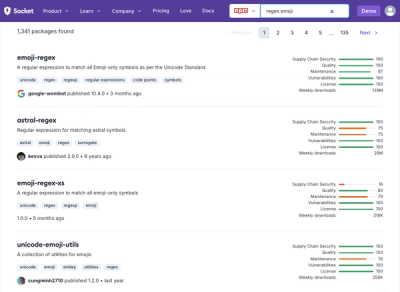
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Mflow is the monad-style flow control library for nodejs.
This library is inspired by followings:
Supported monad-style flows are followings:
Mflow uses ECMAScript 6 generators.
Currently you must use the --harmony-generators
flag when running node 0.11.x.
$ npm install mflow
This is an async flow.
var fs = require('fs');
var mflow = require('mflow');
var async = mflow.async;
var flow = async(function *() {
var a = yield function(f) { fs.readFile('file1', 'utf8', f); };
var b = yield function(f) { fs.readFile('file2', 'utf8', f); };
return a + b;
});
flow(function (err, data) {
if (err) throw err;
console.log(data);
});
Mflow provides following top-level functions:
These functions accept a generator function which has no parameter.
Makes an async flow.
fn
: a generator functionThe yieldable values are followings:
yield
returns the async function's result.
var fs = require('fs');
var mflow = require('mflow');
var async = mflow.async;
var flow = async(function *() {
var a = yield function(f) { fs.readFile('file1', 'utf8', f); };
var b = yield function(f) { fs.readFile('file2', 'utf8', f); };
return a + b;
});
flow(function (err, data) {
if (err) throw err;
console.log(data);
});
Runs multiple async functions in parallel.
fn...
: multiple functions or an array of functionvar fs = require('fs');
var mflow = require('mflow');
var async = mflow.async;
var flow = async(function *() {
var a = function(f) { fs.readFile('file1', 'utf8', f); };
var b = function(f) { fs.readFile('file2', 'utf8', f); };
var results = yield async.join(a, b);
// instead of multiple arguments, you can use an array.
// var results = yield async.join([a, b]);
return results[0] + results[1];
});
flow(function (err, data) {
if (err) throw err;
console.log(data);
});
Makes a lazy flow.
fn
: a generator functionThe yieldable values are followings:
var mflow = require('mflow');
var lazy = mflow.lazy;
var x = 10;
var result = lazy(function *() {
return x + 10;
});
console.log(result()); // 20
When a non-function value is yielded, the value is returned without any change.
var mflow = require('mflow');
var lazy = mflow.lazy;
var x = 10;
var lazyValue1 = lazy(function *() { return x + 10; });
var lazyValue2 = lazy(function *() { return x * 10; });
function add(value1, value2) {
return lazy(function *() {
var a = yield value1;
var b = yield value2;
return a + b;
});
};
console.log(add(lazyValue1, lazyValue2)); // 120
console.log(add(1, 2)); // 3
Runs a flow which results a successful value or null
.
fn
: a generator functionnull
The yieldable values are followings:
null
undefined
When null
or undefined
is yielded, the flow is stopped and results null
.
Otherwise the yielded value is returned without any change.
var mflow = require('mflow');
var maybe = mflow.maybe;
function add(x, y) {
return maybe(function *() {
var a = yield x;
var b = yield y;
return a + b;
});
}
console.log(add(1, 2)); // 3
console.log(add(1, null)); // null
Makes a state flow.
fn
: a generator functionThe yieldable values are followings:
var mflow = require('mflow');
var state = mflow.state;
function pop() {
return function (s) {
return [s.shift(), s];
};
}
function push(value) {
return function (s) {
s.unshift(value);
return [null, s];
};
}
var flow = state(function* () {
var a = yield pop();
if (a === 5) {
yield push(5);
} else {
yield push(3);
yield push(8);
}
return 10;
});
var result = flow([9, 0, 2, 1, 0]);
console.log(result[0]); // 10
console.log(result[1]); // [8, 3, 0, 2, 1, 0]
Gets a flow's state.
yield
, a flow's state are returned.pop
and push
functions in abobe code can be replaced with following implementations using state.get
and state.put
:
var pop = function () {
return state(function *() {
var s = yield state.get();
var ret = s.shift();
yield state.put(s);
return ret;
});
};
var push = function (value) {
return state(function *() {
var s = yield state.get();
s.unshift(value);
yield state.put(s);
});
};
Puts a new state as a flow's state.
s
: a new stateyield
, a flow's state is replaced with the argument.See state.get()
.
Runs a flow and returns the flow's result.
flow
: a state flows
: a flow's stateFollowings are equivalent:
var result = state.eval(flow, [9, 0, 2, 1, 0]);
console.log(result); // 10
var result = flow([9, 0, 2, 1, 0]);
console.log(result[0]); // 10
Runs a flow and returns the flow's state.
flow
: a state flows
: a flow's stateFollowings are equivalent:
var result = state.exec(flow, [9, 0, 2, 1, 0]);
console.log(result); // [8, 3, 0, 2, 1, 0]
var result = flow([9, 0, 2, 1, 0]);
console.log(result[1]); // [8, 3, 0, 2, 1, 0]
$ mocha --harmony-generators
MIT
FAQs
monad-style flow control library
The npm package mflow receives a total of 1 weekly downloads. As such, mflow popularity was classified as not popular.
We found that mflow demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.