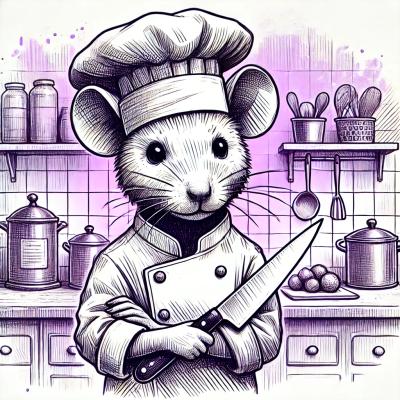
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
A wrapper for requesting audio and video permissions on JavaScript websites.
A lightweight package for requesting camera and microphone permissions from the browser with better error handling.
Note: This library does not include any UI or components and is designed to help you implement those pieces more easily through better error handling. You can, however, use the code included in the examples.
One of the most frustrating pieces of building a video chatting website is helping the user set up camera and microphone access. Ideally, you should be able to use the Permissions API to request media device access, but it isn't yet available on Safari which accounts for a significant group of users.
navigation.mediaDevices.getUserMedia()
is now available on all major browsers, but it returns different errors for the same problems across various browsers (Chrome, Firefox, Edge, Safari) and operating systems (macOS, Windows). Some problems, like the system denying permission, are more common on macOS. Whereas being unable to start the video stream due to another application using it is more Windows specific. Oh, and Firefox is the only one with any error documentation at all.
Handling these errors across browsers and OS's can be difficult, but is crucial to a great user experience. mic-check
categorizes the major errors and allows you to focus on building a great experience!
npm install mic-check
or
yarn add mic-check
import {
MediaPermissionsError
MediaPermissionsErrorType,
requestMediaPermissions
} from 'mic-check';
requestMediaPermissions()
.then(() => {
// can successfully access camera and microphone streams
// DO SOMETHING HERE
})
.catch((err: MediaPermissionsError) => {
const { type, name, message } = err;
if (type === MediaPermissionsErrorType.SystemPermissionDenied) {
// browser does not have permission to access camera or microphone
} else if (type === MediaPermissionsErrorType.UserPermissionDenied) {
// user didn't allow app to access camera or microphone
} else if (type === MediaPermissionsErrorType.CouldNotStartVideoSource) {
// camera is in use by another application (Zoom, Skype) or browser tab (Google Meet, Messenger Video)
// (mostly Windows specific problem)
} else {
// not all error types are handled by this library
}
});
import {
MediaPermissionsError
MediaPermissionsErrorType,
requestAudioPermissions,
requestVideoPermissions,
requestMediaPermissions
} from 'mic-check';
// Requesting AUDIO permission only:
requestAudioPermissions()
.then(() => {})
.catch((err: MediaPermissionsError) => {});
// another way to request AUDIO only...
requestMediaPermissions({audio: true, video: false})
.then(() => {})
.catch((err: MediaPermissionsError) => {});
// Requesting VIDEO permission only:
requestVideoPermissions()
.then(() => {})
.catch((err: MediaPermissionsError) => {});
// another way to request VIDEO only...
requestMediaPermissions({audio: false, video: true})
.then(() => {})
.catch((err: MediaPermissionsError) => {});
Error Type (MediaPermissionsError) | Description |
---|---|
SystemPermissionDenied | Browser does not have access to camera or microphone (common on macOS) |
UserPermissionDenied | User did not grant camera or microphone permissions in the popup |
CouldNotStartVideoSource | Another application or browser tab is using the camera (common on Windows) |
Generic | Everything else |
These are common errors and recommended user actions to resolve them that we have discovered from testing the four major browsers on Windows and macOS.
Problem | OS (macOS, Windows) | Chrome | Safari | Edge | Firefox | Error Type (MediaPermissionsError) | Recommended User Action |
---|---|---|---|---|---|---|---|
Browser doesn't have System Preferences access to camera or mic | macOS | NotAllowedError: Permission denied by system | N/A (Safari always has access) | NotAllowedError: Permission denied | NotFoundError: The object can not be found here. | SystemPermissionDenied | Open Mac System Preferences and enable under Camera |
Browser doesn't have System Preferences access to camera or mic | Windows | NotReadableError: Could not start video source | N/A (Safari not available) | NotReadableError: Could not start video source | NotReadableError: Failed to allocate videosource | N/A | Open Windows Settings and enable under Camera |
User denied permission to access camera or mic | macOS, Windows | NotAllowedError: Permission denied | NotAllowedError: The request is not allowed by the user agent or the platform in the current context, possibly because the user denied permission. | NotAllowedError: Permission denied | NotAllowedError: The request is not allowed by the user agent or the platform in the current context. | UserPermissionDenied | Manually give permission by clicking on Camera Blocked icon (Safari needs a reprompt) |
Camera in use by another application (Zoom, Webex) or tab (Google Meet, Messenger Video) | Windows | NotReadableError: Could not start video source | N/A (Safari not available) | NotReadableError: Could not start video source | AbortError: Starting videoinput failed | CouldNotStartVideoSource | Turn off other video |
All Other Errors | Generic |
We have found Google Meet to have one of the best onboarding experiences for camera and microphone permissions. They do a good job of explaining permissions, showing how to allow permissions, and deep linking into System Preferences or Settings when needed. This example, built with React and Material UI, closely copies the Google Meet experience.
You can try it out by going to examples/react-example
and running:
yarn install && yarn start
Allow custom constraints for getUserMedia()
.
Handle errors for when there are no camera or microphone devices.
Provide support for mobile browsers.
A small, fast and rich-API browser/platform/engine detector for both browser and node.
Made with ❤️ at Glimpse
FAQs
A wrapper for requesting audio and video permissions on JavaScript websites.
We found that mic-check demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.