
// ]
});
getUserProfile(userId)
Retrieving a Person's Profile.
Param | Type | Description |
---|---|---|
userId | String | Page-scoped user ID of the recipient. |
Example:
client.getUserProfile(USER_ID).then(user => {
console.log(user);
// {
// first_name: 'Johnathan',
// last_name: 'Jackson',
// profile_pic: 'https://example.com/pic.png',
// locale: 'en_US',
// timezone: 8,
// gender: 'male',
// }
});
getMessengerProfile(fields)
Retrieves the current value of one or more Messenger Profile properties by name.
Param | Type | Description |
---|---|---|
fields | Array<String> | Value must be among account_linking_url , persistent_menu , get_started , greeting , whitelisted_domains , payment_settings , target_audience , home_url . |
Example:
client.getMessengerProfile(['get_started', 'persistent_menu']).then(profile => {
console.log(profile);
// [
// {
// get_started: {
// payload: 'GET_STARTED',
// },
// },
// {
// persistent_menu: [
// {
// locale: 'default',
// composer_input_disabled: true,
// call_to_actions: [
// {
// type: 'postback',
// title: 'Restart Conversation',
// payload: 'RESTART',
// },
// ],
// },
// ],
// },
// ]
});
setMessengerProfile(profile)
Sets the values of one or more Messenger Profile properties. Only properties set in the request body will be overwritten.
Param | Type | Description |
---|---|---|
profile | Object | Object of Profile. |
Example:
client.setMessengerProfile({
get_started: {
payload: 'GET_STARTED',
},
persistent_menu: [
{
locale: 'default',
composer_input_disabled: true,
call_to_actions: [
{
type: 'postback',
title: 'Restart Conversation',
payload: 'RESTART',
},
],
},
],
});
deleteMessengerProfile(fields)
Deletes one or more Messenger Profile properties. Only properties specified in the fields array will be deleted.
Param | Type | Description |
---|---|---|
fields | Array<String> | Value must be among account_linking_url , persistent_menu , get_started , greeting , whitelisted_domains , payment_settings , target_audience , home_url . |
Example:
client.deleteMessengerProfile(['get_started', 'persistent_menu']);
getPersistentMenu
Retrieves the current value of persistent menu.
Example:
client.getPersistentMenu().then(menu => {
console.log(menu);
// [
// {
// locale: 'default',
// composer_input_disabled: true,
// call_to_actions: [
// {
// type: 'postback',
// title: 'Restart Conversation',
// payload: 'RESTART',
// },
// {
// type: 'web_url',
// title: 'Powered by ALOHA.AI, Yoctol',
// url: 'https://www.yoctol.com/',
// },
// ],
// },
// ]
});
setPersistentMenu(menu)
Sets the values of persistent menu.
Param | Type | Description |
---|---|---|
menu | Array<Object> | Array of menu. |
Example:
client.setPersistentMenu([
{
locale: 'default',
call_to_actions: [
{
title: 'Play Again',
type: 'postback',
payload: 'RESTART',
},
{
title: 'Language Setting',
type: 'nested',
call_to_actions: [
{
title: '中文',
type: 'postback',
payload: 'CHINESE',
},
{
title: 'English',
type: 'postback',
payload: 'ENGLISH',
},
],
},
{
title: 'Explore D',
type: 'nested',
call_to_actions: [
{
title: 'Explore',
type: 'web_url',
url: 'https://www.youtube.com/watch?v=v',
webview_height_ratio: 'tall',
},
{
title: 'W',
type: 'web_url',
url: 'https://www.facebook.com/w',
webview_height_ratio: 'tall',
},
{
title: 'Powered by YOCTOL',
type: 'web_url',
url: 'https://www.yoctol.com/',
webview_height_ratio: 'tall',
},
],
},
],
},
]);
Note: You must set a get started button to use the persistent menu.
deletePersistentMenu
Deletes persistent menu.
Example:
client.deletePersistentMenu();
getGetStarted
Retrieves the current value of get started button.
Example:
client.getGetStarted().then(getStarted => {
console.log(getStarted);
// {
// payload: 'GET_STARTED',
// }
});
setGetStarted(payload)
Sets the values of get started button.
Param | Type | Description |
---|---|---|
payload | String | Payload sent back to your webhook in a messaging_postbacks event when the 'Get Started' button is tapped. |
Example:
client.setGetStarted('GET_STARTED');
deleteGetStarted
Deletes get started button.
Example:
client.deleteGetStarted();
getGreeting
Retrieves the current value of greeting text.
Example:
client.getGreeting().then(greeting => {
console.log(greeting);
// [
// {
// locale: 'default',
// text: 'Hello!',
// },
// ]
});
setGreeting(greeting)
Sets the values of greeting text.
Param | Type | Description |
---|---|---|
greeting | Array<Object> | Array of greeting. |
Example:
client.setGreeting([
{
locale: 'default',
text: 'Hello!',
},
]);
deleteGreeting
Deletes greeting text.
Example:
client.deleteGreeting();
getWhitelistedDomains
Retrieves the current value of whitelisted domains.
Example:
client.getWhitelistedDomains().then(domains => {
console.log(domains);
// ['http://www.example.com/']
});
setWhitelistedDomains(domains)
Sets the values of whitelisted domains.
Param | Type | Description |
---|---|---|
domains | Array<String> | Array of whitelisted_domain. |
Example:
client.setWhitelistedDomains(['www.example.com']);
deleteWhitelistedDomains
Deletes whitelisted domains.
Example:
client.deleteWhitelistedDomains();
getAccountLinkingURL
Retrieves the current value of account linking URL.
Example:
client.getAccountLinkingURL().then(accountLinking => {
console.log(accountLinking);
// {
// account_linking_url:
// 'https://www.example.com/oauth?response_type=code&client_id=1234567890&scope=basic',
// }
});
setAccountLinkingURL(url)
Sets the values of account linking URL.
Param | Type | Description |
---|---|---|
url | String | Account linking URL. |
Example:
client.setAccountLinkingURL(
'https://www.example.com/oauth?response_type=code&client_id=1234567890&scope=basic'
);
deleteAccountLinkingURL
Deletes account linking URL.
Example:
client.deleteAccountLinkingURL();
getPaymentSettings
Retrieves the current value of payment settings.
Example:
client.getPaymentSettings().then(settings => {
console.log(settings);
// {
// privacy_url: 'www.facebook.com',
// public_key: 'YOUR_PUBLIC_KEY',
// test_users: ['12345678'],
// }
});
setPaymentPrivacyPolicyURL(url)
Sets the values of payment privacy policy URL.
Param | Type | Description |
---|---|---|
url | String | Payment privacy policy URL. |
Example:
client.setPaymentPrivacyPolicyURL('https://www.example.com');
setPaymentPublicKey(key)
Sets the values of payment public key.
Param | Type | Description |
---|---|---|
key | String | payment public key. |
Example:
client.setPaymentPublicKey('YOUR_PUBLIC_KEY');
setPaymentTestUsers(users)
Sets the values of payment test users.
Param | Type | Description |
---|---|---|
users | Array<String> | Array of IDs for people that will test payments in your app. |
Example:
client.setPaymentTestUsers(['12345678']);
deletePaymentSettings
Deletes payment settings.
Example:
client.deletePaymentSettings();
getTargetAudience
Retrieves the current value of target audience.
Example:
client.getTargetAudience().then(targetAudience => {
console.log(targetAudience);
// {
// audience_type: 'custom',
// countries: {
// whitelist: ['US', 'CA'],
// },
// }
});
setTargetAudience(type, whitelist, blacklist)
Sets the values of target audience.
Param | Type | Description |
---|---|---|
type | String | Audience type. Valid values include `all |
whitelist | Array<String> | List of ISO 3166 Alpha-2 codes. Users in any of the blacklist countries won't see your bot on discovery surfaces on Messenger Platform. |
blacklist | Array<String> | List of ISO 3166 Alpha-2 codes. Users in any of the whitelist countries will see your bot on discovery surfaces on Messenger Platform. |
Exmaple:
client.setTargetAudience('custom', ['US', 'CA'], ['UK']);
deleteTargetAudience
Deletes target audience.
Example:
client.deleteTargetAudience();
getHomeURL
Retrieves the current value of chat extension home URL.
Example:
client.getHomeURL().then(chatExtension => {
console.log(chatExtension);
// {
// url: 'http://petershats.com/send-a-hat',
// webview_height_ratio: 'tall',
// in_test: true,
// }
});
setHomeURL(url, attributes)
Sets the values of chat extension home URL.
Param | Type | Description |
---|---|---|
url | String | The URL to be invoked from drawer. |
attributes | Object | Other properties of home URL. |
Exmaple:
client.setHomeURL('http://petershats.com/send-a-hat', {
webview_height_ratio: 'tall',
in_test: true,
});
deleteHomeURL
Deletes chat extension home URL.
Example:
client.deleteHomeURL();
generateMessengerCode(options)
Generating a Messenger code.
Param | Type | Description |
---|---|---|
options | Object | Optional parameters of generating a Messenger code. |
options.image_size | Number | The size, in pixels, for the image you are requesting. |
options.data | Object | If creating a parametric code, pass { "data": { "ref": "YOUR_REF_HERE" } } . |
Example:
client
.generateMessengerCode({
data: {
ref: 'billboard-ad',
},
image_size: 1000,
})
.then(code => {
console.log(code);
// {
// "uri": "YOUR_CODE_URL_HERE"
// }
});
passThreadControl(userId, targetAppId, metadata)
- Official DocsPasses thread control from your app to another app.
Param | Type | Description |
---|---|---|
userId | String | The PSID of the message recipient. |
targetAppId | Number | The app ID of the Secondary Receiver to pass thread control to. |
metadata | String | Metadata passed to the receiving app in the pass_thread_control webhook event. |
Example:
client.passThreadControl(USER_ID, APP_ID, 'free formed text for another app');
passThreadControlToPageInbox(userId, metadata)
- Official DocsPasses thread control from your app to "Page Inbox" app.
Param | Type | Description |
---|---|---|
userId | String | The PSID of the message recipient. |
metadata | String | Metadata passed to the receiving app in the pass_thread_control webhook event. |
Example:
client.passThreadControlToPageInbox(
USER_ID,
'free formed text for another app'
);
takeThreadControl(userId, metadata)
- Official DocsTakes control of a specific thread from a Secondary Receiver app.
Param | Type | Description |
---|---|---|
userId | String | The PSID of the message recipient. |
metadata | String | Metadata passed back to the secondary app in the take_thread_control webhook event. |
Example:
client.takeThreadControl(USER_ID, 'free formed text for another app');
requestThreadControl(userId, metadata)
- Official DocsRequests control of a specific thread from a Primary Receiver app.
Param | Type | Description |
---|---|---|
userId | String | The PSID of the message recipient. |
metadata | String | Metadata passed to the primary app in the request_thread_control webhook event. |
Example:
client.requestThreadControl(USER_ID, 'free formed text for primary app');
getSecondaryReceivers
- Official DocsRetrieves the list of apps that are Secondary Receivers for a page.
Example:
client.getSecondaryReceivers().then(receivers => {
console.log(receivers);
// [
// {
// "id": "12345678910",
// "name": "David's Composer"
// },
// {
// "id": "23456789101",
// "name": "Messenger Rocks"
// }
// ]
});
getInsights(metrics, options)
Retrieves the insights of your Facebook Page.
Param | Type | Description |
---|---|---|
metrics | Array | The metrics you want to check. |
options | Object | Optional arguments. |
options.since | number | Optional. UNIX timestamp of the start time to get the metric for. |
options.until | number | Optional. UNIX timestamp of the end time to get the metric for. |
Example:
client.getInsights(['page_messages_active_threads_unique']).then(counts => {
console.log(counts);
// [
// {
// "name": "<METRIC>",
// "period": "day",
// "values": [
// {
// "value": "<VALUE>",
// "end_time": "<UTC_TIMESTAMP>"
// },
// {
// "value": "<VALUE>",
// "end_time": "<UTC_TIMESTAMP>"
// }
// ]
// }
// ]
});
getDailyUniqueActiveThreadCounts
Retrieves a count of the unique active threads your app participated in per day.
Example:
client.getDailyUniqueActiveThreadCounts().then(counts => {
console.log(counts);
// [
// {
// "name": "page_messages_active_threads_unique",
// "period": "day",
// "values": [
// {
// "value": 83111,
// "end_time": "2017-02-02T08:00:00+0000"
// },
// {
// "value": 85215,
// "end_time": "2017-02-03T08:00:00+0000"
// },
// {
// "value": 87175,
// "end_time": "2017-02-04T08:00:00+0000"
// }
// ],
// "title": "Daily unique active threads count by thread fbid",
// "description": "Daily: total unique active threads created between users and page.",
// "id": "1234567/insights/page_messages_active_threads_unique/day"
// }
// ]
});
getBlockedConversations
Retrieves the number of conversations with the Page that have been blocked.
Example:
client.getBlockedConversations().then(counts => {
console.log(counts);
// [
// {
// "name": "<METRIC>",
// "period": "day",
// "values": [
// {
// "value": "<VALUE>",
// "end_time": "<UTC_TIMESTAMP>"
// },
// {
// "value": "<VALUE>",
// "end_time": "<UTC_TIMESTAMP>"
// }
// ]
// }
// ]
});
getReportedConversations
Retrieves the number of conversations from your Page that have been reported by people for reasons such as spam, or containing inappropriate content.
Example:
client.getReportedConversations().then(counts => {
console.log(counts);
// [
// {
// "name": "<METRIC>",
// "period": "day",
// "values": [
// {
// "value": "<VALUE>",
// "end_time": "<UTC_TIMESTAMP>"
// },
// {
// "value": "<VALUE>",
// "end_time": "<UTC_TIMESTAMP>"
// }
// ]
// }
// ]
});
getReportedConversationsByReportType
Retrieves the number of conversations from your Page that have been reported by people for reasons such as spam, or containing inappropriate content.
Example:
client.getReportedConversationsByReportType().then(counts => {
console.log(counts);
// [
// {
// "name": "<METRIC>",
// "period": "day",
// "values": [
// {
// "value": "<VALUE>",
// "end_time": "<UTC_TIMESTAMP>"
// },
// {
// "value": "<VALUE>",
// "end_time": "<UTC_TIMESTAMP>"
// }
// ]
// }
// ]
});
getDailyUniqueConversationCounts
Deprecated
getDailyUniqueConversationCounts
is deprecated as of November 7, 2017. This metric will be removed in Graph API v2.12.
Retrieves a count of actions that were initiated by people your app was in an active thread with per day.
Example:
client.getDailyUniqueConversationCounts().then(counts => {
console.log(counts);
// [
// {
// "name": "page_messages_feedback_by_action_unique",
// "period": "day",
// "values": [
// {
// "value": {
// "TURN_ON": 40,
// "TURN_OFF": 167,
// "DELETE": 720,
// "OTHER": 0,
// "REPORT_SPAM": 0
// },
// "end_time": "2017-02-02T08:00:00+0000"
// },
// {
// "value": {
// "TURN_ON": 38,
// "DELETE": 654,
// "TURN_OFF": 155,
// "REPORT_SPAM": 1,
// "OTHER": 0
// },
// "end_time": "2017-02-03T08:00:00+0000"
// }
// ],
// "title": "Daily unique conversation count broken down by user feedback actions",
// "description": "Daily: total unique active threads created between users and page.",
// "id": "1234567/insights/page_messages_active_threads_unique/day"
// }
// ],
});
setNLPConfigs(config)
Set values of NLP configs.
Param | Type | Description |
---|---|---|
config | Object | Configuration of NLP. |
config.nlp_enabled | Boolean | Optional. Either enable NLP or disable NLP for that Page. |
config.model | String | Optional. Specifies the NLP model to use. Either one of {CHINESE, CROATIAN, DANISH, DUTCH, ENGLISH, FRENCH_STANDARD, GERMAN_STANDARD, HEBREW, HUNGARIAN, IRISH, ITALIAN_STANDARD, KOREAN, NORWEGIAN_BOKMAL, POLISH, PORTUGUESE, ROMANIAN, SPANISH, SWEDISH, VIETNAMESE} , or CUSTOM . |
config.custom_token | String | Optional. Access token from Wit. |
config.verbose | Boolean | Optional. Specifies whether verbose mode if enabled, which returns extra information like the position of the detected entity in the query. |
config.n_best | Number | Optional. The number of entities to return, in descending order of confidence. Minimum 1. Maximum 8. Defaults to 1. |
Example:
client.setNLPConfigs({
nlp_enabled: true,
});
enableNLP
Enabling Built-in NLP.
Example:
client.enableNLP();
disableNLP
Disabling Built-in NLP.
Example:
client.disableNLP();
logCustomEvents(activity)
Log custom events by using the Application Activities Graph API endpoint.
Param | Type | Description |
---|---|---|
activity | Object | |
activity.app_id | Number | ID of the app. |
activity.page_id | String | ID of the page. |
activity.page_scoped_user_id | String | Page-scoped user ID of the recipient. |
activity.events | Array<Object> | Custom events. |
Example:
client.logCustomEvents({
app_id: APP_ID,
page_id: PAGE_ID,
page_scoped_user_id: USER_ID,
events: [
{
_eventName: 'fb_mobile_purchase',
_valueToSum: 55.22,
_fb_currency: 'USD',
},
],
});
getIdsForApps({ user_id, app_secret, ...options })
Given a user ID for an app, retrieve the IDs for other apps owned by the same business
Param | Type | Description |
---|---|---|
user_id | String | Page-scoped user ID. |
app_secret | String | Secret of the app. |
options.app | String | The app to retrieve the IDs. |
options.page | String | The page to retrieve the IDs. |
Example:
client
.getIdsForApps({
user_id: USER_ID,
app_secret: APP_SECRET,
})
.then(result => {
console.log(result);
// {
// data: [
// {
// id: '10152368852405295',
// app: {
// category: 'Business',
// link: 'https://www.facebook.com/games/?app_id=1419232575008550',
// name: "John's Game App",
// id: '1419232575008550',
// },
// },
// {
// id: '645195294',
// app: {
// link: 'https://apps.facebook.com/johnsmovieappns/',
// name: 'JohnsMovieApp',
// namespace: 'johnsmovieappns',
// id: '259773517400382',
// },
// },
// ],
// paging: {
// cursors: {
// before: 'MTQ4OTU4MjQ5Nzc4NjY4OAZDZDA',
// after: 'NDAwMDExOTA3MDM1ODMwA',
// },
// },
// };
});
getIdsForPages({ user_id, app_secret, ...options })
Given a user ID for a Page (associated with a bot), retrieve the IDs for other Pages owned by the same business.
Param | Type | Description |
---|---|---|
user_id | String | Page-scoped user ID. |
app_secret | String | Secret of the app. |
options.app | String | The app to retrieve the IDs. |
options.page | String | The page to retrieve the IDs. |
Example:
client
.getIdsForPages({
user_id: USER_ID,
app_secret: APP_SECRET,
})
.then(result => {
console.log(result);
// {
// data: [
// {
// id: '12345123', // The psid for the user for that page
// page: {
// category: 'Musician',
// link:
// 'https://www.facebook.com/Johns-Next-Great-Thing-380374449010653/',
// name: "John's Next Great Thing",
// id: '380374449010653',
// },
// },
// ],
// paging: {
// cursors: {
// before: 'MTQ4OTU4MjQ5Nzc4NjY4OAZDZDA',
// after: 'NDAwMDExOTA3MDM1ODMwA',
// },
// },
// };
});
createSubscription
Create new Webhooks subscriptions.
Param | Type | Description |
---|---|---|
app_id | String | ID of the app. |
access_token | String | App access token. |
callback_url | String | The URL that will receive the POST request when an update is triggered, and a GET request when attempting this publish operation. |
verify_token | String | An arbitrary string that can be used to confirm to your server that the request is valid. |
fields | Array<String> | One or more of the set of valid fields in this object to subscribe to. |
object | String | Indicates the object type that this subscription applies to. Defaults to page . |
include_values | Boolean | Indicates if change notifications should include the new values. |
Example:
client.createSubscription({
app_id: APP_ID,
access_token: APP_ACCESS_TOKEN,
callback_url: 'https://mycallback.com',
fields: ['messages', 'messaging_postbacks', 'messaging_referrals'],
verify_token: VERIFY_TOKEN,
});
Or provide app id and app secret instead of app access token:
client.createSubscription({
app_id: APP_ID,
access_token: `${APP_ID}|${APP_SECRET}`,
callback_url: 'https://mycallback.com',
fields: ['messages', 'messaging_postbacks', 'messaging_referrals'],
verify_token: VERIFY_TOKEN,
});
Default Fields:
messages
messaging_postbacks
messaging_optins
messaging_referrals
messaging_handovers
messaging_policy_enforcement
getPageInfo
Get page name and page id using Graph API.
Example:
client.getPageInfo().then(page => {
console.log(page);
// {
// name: 'Bot Demo',
// id: '1895382890692546',
// }
});
To avoid sending requests to real Messenger server, specify origin
option when constructing your client:
const { MessengerClient } = require('messaging-api-messenger');
const client = MessengerClient.connect({
accessToken: ACCESS_TOKEN,
origin: 'https://mydummytestserver.com',
});
Warning: Don't do this on production server.
0.6.15 / 2018-02-26
requestThreadControl
:client.requestThreadControl(USER_ID, 'free formed text for primary app');
FAQs
Messaging API client for Messenger
The npm package messaging-api-messenger receives a total of 3,661 weekly downloads. As such, messaging-api-messenger popularity was classified as popular.
We found that messaging-api-messenger demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.